Question
Write a code in java that asks the user to input fractions. We will be working with three java files. For Example, if the user
Write a code in java that asks the user to input fractions. We will be working with three java files.
For Example,
if the user inputs "5 2/4" the program will print "5 1/2"
If the user inputs " 4/ 5" the program will print out "4/5"
If the user inputs "4/2" the program prints out 2.
If the user "accidently" prints L replace it with the number one OR if the user prints O replace it with Zero
MY CODE
Fraction.java-----------------------------------------------------------------------
class Fraction{ /ew type //box: declarations (Attributes) int num; /umerator int den; //denominator
//box: constructors: initialize the new type Fraction(int m, int n){//one //GCD from the Utility int g = Utility.findGCD(m,n); num = m/g; den = n/g; /um = m; //den = n; } Fraction(int m){//two num = m; den = 1; } Fraction(){/o input//tree num = 0; den = 1; } Fraction(String s){//four /*this is where we deal with fractions like "2 5/10" and L/5, 4O/10.. */ } //method static Fraction add(Fraction f, Fraction g){ Fraction h; h = new Fraction(f.num*g.den + g.num*f.den,f.den*g.den); return h; } public String toString(){ //don't change this String s; if (den !=1) s= num+"/"+den; else s=""+num; return s; }
}//class
Test.java----------------------------------------------------------------------
import java.util.Scanner;
class Test{ public static void main(String[] args) {
//scanner to ask for a fraction from the user Scanner myObj = new Scanner(System.in); System.out.print("Enter a Fraction>"); String sFract = myObj.nextLine(); System.out.println("Fraction= |" + sFract+"|"); //extract num and den to call constructor #1 //trim means to remove whitespace from both ends of a string sFract = sFract.trim(); //System.out.println("TrimFraction= |" + sFract+"|");// if a user inputs " 1 / 2" it will print "1/2" int pos = sFract.indexOf('/');//NOTE //System.out.println("Position= |" + pos+"|"); String sNum = sFract.substring(0, pos); sNum = sNum.trim(); //System.out.println("sNum= |" + sNum+"|"); int inum = Integer.parseInt(sNum); //System.out.println("iNum= |" + inum+"|");
String sDem = sFract.substring(pos+1); sDem = sDem.trim(); int iden = Integer.parseInt(sDem); //System.out.println("sDem= |" + iden+"|"); Fraction f = new Fraction(inum,iden); System.out.println("f= "+ f); //Fraction h = new Fraction(Utility.parseFraction()); Fraction h = new Fraction();inputs 3 2/8 System.out.println("h= "+ h);//output 3 1/4 } }
Utility.java------------------------------------------------------------------------------
class Utility{ //method that is called findGCD(int m, int n) static int findGCD(int i, int j){ // find gcd: larger is reduced by the smaller // repeat until they are the same
while (i != j){ if (i > j) i = i - j; else j = j - i; }// while
return i; } }
Project 1 suggestions; In order to do Project1, students need to review the lectures and do the labs in the first 2 weeks 1. At the beginning, we practice how to compile and execute a simple program with only main() method. 2. Next we start with a gcd algorithm and code it to be a while loop in the main() method. 3. The gcd codes with 3 parts input , process, and output are moved out to Utility while the main is shortened by a calling instruction Utility.findGCD(int m, int n) with a output return. 4. The new type (object type) of Fraction is created with 3 boxes: Attributes, Constructor(s), and methods especially the toString() to print the attributes of an object. 5. The analysis of many possible constructors are introduced to allowusers to create the fractions with different types of input. Moreover the toString() can be modified to display the special fractions in different forms. 6. Project 1 is a continuation of Step 5 a. The input from keyboard should be moved to Utility b. The new constructor Fraction(String s) should be created by calling Step 6a, and parsing code of strings from main should be moved to this constructor. c. Finally, the toString() is modified in parallel to Step 6b. Note: You must master by redoing all steps 1-5 LectureFeb3-Morning Feb 3 : Lecture & Project 1 Review Analysis of input (from Users) - direct:(int) 2 params, 1 param *no param? *keyboard *(Scanner):String methods *" 2/6 " *"2 /6" *"2 / 6" "4" "20/5" "1 1/2" " 1 1/2" "1/2" " 10/15" // char o Output (display) - simple forms "2/1" 2 "2/4" 1/2 "5 2/6" 5 1/3 4/3 1 1/3 => 11 11 => Project 1: Do all cases in this page. Submit your zip file of the sources (Test.java, Fraction.java, and Utility.java) to spham@csun.edu BEFORE NEXT Thursday (midnight of Wed go to th) Project 1 suggestions; In order to do Project1, students need to review the lectures and do the labs in the first 2 weeks 1. At the beginning, we practice how to compile and execute a simple program with only main() method. 2. Next we start with a gcd algorithm and code it to be a while loop in the main() method. 3. The gcd codes with 3 parts input , process, and output are moved out to Utility while the main is shortened by a calling instruction Utility.findGCD(int m, int n) with a output return. 4. The new type (object type) of Fraction is created with 3 boxes: Attributes, Constructor(s), and methods especially the toString() to print the attributes of an object. 5. The analysis of many possible constructors are introduced to allowusers to create the fractions with different types of input. Moreover the toString() can be modified to display the special fractions in different forms. 6. Project 1 is a continuation of Step 5 a. The input from keyboard should be moved to Utility b. The new constructor Fraction(String s) should be created by calling Step 6a, and parsing code of strings from main should be moved to this constructor. c. Finally, the toString() is modified in parallel to Step 6b. Note: You must master by redoing all steps 1-5 LectureFeb3-Morning Feb 3 : Lecture & Project 1 Review Analysis of input (from Users) - direct:(int) 2 params, 1 param *no param? *keyboard *(Scanner):String methods *" 2/6 " *"2 /6" *"2 / 6" "4" "20/5" "1 1/2" " 1 1/2" "1/2" " 10/15" // char o Output (display) - simple forms "2/1" 2 "2/4" 1/2 "5 2/6" 5 1/3 4/3 1 1/3 => 11 11 => Project 1: Do all cases in this page. Submit your zip file of the sources (Test.java, Fraction.java, and Utility.java) to spham@csun.edu BEFORE NEXT Thursday (midnight of Wed go to th)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
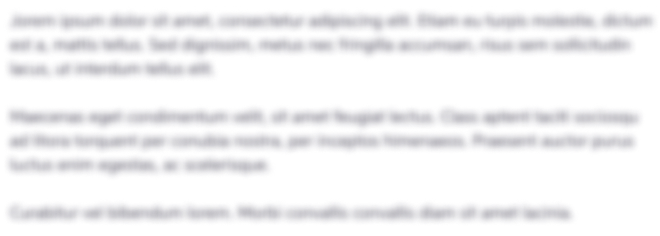
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started