Question
Write a c++ program: Create the Todo_list Class In a3.cpp, write a class called Todo_list that inherits from the Todo_list_base class in Todo_list_base.h: // ...
Write a c++ program:
Create the Todo_list Class
In a3.cpp, write a class called Todo_list that inherits from the Todo_list_base class in Todo_list_base.h:
// ... #include "Todo_list_base.h" class Todo_list : public Todo_list_base { private: // ... public: // ... };
In the private part of Todo_list, add variables for storing 0 or more Todo_items. If you use a vector to do this, make sure to add #include
In the public part of Todo_list, add a default constructor that creates a Todo_list with no items (size 0). Also, implement all the getters and setters listed in Todo_list_base.h.
Test what you've done by adding this function to a3.cpp:
void step_4_1_test() { Date easter(21, 4, 2018); Date halloween(31, 10, 2018); Todo_item weave_basket("Weave basket", easter); Todo_item carve_pumpkin("Carve pumpkin", halloween); Todo_list list; assert(list.size() == 0); list.add_item(weave_basket); assert(list.size() == 1); Todo_item item = list.get_item(0); Date date = item.get_due_date(); assert(item.get_description() == weave_basket.get_description()); assert(date.get_day() == weave_basket.get_due_date().get_day()); assert(date.get_month() == weave_basket.get_due_date().get_month()); assert(date.get_year() == weave_basket.get_due_date().get_year()); list.add_item(carve_pumpkin); assert(list.size() == 2); list.remove_item(0); assert(list.size() == 1); item = list.get_item(0); date = item.get_due_date(); assert(item.get_description() == carve_pumpkin.get_description()); assert(date.get_day() == carve_pumpkin.get_due_date().get_day()); assert(date.get_month() == carve_pumpkin.get_due_date().get_month()); assert(date.get_year() == carve_pumpkin.get_due_date().get_year()); list.remove_item(0); assert(list.size() == 0); cout << "All step_4_1 tests passed! "; }
Step 4.2: Add Reading from a File
Uncomment the read_from_file method in Todo_list_base.h, and then implement it in a3.cpp. The method reads the contents offilename, adding each item to the list. If the file does not exist, it should do nothing and no change is made to the list.
Each line of filename is formatted exactly the same as the output of Todo_item::to_string. todo_example.txt is an example of such a file. It contains 11 Todo_items, and is used in the tests below.
Add #include
Test what you've done by adding this function to a3.cpp:
void step_4_2_test() { Todo_list list; list.read_from_file("todo_example.txt"); assert(list.size() == 11); // 13/02/2023! optometrist in afternoon Todo_item eyes("optometrist in afternoon", Date(13, 2, 2023)); Todo_item item = list.get_item(0); Date date = item.get_due_date(); assert(item.get_description() == eyes.get_description()); assert(date.get_day() == eyes.get_due_date().get_day()); assert(date.get_month() == eyes.get_due_date().get_month()); assert(date.get_year() == eyes.get_due_date().get_year()); cout << "All step_4_2 tests passed! "; }
Step 4.3: Add Writing to a File
Uncomment the write_to_file(filename) method in Todo_list_base.h. When called, it should first sort the items by due date (earliest to latest), and then write each of those due dates to filename. If the file does not exist, it should create it. If the file already exists, then it will get over-written (be careful!).
The output of write_to_file should be formatted in exactly the same style as todo_example.txt. That way, you can read the file back in using read_from_file.
Similarly, uncomment write_to_html_file(filename) in Todo_list_base.h. When called, it first sorts the items by due date (earliest to latest), and then writes each of them to filename using their to_html_item. Also, the first line of the file is the HTML tag
- , and the last line is
Add #include
Test what you've done by adding this function to a3.cpp:
void step_4_3_test() { Todo_list list; list.add_item(Todo_item("Buy tinsel", Date(20, 12, 2018))); list.add_item(Todo_item("21/04/2018@ Weave basket")); list.add_item(Todo_item("Carve pumpkin", Date(31, 10, 2018))); list.write_to_file("step_4_3_output.txt"); list.write_to_html_file("step_4_3_output.html"); // read step_4_3_output.txt back in and compare to list Todo_list list2; list2.read_from_file("step_4_3_output.txt"); assert(list2.size() == 3); assert(list2.get_item(0).get_description() == "Weave basket"); assert(list2.get_item(1).get_description() == "Carve pumpkin"); assert(list2.get_item(2).get_description() == "Buy tinsel"); assert(list2.get_item(0).get_due_date().get_day() == 21); assert(list2.get_item(0).get_due_date().get_month() == 4); assert(list2.get_item(0).get_due_date().get_year() == 2018); assert(list2.get_item(1).get_due_date().get_day() == 31); assert(list2.get_item(1).get_due_date().get_month() == 10); assert(list2.get_item(1).get_due_date().get_year() == 2018); assert(list2.get_item(2).get_due_date().get_day() == 20); assert(list2.get_item(2).get_due_date().get_month() == 12); assert(list2.get_item(2).get_due_date().get_year() == 2018); assert(list2.get_item(0).is_done() == true); assert(list2.get_item(1).is_done() == false); assert(list2.get_item(2).is_done() == false); cout << "All step_4_3 tests run: check the HTML results by hand! "; }
Here are the output files created by the test: step_4_3.txt, step_4_3.html. Make sure to manually check that step_4_3.html looks correct.
Part 5: The Final Program
As a final test of your a3.cpp, write and test a void function called part5() that reads the file part5_todos.txt and then prints this:
done: 476 not done: 524 oldest: 05/01/2018@ freeze-dry magnet-shaped green orange newest: 28/12/2023@ defrost large yellow cherry
Print the output exactly in this format.
In addition, make a file called final_output.txt created by a call to write_to_file. And make a file called final_output.html created by a call to write_to_html_file.
When your part5() function is tested, the contents of part5_todos.txt will behave the same format as todo_example.txt, but the content of the todos, and number of todos, may be different.
The todo_list_base.h has been given below:
// Todo_list_base.h
//////////////////////////////////////////////////////////////////////////// // // Todo_list_base class // ////////////////////////////////////////////////////////////////////////////
class Todo_list_base { public: // destructor virtual ~Todo_list_base() {}
// getters virtual int size() const = 0; virtual Todo_item get_item(int i) const = 0;
// setters virtual void add_item(const Todo_item& item) = 0; virtual void remove_item(int i) = 0;
// virtual void read_from_file(const string& filename) = 0;
// printing virtual void write_to_file(const string& filename) = 0; virtual void write_to_html_file(const string& filename) = 0;
}; // class Todo_list_base
Step by Step Solution
There are 3 Steps involved in it
Step: 1
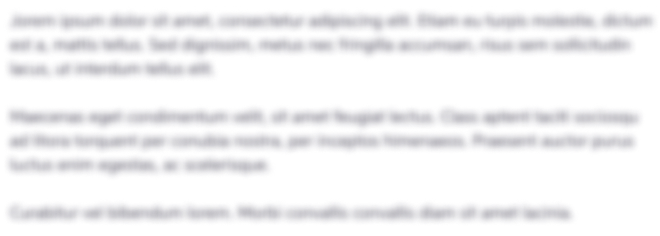
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started