Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a function that receives a StaticArray where the elements are in sorted order, and returns a new StaticArray with squares of the values from
Write a function that receives a StaticArray where the elements are in sorted order, and
returns a new StaticArray with squares of the values from the original array, sorted in
nondescending order. The original array must not be modified.
You may assume that the input array will have at least one element, will contain only
integers in the range
and that elements of the input array are already in
nondescending order. You do not need to write checks for these conditions.
Implement a FAST solution that can process at least elements in a reasonable
amount of time under a minute
Note that using a traditional sorting algorithm even a fast sorting algorithm like merge sort
or shell sort will not pass the largest test case of elements. Also, a solution
using countsort as a helper method will also not work here, due to the wide range of
values in the input array.
For full credit, the function must be implemented with ON complexity.class StaticArrayExceptionException:
Custom exception for Static Array class.
Any changes to this class are forbidden.
pass
class StaticArray:
Implementation of Static Array Data Structure.
Implemented methods: get set length
Any changes to this class are forbidden.
Even if you make changes to your StaticArray file and upload to Gradescope
along with your assignment, it will have no effect. Gradescope uses its
own StaticArray file a replica of this one and any extra submission of
a StaticArray file is ignored.
def initself size: int None:
Create array of given size.
Initialize all elements with values of None.
If requested size is not a positive number,
raise StaticArray Exception.
if size :
raise StaticArrayExceptionArray size must be a positive integer'
# The underscore denotes this as a private variable and
# private variables should not be accessed directly.
# Use the length method to get the size of a StaticArray.
self.size size
# Remember, this is a builtin list and is used here
# because Python doesn't have a fixedsize array type.
# Don't initialize variables like this in your assignments!
self.data None size
def iterself None:
Disable iterator capability for StaticArray class.
This means loops and aggregate functions like
those shown below won't work:
arr StaticArray
for value in arr: # will not work
minarr # will not work
maxarr # will not work
sortarr # will not work
return None
def strself str:
Override string method to provide more readable output."""
return fSTATARR Size: selfsizeselfdata
def getself index: int:
Return value from given index position.
Invalid index raises StaticArrayException.
if index or index self.length:
raise StaticArrayExceptionIndex out of bounds'
return self.dataindex
def setself index: int, value None:
Store value at given index in the array.
Invalid index raises StaticArrayException.
if index or index self.length:
raise StaticArrayExceptionIndex out of bounds'
self.dataindex value
def getitemself index: int:
Enable bracketed indexing."""
return self.getindex
def setitemself index: int, value: object None:
Enable bracketed indexing."""
self.setindex value
def lengthself int:
Return length of the array number of elements
return self.size
if namemain:
# Using the Static Array #
# Can use either the getset methods or bracketed indexing #
# Both are public methods; using the calls the corresponding getset #
# Create a new StaticArray object is the default size
arr StaticArray
# These two statements are equivalent
arr.set 'hello'
arr 'hello'
# These two statements are equivalent
printarrget
printarr
# Print the number of elements stored in the array
printarrlength
# Create a new StaticArray object to store elements
arr StaticArray
# Set the value of each element equal to its index multiplied by
for index in rangearrlength:
arrindex index
# Print the values of all elements in reverse order
for index in rangearrlength:
printarrindex
Step by Step Solution
There are 3 Steps involved in it
Step: 1
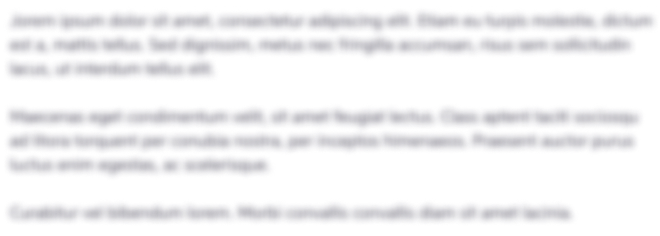
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started