Question
Write a C++ program: Q1. Class Reference (20 marks) Implement a class, called Reference, to represent the characteristics that are common to all the References.
Write a C++ program:
Q1. Class Reference (20 marks)
Implement a class, called Reference, to represent the characteristics that are common to all the References. Namely,
- All references must have a unique identifier (of type int).
- All references have a title and author (both of type string or char*), as well as year of publication (of type int);
Q2. Class Article (20 marks)
Article is a specialized Reference that also has information about the journal where the article is published, start page, and end page. More precisely,
- An Article is a derived class of Reference
- It also stores information about the journal (type string or char*), and the startPage and endPage (both of type int);
- It implements the additional member function int getNumberOfPages(), which returns the total number of pages (computed using starPage and endPage);
Q3. Class Book (20 marks)
Book is also a specialized Reference that has information about the publisher of this reference. It also has number of pages as one of its attributes.
- Book is a derived class of Reference
- It stores the publisher (type string or char*) of this reference and the number of pages of this book (type int);
- It also implements the member functions getNumberOfPages(), which returns the number of pages of this reference. In the case of Book reference, the number of pages is a single number, therefore, the member function simply returns this number;
- It also implements functions related to the publisher.
Q4. Class TextBook (20 marks)
TextBook is a specialized Book. A textbook has an additional attribute, Web URL (Uniform Resource Locator), where students can find additional material such as slides, exercises, etc.
- TextBook is a derived class of Book
- It implements additional functions related to the setting and getting the URL
Q5. ReferenceManager (20 marks)
Create a class called ReferenceManager that will be used as a container to hold objects of classes Article, Book, and TextBook.
- ReferenceManager has a fixed capacity. It uses a fixed size array to store References.
- The first reference added to the container will be added at position zero, the second one at position 1, and so on. A reference is always added at the next available position;
- A ReferenceManager has one regular constructor: ReferenceManager(int capacity). The constructor must create an array of size capacity, which will be used to store References. It will also initialize the instance variables that your implementation requires.
- ReferenceManager will have size data member, which will track the number of references in the array.
- bool add(Reference & reference): adds a reference at the next available position and returns true, or returns false if the reference manager is full.
- int get(int pos): returns the identifier of the Reference object stored at position pos of the ReferenceManager. You can assume that pos is a valid index, i.e. 0 <= pos < size;
- bool delete(int pos): removes the element at position pos of this ReferenceManager and returns true, or returns false if pos was not a valid index. It must also shift the elements of the array towards the beginning of the array;
- bool search(int id); return true if the reference with identifier id exists, false otherwise
Step by Step Solution
There are 3 Steps involved in it
Step: 1
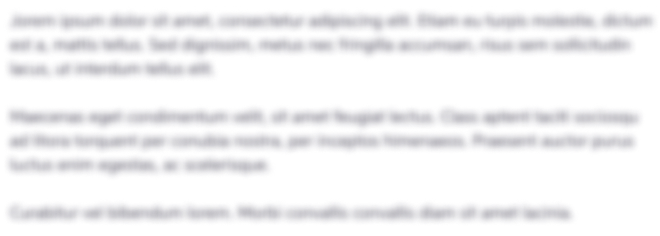
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started