Question
What is wrong with this Haskell code? Question: Define an evaluator for the language E. Its name and type should be eval :: E ->
What is wrong with this Haskell code?
Question:
Define an evaluator for the language E. Its name and type should be
eval :: E -> E
The result of eval should not contain any operations or comparisons, just a value constructed either with IntLit or BoolLit constructors. The result of the example program above should be BoolLit True. Note that E allows nonsensical programs, such as Plus (BoolLit True) (IntLit 1). For such programs, the evaluator can abort.
Code:
data E = IntLit Int | BoolLit Bool | Plus E E | Minus E E | Mult E E | Exponentiate E E | Equals E E deriving (Eq, Show)
program = Equals (Plus (IntLit 1) (IntLit 2)) (Minus (IntLit 5) (Minus (IntLit 3) (IntLit 1)))
eval :: E -> E eval (IntLit i) = (IntLit i) eval (BoolLit b) = (BoolLit b) eval (Plus e1 e2) = genHelper (+) (eval e1) (eval e2) eval (Minus e1 e2) = genHelper (-) (eval e1) (eval e2) eval (Mult e1 e2) = genHelper (*) (eval e1) (eval e2) eval (Exponentiate e1 e2) = genHelper (^) (eval e1) (eval e2) eval (Equals (BoolLit b1) (BoolLit b2)) = (BoolLit (b1 == b2)) eval (Equals (IntLit i1) (IntLit i2)) = (BoolLit (i1 == i2))
genHelper op (IntLit i) (IntLit j) = (IntLit (op i j))
mytree = Branch "A" (Branch "B" (Leaf 1) (Leaf 2)) (Leaf 3)
prog1 = Equals (Plus (IntLit 1) (IntLit 9)) (Mult (IntLit 5) (Plus (IntLit 1) (IntLit 1)))
prog2 = Equals (Equals (Mult (IntLit 4) (IntLit 2)) (Plus (IntLit 5) (Mult (IntLit 2) (IntLit 1)))) (Equals (BoolLit True) (BoolLit True))
Step by Step Solution
There are 3 Steps involved in it
Step: 1
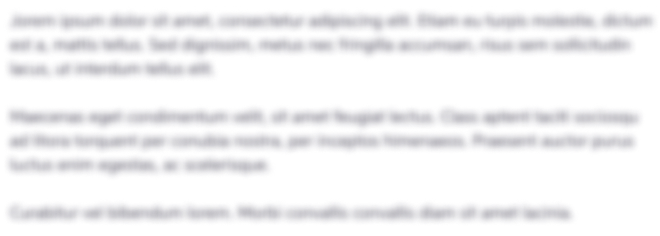
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started