Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a function get _ address 2 that takes a list of House objects and a Person's age, as parameters, and returns the house addresses
Write a function getaddress that takes a list of House objects and a Person's age, as parameters, and returns the house addresses where at least one person of that age lives. If there are no inhabitants in that age in any of the houses it should print the message as shown in the tests.
Example usage:
houses
h House Happy St Blackwood'
h House Agnes Av Aldinga'
h House Daw St Aldinga'
h House Oak St Blackwood'
h House Gorge Av Aldinga'
h House Waterman St Aldinga'
c CarFord'Mustang',
c CarFord'Fiesta',
c CarToyota'Camry',
c CarMazdaCx
c CarMazdaCx
c CarMitsubishi'Colt',
c CarToyota'Corolla',
c CarFord'Escape',
c CarFord'Raptor',
c CarHolden'Barina',
p PersonJim
p PersonAshley
p PersonCleo
p PersonJessica
p PersonAdam
p PersonAndrew
p PersonJake
p PersonJoshua
p PersonAmica
p PersonHelen
p PersonBarney
p PersonSandra
p PersonJohn
p PersonPatrick
p PersonSammy
haddcarcc
haddcarc
haddcarc
haddcarccc
haddcarc
haddcarcc
haddinhabitantpppp
haddinhabitantpp
haddinhabitantppp
haddinhabitantp
haddinhabitantpp
haddinhabitantppp
houses.extendhhhhhh
printgetaddresshouses
printgetaddresshouses
output:
Looks like there are no inhabitants of years old.
Daw St Aldinga', Waterman St Aldinga'
Here are the Classes:
class Person:
def initself name, age:
self.name name
self.age age
def strself:
return 'This is self.name strselfage years old.
def isteenagerself:
return self.age
class Car:
def initself make,model,age:
self.make make
self.model model
self.age age
self.owners
def strself:
return 'This is: self.make strselfage years old.
def addownerselfo:
for i in rangeleno:
if isinstanceoiPerson and isinstanceoistr:
self.ownersoi oi
else:
printError: cannot update the list of car owners'
break
def getownerslistself:
olist nname for n in self.owners.keys
return xy for xy in zipolist,self.owners.values
class House:
def initself address:
self.address address
self.inhabitants
self.cars
def strself:
return 'This house is inhabited by strlenselfinhabitants people.
def addinhabitantselfp:
for i in rangelenp:
if isinstancepiPerson:
self.inhabitants.appendpi
else:
printError: cannot update the list of inhabitants'
break
def addcarselfc:
for i in rangelenc:
if isinstanceciCar:
self.cars.appendci
else:
printError: cannot update the list of cars'
break
def getinhabitantsself:
inhabnames nname for n in self.inhabitants
inhabages nage for n in self.inhabitants
return n:a for na in zipinhabnames,inhabages
def getcarsself:
carmakes cmake for c in self.cars
carmodels cmodel for c in self.cars
return xy for x y in zipcarmakes,carmodels
Step by Step Solution
There are 3 Steps involved in it
Step: 1
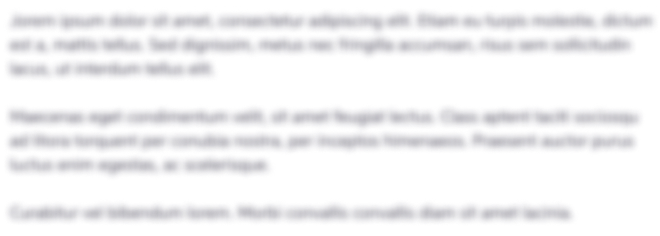
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started