Question
Write a function that deletes the n th element from a linked list. If the linked list doesn't even have n nodes, don't delete any
Write a function that deletes the nth element from a linked list. If the linked list doesn't even have n nodes, don't delete any of them. The function signature is: node *deleteNth(node *head, int n). Try implementing the function iteratively and recursively. (In terms of how to interpret n, you can start counting your nodes from zero or one; your choice.)
I gave it a shot on the iterative version but I'm not getting it. I have no idea where to start on the recursive version. Help
#include
#include
#include
typedef struct node
{
int data;
struct node *next;
} node;
node *create_node(int data)
{
node *new_node = malloc(sizeof(node));
if (new_node == NULL)
{
fprintf(stderr, "Error: Out of memory in create_node() ");
exit(1);
}
new_node->data = data;
new_node->next = NULL;
return new_node;
}
node *tail_insert(node *head, int data)
{
node *temp = head;
if (head == NULL)
return create_node(data);
while (temp->next != NULL)
temp = temp->next;
temp->next = create_node(data);
return head;
}
void print_list(node *head)
{
if (head == NULL)
{
printf("(empty list) ");
return;
}
printf("List = ");
while (head != NULL)
{
printf("%d%c", head->data, (head->next == NULL) ? ' ' : ' ');
head = head->next;
}
}
node *deleteNth(node *head, int n)
{
int i;
node *temp = head;
if (head == NULL)
return NULL;
if (n == 0)
{
head = temp->next;
free(temp);
return head;
}
// Find previous node of the node to be deleted
for (i = 0; temp != NULL && i < n - 1; i++)
temp = temp->next;
// If position is more than number of nodes
if (temp == NULL || temp->next == NULL)
return head;
// Node temp->next is the node to be deleted
// Store pointer to the next of node to be deleted
node *next = temp->next->next;
// Unlink the node from linked list
free(temp->next); // Free memory
temp->next = next; // Unlink the deleted node from list
return head;
}
int main(void)
{
int i;
node *head = NULL, *tail = NULL;
int a[] = {1, 5, 7, 8, 4, 12, 2};
srand((unsigned int)time(NULL));
for (i = 0; i < 7; i++)
{
tail = tail_insert(tail, a[i]);
if (head == NULL)
head = tail;
}
print_list(head);
head = deleteNth(head, 6);
print_list(head);
printf(" ");
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
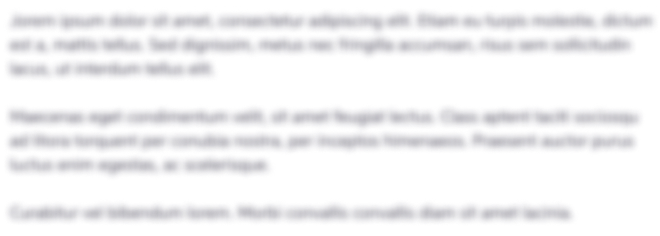
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started