Question
When I run the program it scratches after I convert infix to postfix (In visual studio code, it says: segmentation fault) It works fine, but
When I run the program it scratches after I convert infix to postfix (In visual studio code, it says: segmentation fault) It works fine, but I cannot debug it. please help me to debug the code.
#include
class Expression { public: Expression(string input, int direction); // constructor string inToPost(); // method to transform the local infix expression to postfix string postToIn(); // method to transform the local postfix expression to infix double evaluate(); // method to evaluate the expression
private: string infix; // private string variable to store the infix expression string postfix; // private string variable to store the postfix expression
};
Expression::Expression(string input, int direction) {
if (direction == 1) infix = input; else if(direction == 2) postfix = input; }
// inToPost() method
string Expression::inToPost() { stack
s.push('(');
else if (infix[i] == ')') { while (s.top() != '(') { postfix += s.top(); s.pop(); } s.pop(); } //If an operator is scanned else { while (!s.empty() && s.top() != '(' && (infix[i] == '*' || infix[i] == '/' || infix[i] == '+' || infix[i] == '-')) { postfix += s.top(); s.pop(); } s.push(infix[i]); } } //Pop all the remaining elements from the stack
while (!s.empty()) { postfix += s.top(); s.pop(); } return postfix; } // postToIn() method string Expression::postToIn() { stack
// elements from stack apply the operator
else { string val1 = s.top(); s.pop(); string val2 = s.top(); s.pop(); string temp = "(" + val2 + postfix[i] + val1 + ")"; s.push(temp); } } // There must be a single element in stack now which is the result return s.top(); } // evaluate() method takes the local postfix expression and returns the value of the expression.
double Expression::evaluate() { stack
}
int main() { string input; int direction; cout << "Enter expression in infix or postfix notation: "; cin >> input; cout << "Enter direction (1 for infix to postfix, 2 for postfix to infix): "; cin >> direction; Expression expression(input, direction); if (direction == 1) cout << "Postfix: " << expression.inToPost() << endl; else if (direction == 2) cout << "Infix: " << expression.postToIn() << endl; cout << "Evaluate expression: " << expression.evaluate() << endl; return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
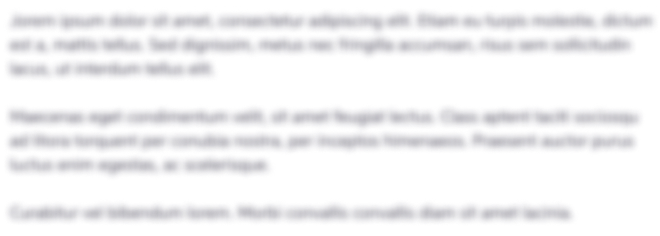
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started