Answered step by step
Verified Expert Solution
Question
1 Approved Answer
/****************************************************************************** Welcome to GDB Online. GDB online is an online compiler and debugger tool for C, C++, Python, Java, PHP, Ruby, Perl, C#, OCaml, VB,
/******************************************************************************
Welcome to GDB Online.
GDB online is an online compiler and debugger tool for C, C++, Python, Java, PHP, Ruby, Perl,
C#, OCaml, VB, Swift, Pascal, Fortran, Haskell, Objective-C, Assembly, HTML, CSS, JS, SQLite, Prolog.
Code, Compile, Run and Debug online from anywhere in world.
*******************************************************************************/
/*
* CECS 2203, Computer Programming I Lab
* Winter 2022, Sec. 07
* Date: December 19, 2022
* Topic: Lab 4 - Christmas Tree
* File name: lab04.cpp
* Name:
*/
/*
* This program prompts the user for the number of lines in a
* Christmas tree, and then prints the tree. Use the algorithm
* developed in the previous course meeting to implement a
* solution to the problem.
*/
// write the required include and using statements
#include
using namespace std;
// declare a method named printSpaces which has no return
// value and receives an integer as parameter
void printSpaces(int);
// declare a method named printDashes which has no return
// value and receives an integer as parameter
void printDashes(int);
// declare a method named spacesBefore which returns an
// integer and has two integers as parameters
int spacesBefore(int,int);
// declare a method named spacesMiddle which returns an
// integer and has one integer as parameter
int spacesMiddle(int);
// Declare a method named computeDashes which returns an
// integer and has an integer as parameter
int computeDashes(int);
// Declare a method named printTree which receives an
// integer as parameter and has no return value
void printTree(int,int);
// Declare a method named personalInfo which has no parameters or return value
void personalInfo();
int main(){
// declare an integer variable named rows and initialize to 0
int rows=0,row=0;
// prompt the user for the number of rows using the phrase
// "Enter the number of rows for the Christmas tree: "
cout
// store the value in the appropriate variable
cin >>rows;
cout
// call the printTree method
printTree(rows,row);
// call the personalInfo method
personalInfo();
//system("pause"); // For Visual Studio only!
return 0;
}
// The printSpaces method has no return value and receives an integer
// as parameter. This method prints the number of spaces indicated by
// the value of the parameter. No variables are declared in this method.
void printSpaces(int row){
while(row>0){
cout
row--;
}
}
// The printDashes method has no return value and receives an integer
// as parameter. This method prints the number of dashes indicated by
// the value of the parameter. No variables are declared in this method.
void printDashes(int){
}
// The spacesBefore function receives 2 integers as parameters and returns
// an integer value. This method implements the algorithm discussed in
// class to compute the number of spaces before the star or bar and returns
// the value. No variables are declared in this method.
int spacesBefore(int rows,int middle){
return rows-middle-1;
}
// The spacesMiddle function receives 1 integer as parameter and returns
// an integer value. This method implements the algorithm discussed
// in class to compute the number of spaces between stars and returns the
// value. No variables are declared in this method.
int spacesMiddle(int rows){
return 2*rows-1;
}
// The computeDashes function receives 1 integer as parameter and returns
// an integer value. This method implements the algorithm discussed in
// class to compute the number of dashes and returns the value.
// No variables are declared in this method.
int computeDashes(int rows){
return 2*rows - 1;
}
// The printTree method has no return value and receives an integer
// as parameter. This method implements the algorithm discussed in class
// to print the Christmas tree. No variables are declared in this method.
void printTree(int rows,int row){
while(row
printSpaces(spacesBefore(rows,row));
cout
if(spacesMiddle(row)
cout
}
else{
printSpaces(spacesMiddle(row));
cout
cout
Christmas Tree Problem -Need to know total number of rows, rows -prompt the user -develop a for - call method to compute spaces before - print the spaces - printstar - condition to continue - if true then compute middle spaces - print the spaces - print the star - insert new line CRLF - call the method to compute dashes - print the dashes - compute spaces before stand - print the spaces - print the stand nter the number of rows for the Christmas tree: 7 Program developed by, ID - Progxam finished with exit code 0 Press BNTYR to exit console }
row++;
}
}
// The personalInfo method prints a statement with your personal information using
// the phrase "***** Program developed by [YOUR NAME], ID# [YOUR ID NUMBER] *****"
// where the square brackets and the text within is substituted with your personal
// information. Make sure to add a blank line before and after the phrase is printed.
void personalInfo(){
cout
}
note: i don't know how to do the rest of the three
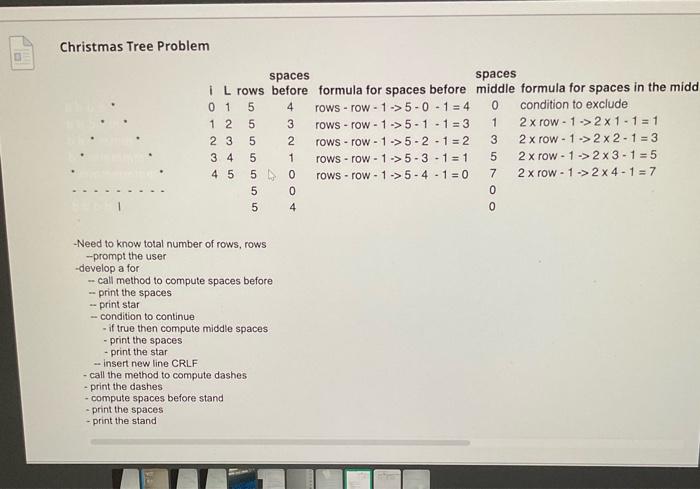
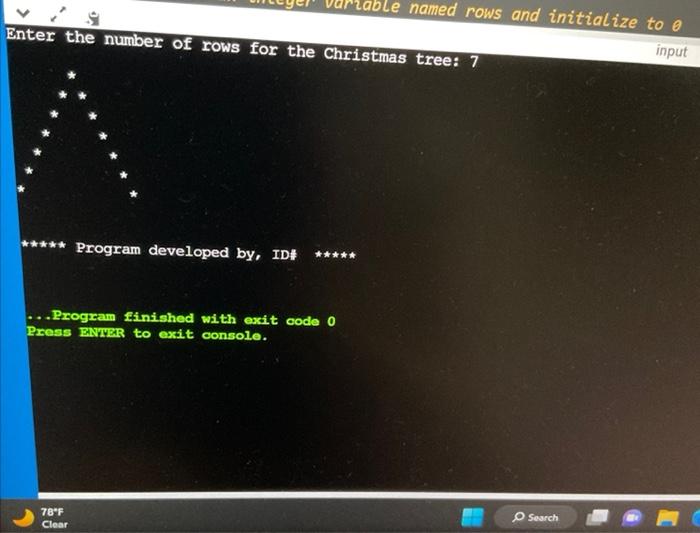
Step by Step Solution
There are 3 Steps involved in it
Step: 1
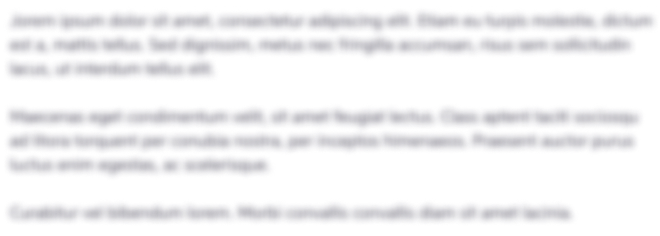
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started