Question
Why won't my code build and run? and what is the correct code so it builds successfully? getting errors in the Product.cpp file error:(Expected unqualified-id)
Why won't my code build and run? and what is the correct code so it builds successfully?
getting errors in the Product.cpp file error:(Expected unqualified-id)
code below
Main.cpp
#include
#include
#include "InventorySystem.hpp"
#include
#include "Product.hpp"
#include
#include
using namespace std;
int main() {
srand(time(NULL));
Inventory_System *h1 = nullptr;
h1 = new Inventory_System("Radioshack", 117);
h1->BuildInventory();
h1->ShowInventory();
h1->ShowDefectInventory();
h1->Terminate();
delete h1;
return 0;
};
InventorySystem.cpp
#include "InventorySystem.hpp"
#include "InventoryItem.hpp"
#include "Product.hpp"
#include
#include
#include
Inventory_System::Inventory_System() {
}
Inventory_System::Inventory_System(std::string name, int id) :
store_name(name),
store_id(id) {
}
Inventory_System::~Inventory_System() {
for (int i = 0; i < item_count; i++) {
delete ptr_inv_item[i];
}
}
int Inventory_System::GetStoreId(){
return store_id;
}
int Inventory_System::GetItemCount(){
return item_count;
}
std::string Inventory_System::GetStoreName(){
return store_name;
}
void Inventory_System::BuildInventory() {
std::ifstream file("products_test.txt", std::ios::in);
std::string name, quantity, price, condition;
Inventory_Item *item;
if (!file) {
std::cerr << "Error: Failed to open file ";
exit(-1);
}
else {
while (!file.eof()) {
item = new Product();
Product *product = static_cast
std::getline(file, name, ';');
product->SetItemName(name);
std::getline(file, quantity, ';');
product->SetItemQuantity(stoi(quantity));
std::getline(file, price, ';');
product->SetProductPrice(stold(price));
std::getline(file, condition, ' ');
switch (condition[0]) {
case 'D':
product->SetProductCondition(PC_DEFECTIVE);
break;
case 'N':
product->SetProductCondition(PC_NEW);
break;
case 'R':
product->SetProductCondition(PC_REFURBISHED);
break;
case 'U':
product->SetProductCondition(PC_USED);
break;
default:
product->SetProductCondition(PC_NEW);
break;
}
product->SetProductId(rand() % 10000);
ptr_inv_item[item_count] = product;
item_count++;
}
}
}
void Inventory_System::ShowInventory() {
for (int i = 0; i < item_count; i++) {
Product *temp = static_cast
temp->Display();
}
}
void Inventory_System::ShowDefectInventory() {
for (int i = 0; i < item_count; i++) {
Product *temp = static_cast
if (temp->GetProductCondition() == PC_DEFECTIVE) {
temp->Display();
}
}
}
void Inventory_System::Terminate() {
std::ofstream file;
std::stringstream ss;
file.open("example.txt");
for (int i = 0; i < item_count; i++) {
Product *temp = static_cast
file << temp->GetItemName() << ";"
<< temp->GetItemQuantity() << ";"
<< temp->GetProductPrice() << ";";
switch (temp->GetProductCondition()) {
case PC_DEFECTIVE:
file << "D ";
break;
case PC_NEW:
file << "N ";
break;
case PC_REFURBISHED:
file << "R ";
break;
case PC_USED:
file << "U ";
break;
default:
file << "D ";
break;
}
}
}
InventorySystem.h
#ifndef InventorySystem_hpp
#define InventorySystem_hpp
#include "InventoryItem.hpp"
#include "Product.hpp"
#include
#include
class Inventory_System {
public:
Inventory_System();
Inventory_System(std::string, int);
~Inventory_System();
int GetStoreId();
int GetItemCount();
std::string GetStoreName();
void BuildInventory();
void ShowInventory();
void ShowDefectInventory();
void Terminate();
private:
std::string store_name;
int store_id, item_count = 0;
Inventory_Item **ptr_inv_item = new Inventory_Item*[512];
};
#endif /* InventorySystem_hpp */
InventoryItem.cpp
#include "InventoryItem.hpp"
#include
Inventory_Item::Inventory_Item(){
}
Inventory_Item::Inventory_Item(std::string name, int quantity) :
item_name(name),
item_quantity(quantity) {
}
Inventory_Item::~Inventory_Item() {
std::cout << "Inventory Item " << item_name << " with " << item_quantity << " item(s) destroyed. ";
}
std::string Inventory_Item::GetItemName() const {
return item_name;
}
int Inventory_Item::GetItemQuantity() const {
return item_quantity;
}
void Inventory_Item::SetItemName(std::string name) {
item_name = name;
}
void Inventory_Item::SetItemQuantity(int quantity) {
item_quantity = quantity;
}
void Inventory_Item::Display() const {
std::cout << item_name << " (" << item_quantity << ") ";
}
InventoryItem.h
#ifndef InventoryItem_hpp
#define InventoryItem_hpp
#include
#include
class Inventory_Item {
public:
Inventory_Item();
Inventory_Item(std::string name, int quantity);
~Inventory_Item();
std::string GetItemName() const;
int GetItemQuantity() const;
void SetItemName(std::string);
void SetItemQuantity(int);
virtual void Display() const;
protected:
std::string item_name;
int item_quantity;
private:
};
#endif /* InventoryItem_hpp */
Product.cpp
#include "Product.hpp"
#include
#include
Product::Product() :
Inventory_Item(),
product_id(0),
product_price(0.0) {
}
Inventory_Item(),
product_id(0),
product_price(0.0){
}
Product::Product(std::string name, int quantity) :
Inventory_Item(name, quantity),
product_id(rand()% 1000),
product_price(100.0 * ((rand() + 1) / double(RAND_MAX + 2))){
}
Product::~Product() {
std::cout << "Product " << product_id << " priced $" << product_price << " has been destroyed.";
}
int Product::GetProductId() const {
return product_id;
}
double Product::GetProductPrice() const {
return product_price;
}
T_Condition Product::GetProductCondition() const {
return condition_;
}
void Product::SetProductId(int id){
product_id = id;
}
void Product::SetProductPrice(double price) {
product_price = price;
}
void Product::SetProductCondition(T_Condition condition) {
condition_ = condition;
}
void Product::Display() const{
Inventory_Item::Display();
std::cout << product_id << " $" << std::setprecision(4) << product_price << ' ';
}
Product.h
#ifndef Product_hpp
#define Product_hpp
#include "InventoryItem.hpp"
#include
#include "Product.hpp"
#include
typedef enum { PC_NEW, PC_USED, PC_REFURBISHED, PC_DEFECTIVE } T_Condition;
class Product : public Inventory_Item {
public:
Product();
Product(std::string, int);
~Product();
int GetProductId() const;
double GetProductPrice() const;
T_Condition GetProductCondition() const;
void SetProductId(int);
void SetProductPrice(double);
void SetProductCondition(T_Condition);
virtual void Display() const;
private:
T_Condition condition_;
int product_id;
double product_price;
};
#endif /* Product_hpp */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
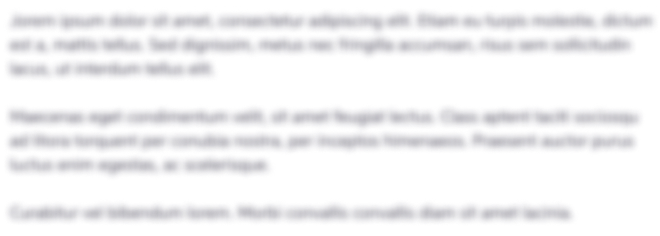
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started