Question
Write a C++ program that simulates an IoT (Internet of Things) controller. Any number of IoT devices can connect to this controller and report their
Write a C++
program that simulates an IoT (Internet
of Things) controller. Any number of IoT devices can connect to this controller and
report their status. The controller will thus have a complete picture of all the IoT
devices that have reported to it, the current status of those devices and when last the
status changed/updated.
To simulate the messages sent by the various IoT devices, a text le called device data.txt
is provided that contains a number of device messages sent, with each line representing
a distinct message. Each message contains a device name, a status value and a Unix
epoch value which are separated by commas. Your C++ program will read this file
line by line and sent the contained message to a new child process. The child process will
separate the message into device name, status value and epoch value, convert the epoch
to a date and time string and send the data back to the parent process. The parent
process will use this data to create and maintain a IoT device list with associated status
value and time.
Implementation:
The following steps show how your program should work:
1. Your program opens the provided IoT device data input le.
2. It then reads the input le line by line.
3. For each input line, it creates a new child process with the fork() function.
4. The parent process then sends the input line to the child process via a pipe.
5. The child process transforms the input line to data values and sends them back
to the parent via a pipe.
6. The parent process uses the received data to update an IoT devices status list.
7. When the reading of the input le is complete, the program prints the nal IoT
devices status list.
IMPORTANT:
You have to communicate by using pipes and the read() and write() functions.
You have to use the fork() function to create child processes.
You are not allowed to execute other commands from within your program by
using functions like execve() and friends.
You have to print verbose output to clearly show what task each process performs.
Your program should either use the le device data.txt or ask for a device data
file name.
Contents of:
device data.txt
FrontDoor,Closed,1532700000 LivingLight,Off,1532700000 LivingTemp,19,1532700000 LivingTemp,18.5,1532703600 FrontDoor,Open,1532707200 LivingTemp,18,1532707200 LivingLight,On,1532707203 FrontDoor,Closed,1532707205 LivingTemp,18.1,1532710800
EXAMPLE OUTPUT:
Parent sent file line 8
child received message line: FrontDoor,Closed,152707205
Child sent device: FrontDoor
Child sent value: Closed
Child sent time: 18:00:05 27/07/2018
Parent received update for FrontDoor: Closed at 18:00:05 27/07/2018
Parent sent file line 9
Child received message line: LivingTemp,18.1,1532710800
Child sent device: LivingTemp
Child send value: 18.1
Child sent time: 19:00:00 27/07/2018
Parent received update for LivingTemp: 18.1 at 19:00:00 27/07/2018
=========
Device List:
=========
FrontDoor Closed @ 18:00:05 27/07/2018
LivingLight: On @ 18:00:03 27/07/2018
LivingTemp: 18.1 @ 19:00:00 27/07/2018
Step by Step Solution
There are 3 Steps involved in it
Step: 1
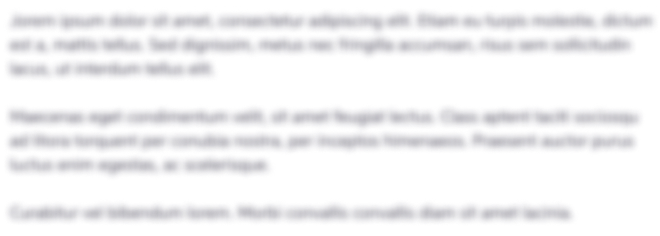
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started