Question
Write a C program to process and report mark records. The mark records are given by comma-separated values (CSV) format: marks.txt Myrie,76.7 Hatch,66.5 Costa,45.1 Dabu,74.4
Write a C program to process and report mark records. The mark records are given by comma-separated values (CSV) format:
marks.txt
Myrie,76.7
Hatch,66.5
Costa,45.1
Dabu,74.4
Sun,67.7
Chabot,80.4
Giblett,59.1
Suglio,85.7
Smith,60.1
Bodnar,93.6
Allison,67.7
Koreck,77.4
Parr,92.5
Lamont,98.1
Peters,82.3
Wang,98.1
Eccles,77.8
Pereira,80.3
He,85.7
Ali,88.0
Compute average, standard deviation and letter grades, output results to:
report.txt
Letter grade
Myrie B
Hatch C
Costa F
Dabu B
Sun C
Chabot B
Giblett D
Suglio A
Smith C
Bodnar A
Allison C
Koreck B
Parr A
Lamont A
Peters B
Wang A
Eccles B
Pereira B
He A
Ali A
Stats summary
count: 20
average: 77.9
standard deviation: 13.5
median: 77.6
myrecord.h
// add descriptions to function declarations
#include
#include
#include
#include
#define MAX_REC 100
#define MAX_LINE 100
typedef struct {
// your design and implementation
} RECORD;
char letter_grade(float s);
int import_data(RECORD dataset[], char *filename);
int report_data(RECORD dataset[], int n, char *filename);
void swap(float *first, float *second);
void quick_sort(float *a, int left, int right);
myrecord.c
#include "myrecord.h"
char letter_grade(float s){
// your code
}
int import_data(RECORD dataset[], char *filename) {
// your implementation
char delimiters[] = ", ";
char line[100];
int i = 0; // record counter
char *token = NULL;
while(fgets(line, sizeof(line), fp) != NULL){
token = (char *) strtok(line, delimiters);
strcopy(dataset[i].name, token); // copy string char array
token = (char *) strtok(NULL, delimiters);
dataset[i].score = atof(token); // convert string to float
i++;
}
}
int report_data(RECORD dataset[], int n, char *filename) {
// your implementation
// fprintf(fp, "%-15s%c ", dataset[i].name, letter_grade(dataset[i].score));
// compute mean, standard deviation,
// fprintf(fp, " Stats summary ");
// fprintf(fp, "%-20s%d ", "count:", n);
// fprintf(fp, "%-20s%3.1f ", "average:", mean);
// fprintf(fp, "%-20s%3.1f ", "standard deviation:", stddv);
// compute median
// create float array a[n], sort array a[n] using quick sort
// then calculate the median
// fprintf(fp, "%-20s%3.1f", "median:", median);
}
void swap(float *first, float *second)
{
float temp = *first;
*first = *second;
*second = temp;
}
void quick_sort(float *a, int left, int right)
{
// modify the quick_sort for sorting float array
if(left < right){
int i = partition(a, left, right);
quick_sort(a, left, i - 1);
quick_sort(a, i + 1, right);
}
}
Specifically, write C programs myrecord.h and myrecord.c containing the following:
- Definition of a structure named RECORD to hold a persons name of 20 characters and the score of float type.
- char letter_grade(float score); converts a float score to a letter grade according to ranges A=[85, 100], B=[70, 85), C=[60, 70), D=[50,60), F=[0,50), and returns the letter grade.
- int import_data(record dataset[], char *filename); imports data from file of name passed by filename, and stores all record entries in an array of RECORD dataset[], and returns the number of records.
- void report_data(RECORD dataset[], int count, char *filename); computes the letter grades of dataset[], average score, standard deviation, and median, and outputs the results to file of name passed by filename.
main.c to test.
main.c
#include "myrecord.h"
int main(int argc, char *args[]) {
char infilename[40] = "marks.txt"; //default input file name
char outfilename[40] = "report.txt"; //default output file name
if (argc > 1) {
if (argc >= 2) strcpy(infilename, args[1]);
if (argc >= 3) strcpy(outfilename, args[2]);
}
RECORD dataset[MAX_REC];
int record_count = 0;
record_count = import_data(dataset, infilename);
if (record_count >=1) {
report_data(dataset, record_count, outfilename);
printf("output to file ");
}
else
printf("no data, no output to file ");
FILE *fp =fopen(outfilename, "r");
if ( fp == NULL ) {
printf("output file does not exist ");
return 0;
}
char buf[100];
while( !feof(fp) ) {
fgets(buf, sizeof(buf), fp);
printf("%s", buf);
}
fclose(fp);
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
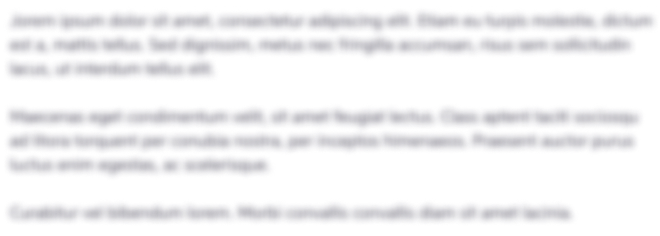
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started