Question
Write a C program to convert user-entered decimal integer to binary representation. Algorithm requirements: For negative input, the program should implement all of the following
Write a C program to convert user-entered decimal integer to binary representation.
- Algorithm requirements:
- For negative input, the program should implement all of the following steps to get the 2s complement binary:
- Convert magnitude to 16-bit binary
- Flip all bits
- Add 1
- For decimal-to-binary conversion the division-remainder algorithm should be implemented
- For negative input, the program should implement all of the following steps to get the 2s complement binary:
- Data validation requirements
- The binary to be displayed should be of numOfBits (i.e., in the starting code its 8)
- For negative integer input, the binary to be displayed should be of the 2s complement form.
- The valid range of the input integer should be checked so as to represent as a numOfBits binary. If user enters any number outside the range, prompt an error message instead of trying to display binary.
- Other requirements
- Name the program DecimalToBinaryConverter.c
- Include program header comments like author, description, etc.
- Include reasonable amount of in-line comments to explain major steps
- The program should allow user to continue entering integer numbers. Each time an integer is entered, it should display the binary of the integer if in range.
- The program should be terminated when random non-integer input is entered.
#include #include #include #include void magnitudeToBinary(int n, int arr[], int numOfBits); void flipBits(int arr[], int numOfBits); void addOne(int arr[], int numOfBits); void printArray(int arr[], int numberOfElements); int main(void) { //Declare an array of size 8 to simulate 8-bit binary int numOfBits = 8; // change the value to test different number of bits binary int arr[numOfBits]; int upperBound = pow(2, numOfBits - 1) - 1; int lowerBound = - pow(2, numOfBits - 1); //Declare integer n to hold the decimal integer int n = 0; puts("Enter integers and convert it to binary."); puts("Non-numeric input terminates program."); //Continually taking input and converting it to binary while (scanf("%d", &n) == 1) {//if successfully read in ONE decimal integer //Check if n is within the range of a 8-bit binary if((n < lowerBound) || n > upperBound) { printf("%d is out of range. ", n); printf("Enter a number within [%d, %d] to be represented in %d-bit binary.", lowerBound, upperBound, numOfBits); continue; //out of range, continue to next round } //Initialize the array to all 0s for(int i = 0; i < numOfBits; i ++){ arr[i] = 0; } //Convert magnitude (absolute value) to binary magnitudeToBinary(abs(n), arr, numOfBits); //if the number is negative: perform the 2's complement procedure //--> flip all bits, then add 1. if(n < 0){ //Flip all bits in char arr flipBits(arr, numOfBits); //Add 1 addOne(arr, numOfBits); } //Output binary: printf("Integer: %d, binary: ", n); printArray(arr, numOfBits); } return EXIT_SUCCESS; } /* printArray: print numberOfElements in the argument integer array */ void printArray(int arr[], int numberOfElements){ int i = 0; for(; i < numberOfElements; i ++){ printf("%d", arr[i]); } puts("");//new line } /* addOne: arithmatically add 1 to the binary simulated by character array * INPUT: int arr[]: the integer array holding the binary representation * int numOfBits: the number of bits in the binary rep. * */ void addOne(int arr[], int numOfBits){ /* Truth table * ****************************** * carry arr[i] | arr[i] carry * 1 0 | 1 0 * 1 1 | 0 1 * 0 0 | 0 0 * 0 1 | 1 0 * *********************************/ int carry = 1; for(int i = numOfBits - 1; i >= 0; i --){ if(carry != arr[i]){ arr[i] = 1; carry = 0; } else if(carry == 1){ arr[i] = 0; } else{ arr[i] = 0; } } return; } /* flipBits: perform 1's complement on the binary, i.e., change 1 to 0, 0 to 1 * INPUT: int arr[]: the int array holding the binary rep. * int numOfBits: the number of bits in the binary rep.* * */ void flipBits(int arr[], int numOfBits){ //**********Implement your solution here*********** return; } /* magnitudeToBinary: Convert a non-negative decimal integer to binary using division-remaider algorithm Store the representation in a int array) * * INPUT: int n: The decimal integer number to be converted * int arr[]: the int array holding the binary rep. * int numOfBits: the number of bits in the binary * * */ void magnitudeToBinary(int n, int arr[], int numOfBits){ //Implement the division-remaider algorithm here return; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
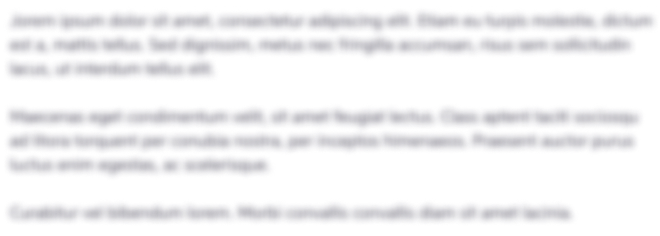
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started