Question
Write a C program to simulate a falling object . The program should ask for the initial height of the object, in feet. The output
Write a C program to simulate a falling object. The program should ask for the initial height of the object, in feet. The output of the program should be the time for the object to fall to the ground, and the impact velocity, in ft/s and miles/hour. Your program should use Eulers method to numerically solve the differential equations describing the motion of a falling object. In Eulers method, the state of the object at some small future time is calculated using the values at the present time. This small future timestep is typically called delta time, or dt. Thus the position (p) and speed (v) of the object in the next timestep t + dt is written as a simple function of the position and speed at the current timestep t (g is the acceleration due to gravity): v(t+dt) = v(t) + g * dt
p(t+dt) = p(t) + v(t+dt) * dt You should actually start out with the velocity as zero, and the position at the initial height of the object. Then your position (above the ground) would be: p(t+dt) = p(t) - v(t+dt) * dt And you would integrate until the position becomes less than or equal to zero. The input/output formats for your program should look like this: Program to calculate fall time and impact speed of a falling object dropped from a specific height. Enter initial height in feet: 100 Falling time = 2.492224 seconds Impact speed = 80.249613 feet/sec Impact speed = 54.715645 mph Part of this assignment is to determine an optimum value for dt. Clearly, the value of the time step that you use in the simulation will be important. If the timestep (dt) is too large, then the results will not be accurate. However, if the time step is too small, then the program run time will be excessive. For this assignment, you must determine the value of delta time that is as large as possible but at the same time, results in an answer that is accurate to 5 significant figures i.e., further decreases in the time step no longer change the result past the 5th digit to the right of the decimal place. Turn in your program configured with this value of dt. Accuracy and precision is important for this assignment. You should use a double float data type for all real numbers. Also assume the effect of gravity does not vary with distance from the earth, the rate of acceleration is 32.2 feet per second per second, and the resistance of passing through the atmosphere is non-existent. Using symbolic constants for gravity G = 32.2 and delta time DT = .000??????? makes sense.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
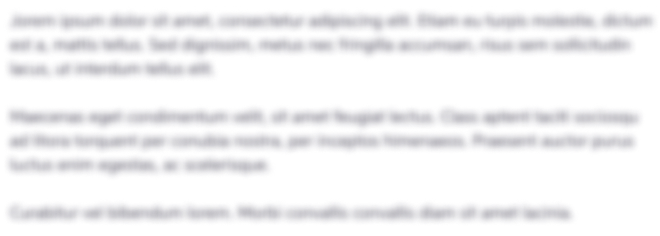
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started