Question
When the user clicks on the canvas, display the cheese and automatically rotate Mickey and move it towards the cheese. Find the angle from Mickey
- When the user clicks on the canvas, display the cheese and automatically rotate Mickey and move it towards the cheese.
- Find the angle from Mickey to the cheese with relation to the positive x, which is
- mikeyAngle = Math.atan2((cheeseY - mikeyY), (cheeseX - mikeyX));
- Translate Mickey along this angle for a small distance (mickeyDistance) with the following values:
- mikeyX += mikeyDistance * Math.cos(-mikeyAngle);
- mikeyY -= mikeyDistance * Math.sin(-mikeyAngle);
- Rotate Mickey by this angle
- Repeat the steps above
- When Mickey moves to within 15 pixels of the cheese, remove the cheese
Who Moved My Cheese?
- mickeyandcheesejs
-
let ctx; let mickeyX; let mickeyY; let cheeseX; let cheeseY;
//Setup function setup(){ ctx = document.getElementById("surface").getContext("2d"); document.getElementById("surface").addEventListener("click", clicked) addEventListener("keydown", keydown); mickeyX = 300; mickeyY = 300; draw(); }
//Clicked event function clicked(event){ cheeseX = event.offsetX; cheeseY = event.offsetY; draw() }
//Keydown event function keydown(event){ event.preventDefault(); if (event.key == "ArrowUp"){ mickeyY -= 10; } else if (event.key == "ArrowDown"){ mickeyY += 10; } else if (event.key == "ArrowLeft"){ mickeyX -= 10; } else if (event.key == "ArrowRight"){ mickeyX += 10; } if (detectHit(mickeyX, mickeyY, cheeseX, cheeseY)){ cheeseX = undefined; cheeseY = undefined; } draw(); }
// Draw the cheese and mickey function draw() { ctx.clearRect(0, 0, 600, 600); drawMickey(mickeyX, mickeyY); if(cheeseX != undefined) drawCheese(cheeseX, cheeseY); }
//Detect hit function detectHit(x1, y1, x2, y2){ return Math.sqrt(((x1 - x2) * (x1 - x2)) + ((y1 - y2) *(y1 - y2))) < 15 }
// Draw a piece of cheese at the given coordinates function drawCheese(x,y) { ctx.save(); // Move to the position (x, y) ctx.translate(x, y); ctx.beginPath(); ctx.fillStyle = "yellow"; // Draw the triangle ctx.lineTo(-30, 20); ctx.lineTo(20, 0); ctx.lineTo(-30, -20); ctx.lineTo(-30, 20); ctx.fill(); // Draw the four circles ctx.fillStyle="orange"; ctx.beginPath(); ctx.arc(2, 1, 4, 0, 2*Math.PI); ctx.fill(); ctx.beginPath(); ctx.arc(-18, 6, 4, 0, 2 * Math.PI); ctx.fill(); ctx.beginPath(); ctx.arc(-10, -5, 4, 0, 2 * Math.PI); ctx.fill(); ctx.beginPath(); ctx.arc(-24, -6, 4, 0, 2 * Math.PI); ctx.fill(); ctx.restore(); }
// Draw Mickey mouse at x, y at the given coordinates function drawMickey(x, y) { ctx.save(); // Move to the position (x , y) ctx.translate(x, y); // Draw a black circle for the tiny nose ctx.beginPath(); ctx.arc(0, 0, 2, 0, 2 * Math.PI); ctx.fill(); // Draw the triangle ctx.beginPath(); ctx.lineTo(0, 0); ctx.lineTo(-20, 10); ctx.lineTo(-20, -10); ctx.lineTo(0, 0); ctx.stroke(); // Draw the two white circles for the ears ctx.fillStyle = "white"; ctx.beginPath(); ctx.arc(-20, -10, 5, 0, 2 * Math.PI); ctx.fill(); ctx.stroke(); ctx.beginPath(); ctx.arc(-20, 10, 5, 0, 2 * Math.PI); ctx.fill(); ctx.stroke(); // Draw the two black circles for the eyes ctx.fillStyle = "black"; ctx.beginPath(); ctx.arc(-14, -4, 2, 0, 2 * Math.PI); ctx.fill(); ctx.beginPath(); ctx.arc(-14, 4, 2, 0, 2 * Math.PI); ctx.fill();
// Draw the whiskers ctx.beginPath(); ctx.lineTo(-4, -2); ctx.lineTo(-4, -10); ctx.lineTo(-4, -2); ctx.lineTo(0, -8); ctx.lineTo(-4, -2); ctx.stroke(); ctx.beginPath(); ctx.lineTo(-4, 2); ctx.lineTo(-4, 10); ctx.lineTo(-4, 2); ctx.lineTo(0, 8); ctx.lineTo(-4, 2); ctx.stroke(); ctx.restore(); }
-
Step by Step Solution
There are 3 Steps involved in it
Step: 1
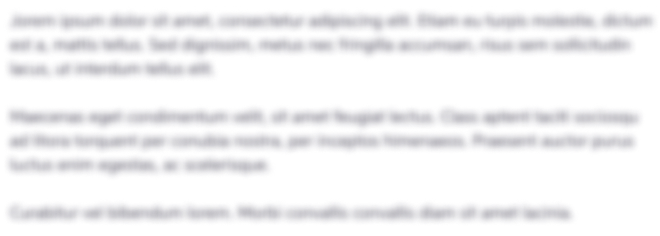
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started