Question
Write a menu driven C program, for manipulating the records of students in a classroom. The class may include at most 25 students. (Using unions
Write a menu driven C program, for manipulating the records of students in a classroom. The class may include at most 25 students.
(Using unions and enumerated data) types,
define a structure for students with different majors Each student should have a student name, student ID, student major (Biology, Mathematics, or Chemistry) and student average. If the student has a (Biology major), then they should have marks for 106 and one for 206. Those having a (Mathematics major) should have marks for 101 and 102. Those with a (Chemistry major) should have marks for 101, 102, and 113. ----- #( a separate functions to perform the following menu) 1)Add a new student to the classroom. 2) Delete a student from the classroom. 3) Modify the record of a particular student given his name or ID number. 4)Print the record of a particular student given his name or ID number. 5)Print the records of all the students in the classroom. 6)Exit the program #( that mean 6 function (adding , deleting, modifying ,print particular student ,print all student and Exit))
IMPORTANT REMAKRS: 1'When adding a new student, your program should input a student name and their ID, enter their major along with the marks needed according to the major, and then compute the average according to the marks stored in the structure. Only one student is added with each add option. No two students share the exact name. No two students share the exact ID number. Search for the student using a recursive sequential search algorithm to make sure if they exist.
2-The user is allowed to delete a student based on either the student's unique name or ID number. The order of the students in the array should not be changed. Use shifting within the array to shift the elements so as to delete the unwanted student from the array.
3-The user can modify the marks of the student given his name or ID number. The marks modified are dependent on the major of the student. Make sure to recalculate the average after the modification. 4- Implement a recursive function to print the records of all the students in the classroom.
5- Make sure all error messages are accounted for (e.g. printing the students from an empty list, deleting a non-existing student, etc.)
------------------ THE BEGINNING SHOULD BE LIKE :
typedef struct { Char name [20]; int id; Double avrage;
enum { biology=1, Mathematics, Chemistry } major ; Union { Struct { b1 ,b2}bioMarks; Struct { m1 ,m2}mathMarks; Struct { c1 ,c2, c3}chemMarks; }Sub; } students ;
((I need the function and it's main )) ((Make sure that there are 6 function for (adding , deleting, modifying ,print particular student ,print all student and Exit))
#IN C PROGRAMMING LANGUAGE
//--------------------------------------- my code is :
#include
#include
typedef struct{
enum{Biology=1,Mathematic,Chemistry}Major;
char name [20];
int id;
float avg;
union{
struct{float b106,b206; }bio;
struct{float m101,m102; }math;
struct{float c101,c102,c113; }chym;
}Marks;
}student;
int CheckName(student A[],char check [],int n){
if (n==0)
return -1;
else{
if(strcmp(A[n-1].name,check)==0)
return n-1;
else return CheckName(A,check,n-1);
}}
int CheckID(student A[],int key,int n) //n length of Array.
{
if (n==0)
return -1;
else {
if (A[n-1].id==key)
return n-1;
else return CheckID(A,key,n-1);
}}
void BIOMARKS(student A[],int n){
printf("Enter the student mark for Bio 106 ");
scanf("%f",&A[n].Marks.bio.b106);
printf("Enter the student mark for Bio 206 ");
scanf("%f",&A[n].Marks.bio.b206);
break;
}
void MATHMARKS(student A[],int n){
printf("Enter the student mark for math 101 ");
scanf("%f",&A[n].Marks.math.m101);
printf("Enter the student mark for math 102 ");
scanf("%f",&A[n].Marks.math.m102);
}
void CHYMMARKS(student A[],int n){
printf("Enter the student mark for chymistry 101 ");
scanf("%f",&A[n].Marks.chym.c101);
printf("Enter the student mark for chymistry 102 ");
scanf("%f",&A[n].Marks.chym.c102);
printf("Enter the student mark for chymistry 113 ");
scanf("%f",&A[n].Marks.chym.c113);
}
void ADD(student A[],int n){
printf("Add name ");
gets(A[n].name);
CheckName(A,A[n].name,n);
if (CheckName(A,A[n].name,n)!=-1)
printf("The student name already exist");
else{
printf("ID ");
scanf("%d",&A[n].id);
CheckID(A,A[n].id,n);
if (CheckID(A,A[n].id,n)!=-1)
printf("The student ID already exist");
else{
do {
printf("1-Biology. ");
printf("2-Mathmatics. ");
printf("3-Chemistry. ");
scanf("%d",&A[n].Major);
if(A[n].Major>3||A[n].Major<1)
printf("Invalid choice, Please Choose a number from the menu... ");
}while(A[n].Major>3||A[n].Major<1);
switch (A[n].Major){
case 1:
BIOMARKS(A,n);
break;
case 2:
MATHMARKS(A,n);
break;
case 3:
CHYMMARKS(A,n);
}
}
}
}
float avgmarks(student A[],int n){
switch (A[n].Major){
case 1:
return (A[n].Marks.bio.b106+A[n].Marks.bio.b206)/2;
break;
case 2:
return (A[n].Marks.math.m101+A[n].Marks.math.m102)/2;
break;
case 3:
return (A[n].Marks.chym.c101+A[n].Marks.chym.c102+A[n].Marks.chym.c113)/3;
}
}
void Delet(student A[],n){
printf("Choose how would you to delete.. ");
int choice;
do {
printf("1- By student name ");
printf("2- By student ID");
scanf("%d",&choice);
if (choice >2 || choice <0)
printf("Invalid choice ");
}while(choice >2 || choice <0);
}
void Mod(){
}
void PrintS(student A[],int n){
printf("The student name is: %s",A[n].name);
printf("The student ID is %d",A[n].id);
printf("The student major is ");
switch (A[n].Major){
case 1:
printf("Biology. ");
printf("The student mark for Bio 106 is %f ",A[n].Marks.bio.b106);
printf("The student mark for bio 206 is %f ",A[n].Marks.bio.b206);
printf("The student average is ");
printf("%f ",avgmarks(A,n));
break;
case 2:
printf("Mathmatics. ");
printf("The student mark for Mathmatic 101 is %f ",A[n].Marks.math.m101);
printf("The student mark for Mathmatic 102 is %f ",A[n].Marks.math.m102);
printf("The student average is ");
printf("%f ",avgmarks(A,n));
break;
case 3:
printf("Chymistry. ");
printf("The student mark for chymistry 101 is %f ",A[n].Marks.chym.c101);
printf("The student mark for chymistry 102 is %f ",A[n].Marks.chym.c102);
printf("The student mark for chymistry 113 is %f ",A[n].Marks.chym.c113);
printf("The student average is ");
printf("%f ",avgmarks(A,n));
}
}
int main(){
student S[3];
int Choice;
int n=0;
char dummy;
do {
printf("1- Add a new Student to the classroom. ");
printf("2- Delete a Student from the classroom. ");
printf("3- Modify a Student record in the classroom. ");
printf("4- Print the record of a partical Student. ");
printf("5- Print the records of all Students in the classroom. ");
printf("6- Quit. ");
printf("Enter the number of your Choice.. ");
scanf("%d",&Choice);
if (Choice <1 || choice>6)
printf("Invalid choice, Please Choose a number from the menu... ");
}while(Choice <1 || choice>6);
switch (Choice){
case 1:
scanf("%c",&dummy);
ADD(S,n);
break;
case 2:
printf("deleted ");
break;
case 3:
printf("modified ");
break;
case 4:
PrintS(S,n);
break;
case 5:
for (int i=0; i PrintS(S,i); } break; case 6: return 0; } } I have problem with the modifying and delete can some one help me pls to complete my code :)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
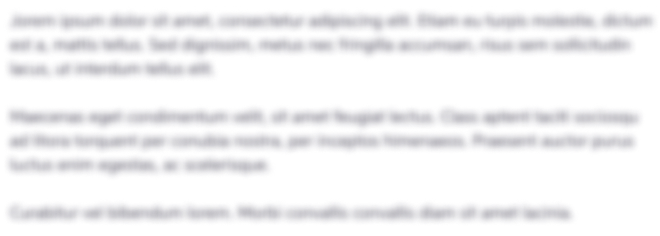
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started