Question
Why am I receiving error codes with my program? Can someone fix the problem without changing the MAIN function. Thanks!!!( This is C programming )
Why am I receiving error codes with my program? Can someone fix the problem without changing the MAIN function. Thanks!!!( This is C programming )
#include
#include
int Add(int a, int b)
{
return a + b;
}
int Mult(int a, int b)
{
return a*b;
}
int IAvg(int a, int b)
{
return (a + b) / 2;
}
float FAvg(int a, int b)
{
return (a + b) / 2;
}
int Big(int a, int b)
{
if (a >= b)
{
return a;
}
return b;
}
int Small(int a, int b)
{
if (a <= b)
{
return a;
}
return b;
}
int Max(int* a, int size)
{
int i = 0;
int max = 0;
for (i = 0; i < size; i++)
{
if (max < a[i])
{
max = a[i];
}
}
return max;
}
int Min(int* a, int size)
{
int i = 1;
int min = a[0];
for (i = 1; i < size; i++)
{
if (min > a[i])
{
min = a[i];
}
}
return min;
}
int Sum(int* a, int size)
{
int i = 0;
int sum = 0;
for (i = 0; i < size; i++)
{
sum = sum + a[i];
}
return sum;
}
char numVowels(char phrase[])
{
for(i=0;i { if(phrase[i]=='a' || phrase[i]=='A' || phrase[i]=='e' || phrase[i]=='E' || phrase[i]=='i' || phrase[i]=='I' || phrase[i]=='o' || phrase[i]=='O' || phrase[i]=='u' || phrase[i]=='U') vow=vow+1; } return vow; } void niceline(int i, char s, char m) { int j = i - 2; int k = 0; printf("%s", s); for (k = 0; k < j; k++) { printf("%s", m); } printf("%s", s); } void box(int i, int j, char s, char m) { int k = 0; for (k = 0; k < j; k++) { printf("%s", s); } for (k = 0; k < i - 2; k++) { niceline(7, s, m); } for (k = 0; k < j; k++) { printf("%s", s); } } int main() { int a, b, c; float f; a = 5; b = 4; printf("Using values %d and %d ", a, b); c = Add(a, b); printf("Add %d ", c); c = Mult(a, b); printf("Mult %d ", c); c = IAvg(a, b); printf("IAvg %d ", c); f = FAvg(a, b); printf("FAvg %.2f ", f); c = Big(a, b); printf("Big %d ", c); c = Small(a, b); printf("Small %d ", c); int nums[12] = { 22,43,35,8,43,17,54,41,29,48,36,19 }; int many = 12; for (a = 0; a < many; ++a) { printf("%2d ", nums[a]); printf(" "); } printf("max: %2d ", Max(nums, many)); printf("min: %2d ", Min(nums, many)); printf("sum: %2d ", Sum(nums, many)); char phrase[] = "DSU ... in Madison SD since 1881"; printf("String:: %s ", phrase); many = numVowels(phrase); printf("There are %d vowels ", many); niceline(6, 'a', 'b'); niceline(7, '2', '1'); box(4, 5, '@', ' '); box(6, 3, ' ', '#'); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
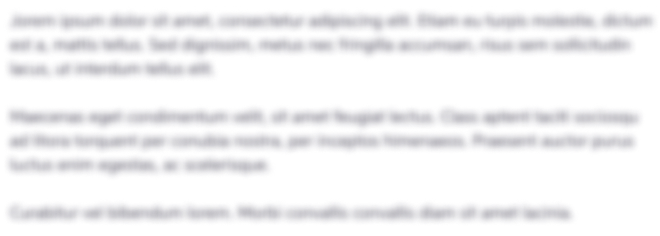
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started