Question
Write a C++ program that implements a memory game where the user is asked to remember colors that are displayed randomly for a short period
Write a C++ program that implements a memory game where the user is asked to remember colors that are displayed randomly for a short period of time. The user is first prompted for the number of random colors to display. An example prompt is given below:
Valid entries are between 2 and 5 inclusively. There are 5 possible colors that can be displayed by this program. The following 5 colors are to be utilized by this program.
Color RGB Color #
Red (255,0,0) 0
Green (0,255,0) 1
Blue (0,0,255) 2
Yellow (255,255,0) 3
Purple (255,0,255) 4
The program will then display the desired number of colors as selected from the table given above. Your program should perform the following steps:
1. Generate N random numbers between 0 and 4 inclusively. The random numbers do not have to be unique duplicates are allowed. N is the number of colors to display as entered by the user on this prompt. For this example, N is set to 5.
2. The random numbers will correspond to a color. For instance, if the first random number is 2, it corresponds to Blue. If the second random number is 4, it corresponds to Purple, and so on.
3. All random numbers should be stored in an array of integers with the MAX_ELE set to 5.
4. The program then prompts the user for a delay. This is the amount of time the random colors will actually be displayed on the screen. Valid times are between 1 and 3 seconds inclusive. An example of this prompt is given below:
This example will display the random colors for a total of 1 second.
5. The program will next display a 75x75 rectangle corresponding to each random color generated in step 3 as shown below (Suggested coordinates are shown):
For this example, the following screen is displayed for a total of 1 second:
The distance between each rectangle is 25. The text under each rectangle should be aligned with the left edge of each rectangle at approximately 20 pixels beneath the rectangle. Note that the 5 colors are unique and are displayed in a random order - for this example blue, red, green, green, and red. For this example, the colors are displayed for 1 second - (i.e., the user has 1 second to remember the order of these colors).
6. The program will next remove the randomly displayed colors (i.e., clearGraphics()), and then display the following menu prompting the user for the 1st (left-most) color:
The score will be incremented by 2 for each correct answer and decremented by 2 for each incorrect answer.
If the user believed that the 1st color displayed was Red, he should enter 1 for the choice, 2 for green and so on. In this case the user will enter 3 for blue and the following will be displayed in the Graphics Windows:
Note that the left-most rectangle is now displayed the word CORRET! is displayed, and the score is incremented by 2 for this correct answer
The user will then be asked to prompt for the 2nd color as shown below:
In this case, the user believes the color is Red and enters 1 as shown above. Upon entering 1, the following is displayed in the Graphics Window:
Red is the correct answer and the score is once again incremented by 2. Note that the 2nd rectangle is now drawn.
The user is next prompted for the 3rd color as shown below:
A score is accumulated for each selection. If the user chooses the incorrect color, 2 points are deducted from his score. If he chooses the correct color, 2 points are added to his score. The next example illustrates the result when the incorrect color is chosen.
The user is now asked to select the 3rd color:
In this case, the user believes that the 3rd color is once again Red and enters 1. Upon selecting Red, the following is displayed in the Graphics Window:
Red is incorrect, and 2 points are deducted from the score. Note that the 3rd rectangle is not drawn. The user will be continually prompted until he enters the correct color and the score is decremented by 2 for each incorrect answer. Again the user is prompted for the 3rd color as shown below:
In this case the user believe the 3rd color is Green which is correct. Upon entering 2, the following is displayed in the Graphics Window:
Note that the correct answer increments the score by 2 and the 3rd rectangle is now drawn.
The user will then be prompted to choose the 4th color as shown below:
This is the correct choice and the following will be displayed in the Graphics Window:
The user is then prompted for the last color as shown below:
For this example, the user believes that the 5th color is Red, which is correct. Upon entering 1, the following is displayed in the Graphics Window:
Note that the score and number of turns are both displayed.
The user is then prompted to play the game again.
Allow the user to repeat the program as many times as desired.
:
Required Functions
The following functions are required when implementing this program. You are free to add any extra functions that you like.
void getNoColors(int* no_colors);
This function prompts the user for the number of colors to select from the 5 possible colors. Valid values are between 2 and 5 inclusively.
Input: no_colors - pointer to integer, similar to pass by reference, but uses pointer instead. Variable no_colors is changed when function completes.
void setColors(int* colors, int no_colors);
This function randomly selects no_colors colors from the 5 possible colors and stores these random values into an array of integers - colors.
Input: no_colors - The number of desired colors to process (between 2 and 5 inclusively).
Input: colors - Array of integers used in storing the randomly generated values. These values are between 0 and 4 inclusively. These numbers correspond to the color number given above in table.
void getDelay(int* no_secs);
Prompts the user for the number of seconds to display the the randomly generated colors. The user will will enter a number between 1 and 3 (pertanining to seconds) inclusively.
Input: no_secs - pointer to integer, similar to pass by reference, but uses pointer instead. Variable no_secs is changed when user is prompted and enters a value for the delay - this is the number of seconds to display the random colors.
void displayColors(int* colors, int no_colors, int delay);
This function draws no_color rectangles and colors each corresponding rectangle based on the randomly generated numbers stored in colors. The rectangles are displayed on the screen for delay seconds, after which these rectangles are removed.
Input: colors: Array of random numbers between 0 and 4 inclusively. These random numbers correspond to the color # shown above. For instance, a number of 0 corresponds to red, 1 corresponds to green, etc.
Input no_colors: The number of desired colors to display (between 2 and 5 inclusively).
Input delay: The number of seconds to display the randomly generated colors. The colors are removed immediately after this delay has completed.
void game(int* colors, int no_colors, int delay);
This is the most important function for this program. This function performs the following steps as covered in the overview starting with step 5 on pages 2 thru 8.
1. displays the menu for selecting a color.
2. If the user selects the correct color, the colored rectangle is redrawn at its previously displayed location and an informational message is displayed iin the graphics window indicating that he selected the correct rectangle. 2 points are added to his score and his total score is displayed in the graphics window as well.
3. If the user selects the incorrect color, an information message is displayed in the graphics window indicating that his selection is incorrect. 2 points are also deducted from his score. His total score is also displayed in the graphics window.
4. The game continues until the user has correctly selected all the colors in the correct order as they were originally displayed by the displayColors function.
5. An information message is displayed once the game is over. The total score and the number of turns required to play the game is displayed as well (all displays are in the graphics window).
Guidelines for main:
-
Invoke getNoColors function and prompt the user for the number of colors to display.
-
Invoke the getDelay function and prompt the user for the number of seconds to display the colors to the user.
-
Invoke the setColors function and randomly set the colors to be displayed.
-
Invoke the displayColors function that displays the colors to the user for the desired number of seconds.
Invoke the game function and allow the user to select each color in order.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
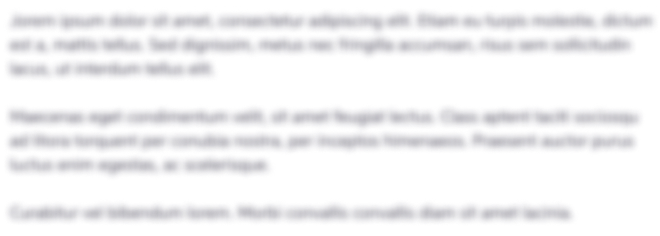
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started