Question
Write a java code for below instruction: For this programming assignment, you'll create a JavaFX application that displays and controls Vehicle objects created by your
Write a java code for below instruction:
For this programming assignment, you'll create a JavaFX application that displays and controls Vehicle objects created by your Programming Assignment 2 Vehicle class. The diagram below shows what your GUI should look like. Your GUI should allow the user to create up to five vheicles. As each vehicle is created, it is represented as a colored circle in the central drawing pane and as a label in the left area of the GUI (with the same background color as the circle). In the figure below, the user has just used the Create Vehicle button to create the first vehicle at location (100, -120) with velocity 50 and direction 90. The first vehicle color is red, so the vehicle is represented by a red circle in the central drawing pane and via the red label on the left.
Details of the GUI's operation: Your application must obey the following rules:
Your class for the GUI must be called VehicleGUI and it must be part of the vehicle package that contains the Vehicle class.
There can be only five vehicles created by your GUI. If the user tries to create a sixth vehicle; your GUI will simple ignore the request.
Each of the vehicles created must have a unique color chosen from the following: RED, GREEN, BLUE, PURPLE, PINK.
The central drawing pane should be 300 by 300 and surrounded by a black border
When the Create Vehicle button is press, your GUI should attempt to create a vehicle. In order to succesfully create a vehicle, the following must hold: 1) there must be less than five vehicles, the four text fields at the top of the GUI (X Coordinate, Y Coordinate, Velocity, and Direction) plus the Vehicle Number field must be filled with numeric values and the Y Coordinate value must be zero or less. If any of these conditions do not hold, simply ignore the request. When creating the vehicle, negate the Y Coordinate value, which will produce a Y Coordinate value suitable for JavaFX. After the vehicle is created, draw the circle with one of the remaining colors and create the label for the vehicle.
When the Delete Vehicle button is pressed, your GUI should attempt to delete the vehicle identified by the number in the Vehicle Number text field. If the Vehicle Number text field is empty or does not hold a number, simply ignore the request. Remove the circle and label for this vehicle and return its color to the available colors.
When the Accelerate button is pressed, your GUI should attempt to change the velocity of the vehicle identified by the Vehicle Number field, using the values in the Accelerate and Time text fields. If any of these three text fields do not contain numeric values, simply ignore the request. Note that this will not have an effect on the GUI.
When the Change Direction button is pressed, your GUI should attempt to change the direction of the vehicle identified by the Vehicle Number field, using the value in the Direction text field. If either of these text fields do not contain numeric values, simply ignore the request. Note that this will not have an effect on the GUI.
When the Move Vehicle is pressed, your GUI should attempt to move the vehicle identified by the Vehicle Number field, using the value in the Time field as the amount of time the vehicle travels. If either of the text fields do not contain numeric values, simply ignore the request. If either of the new X, Y values is outside the drawing area (the values are negative or greater than 300, ignore the request. If the request is successful, change the vehicle's coordinates and also the representing circle's coordinates.
When the Quit button is pressed, terminate the application. You can use System.exit() to do this.
[Can anyone help me to get answer for this java program]
Vehicle.java file
package vehicle; public class Vehicle { // should be private //make the given variables private private double velocity; private double x; private double y; private double direction;
//class constructor public Vehicle(double vehObj, double x, double y, double d) {
// d is given as degrees, you should store it internally as radians //set the values this.velocity = vehObj; this.x = x; this.y = y;
//set the radian of direction this.direction = d * ( 3.14 / 180.0 );
}
//The method to get velocity public double getVelocity() { return this.velocity; }
//The method to get position public double getDirection() {
//return in degree return this.direction / ( 3.14 / 180.0 ); }
public double [] getPosition() { // return an array of doubles as the x, y pair double ar[] = {this.x, this.y}; return ar; }
public void changeVelocity(double accelartion, int time) { // acceleration can be positive or negative // velocity is changed based on duration of acceleration // vehObj = vo + at; this.velocity = this.velocity + accelartion * time; } public void changeDirection(double nd) { // change the direction // the parameter nd is in degrees // store the result in radians direction = Math.toRadians(nd); }
public double [] showNewPosition(int t) { // return the new x,y pair based on travelling for time t // based on current velocity, direction and position // does not change the vehicle's position
double dist = this.velocity * t; double xVal = dist * Math.cos(this.direction); double yVal = dist * Math.sin(this.direction); double arryNew[] = {Math.round(xVal), Math.round(yVal)}; return arryNew; }
public void changePosition(int t) { // changes the x,y pair based on travelling for time t // based on current velocity, direction and position.
//update the position double dist = this.velocity * t; x = Math.round(dist * Math.cos(this.direction)); y = Math.round(dist * Math.sin(this.direction)); } public String toString() {
// override the Object toString method to produce a //formatted string String newStr=""; // representation of a vehicle: newStr=""; // "
// overloading of toString that takes an array of two doubles // and returns a position string: "(x,y)" String cc=""; cc=cc+"("+p[0]+","+p[1]+")"; return cc; } private double rndTo2DP(double d) { // rounds d to have two decimal places return Math.round(100*d)/100.0; }
}
Vehicle Display rection 90 Y Coordinate -120 Velocity 50 x Coordinate 100 Acceleration ime Direction Change Vehicle Number Create Vehicle Delete Vehicle Accelerate Change Direction Move Vehicle QuitStep by Step Solution
There are 3 Steps involved in it
Step: 1
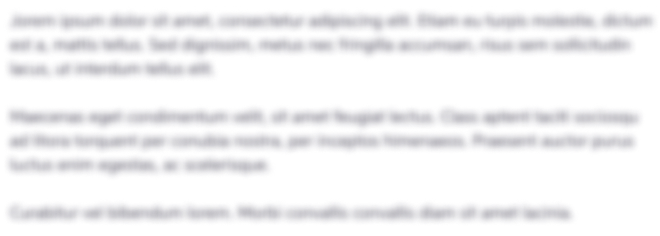
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started