Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a method countDuplicates that returns the number of duplicates in a sorted list. The list will be in sorted order, so all of
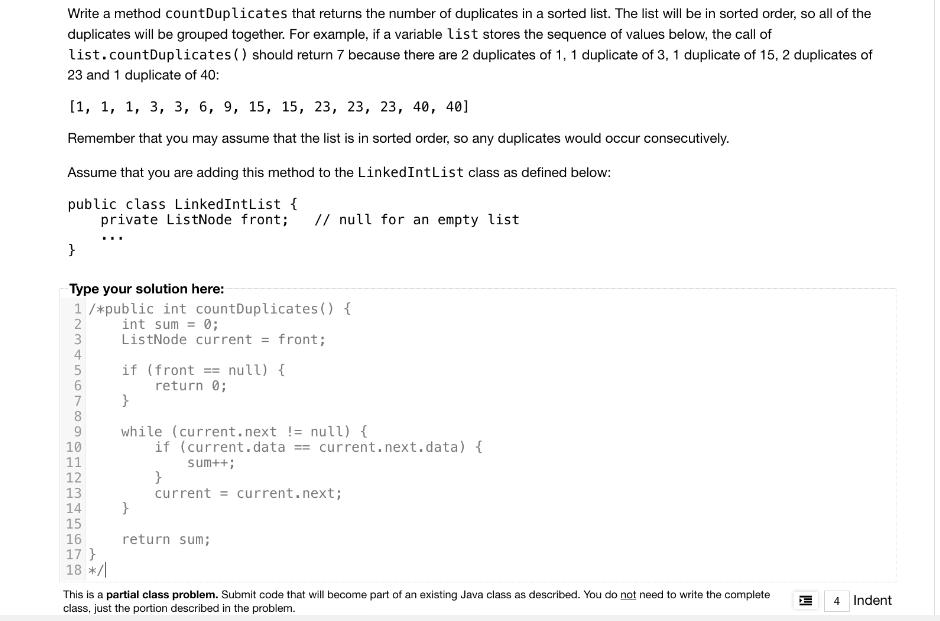
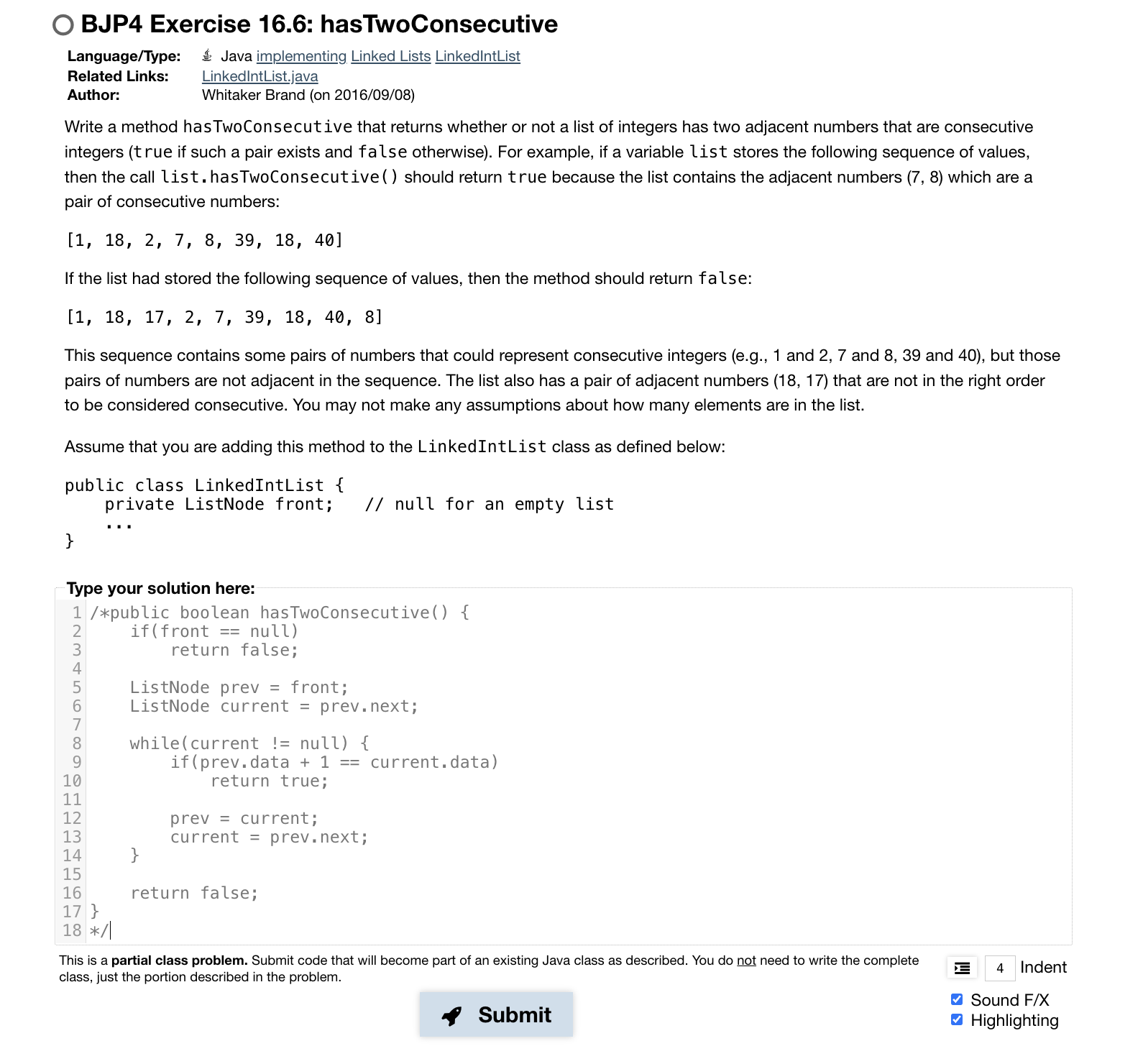
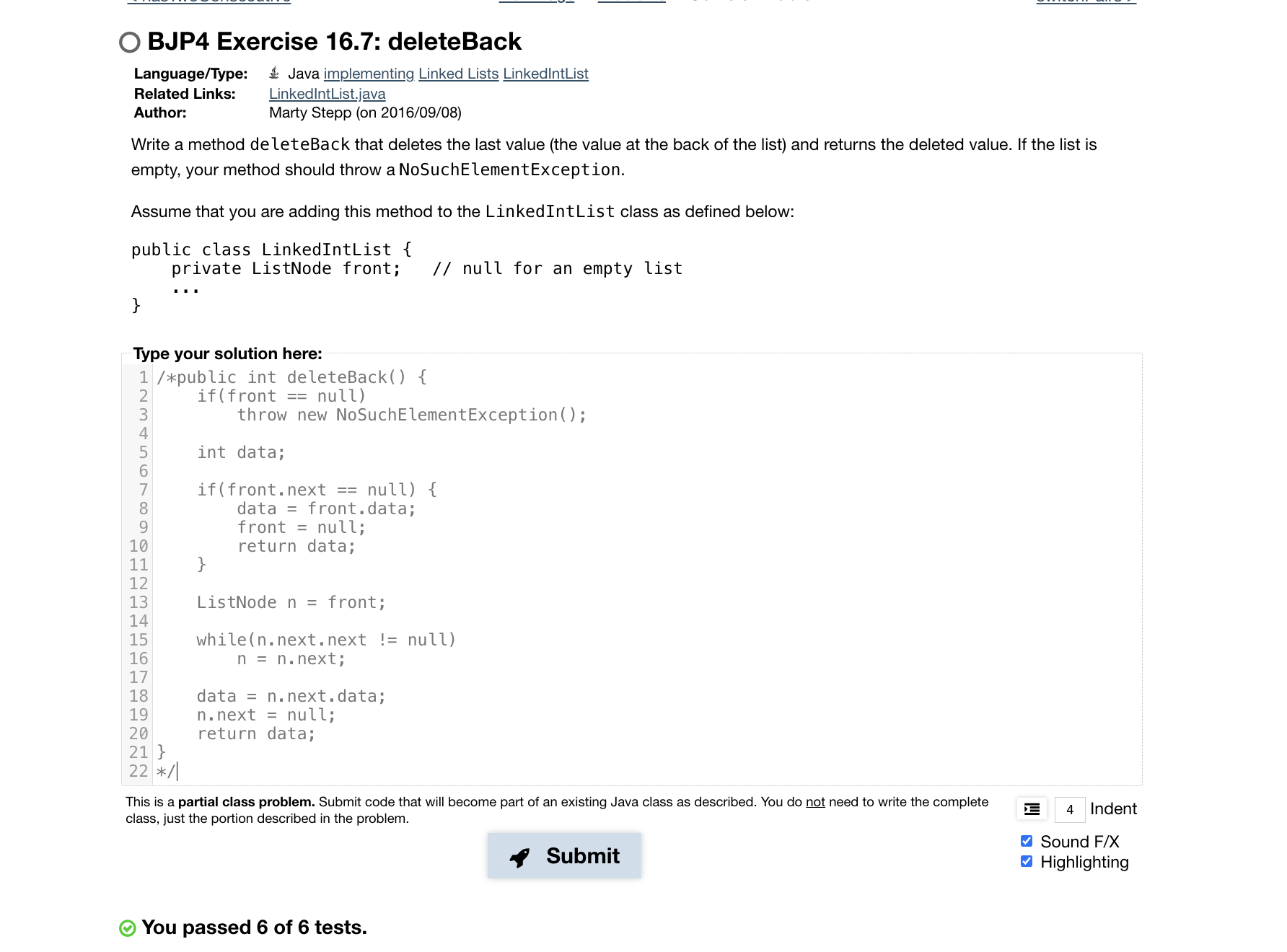
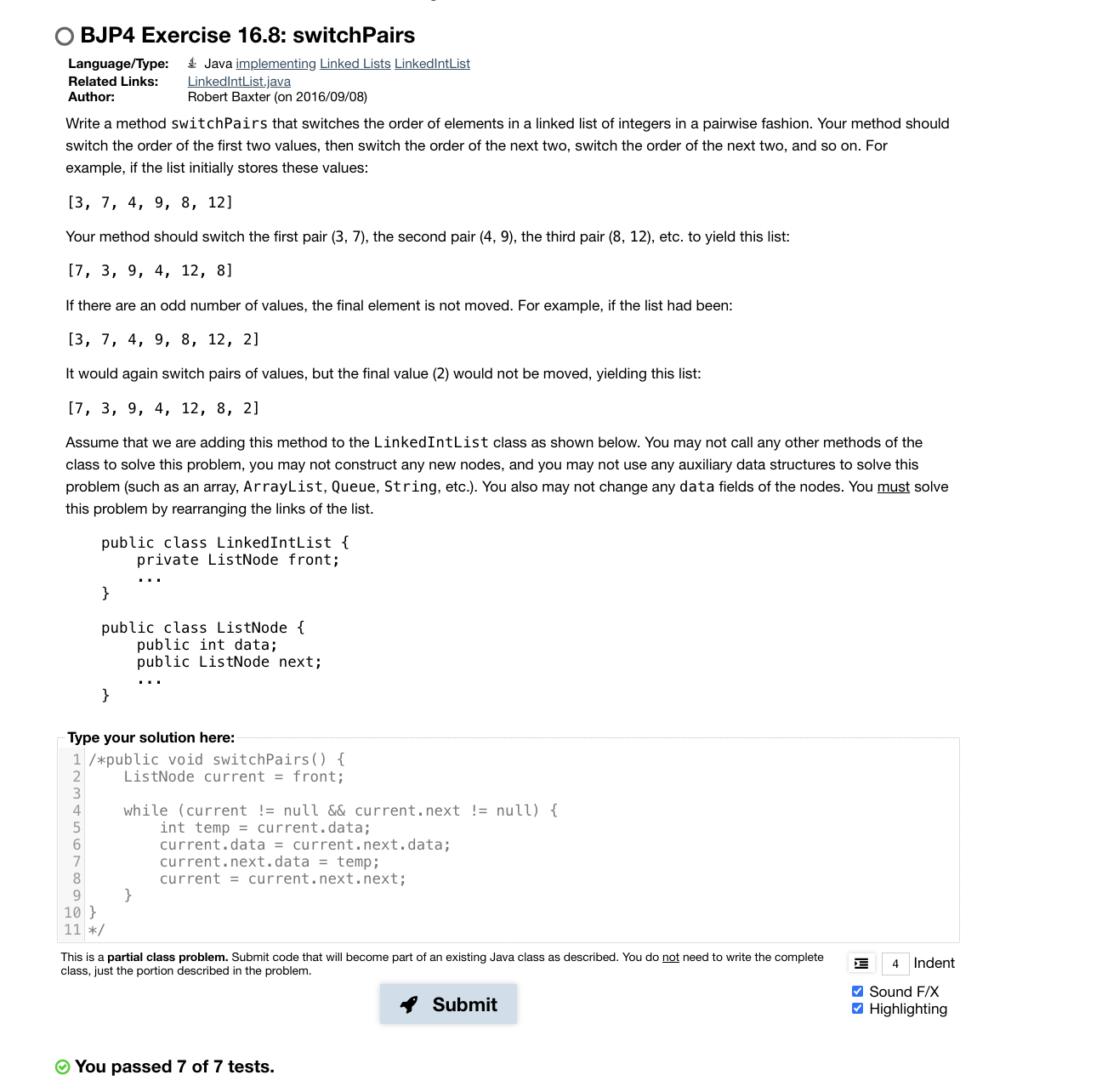
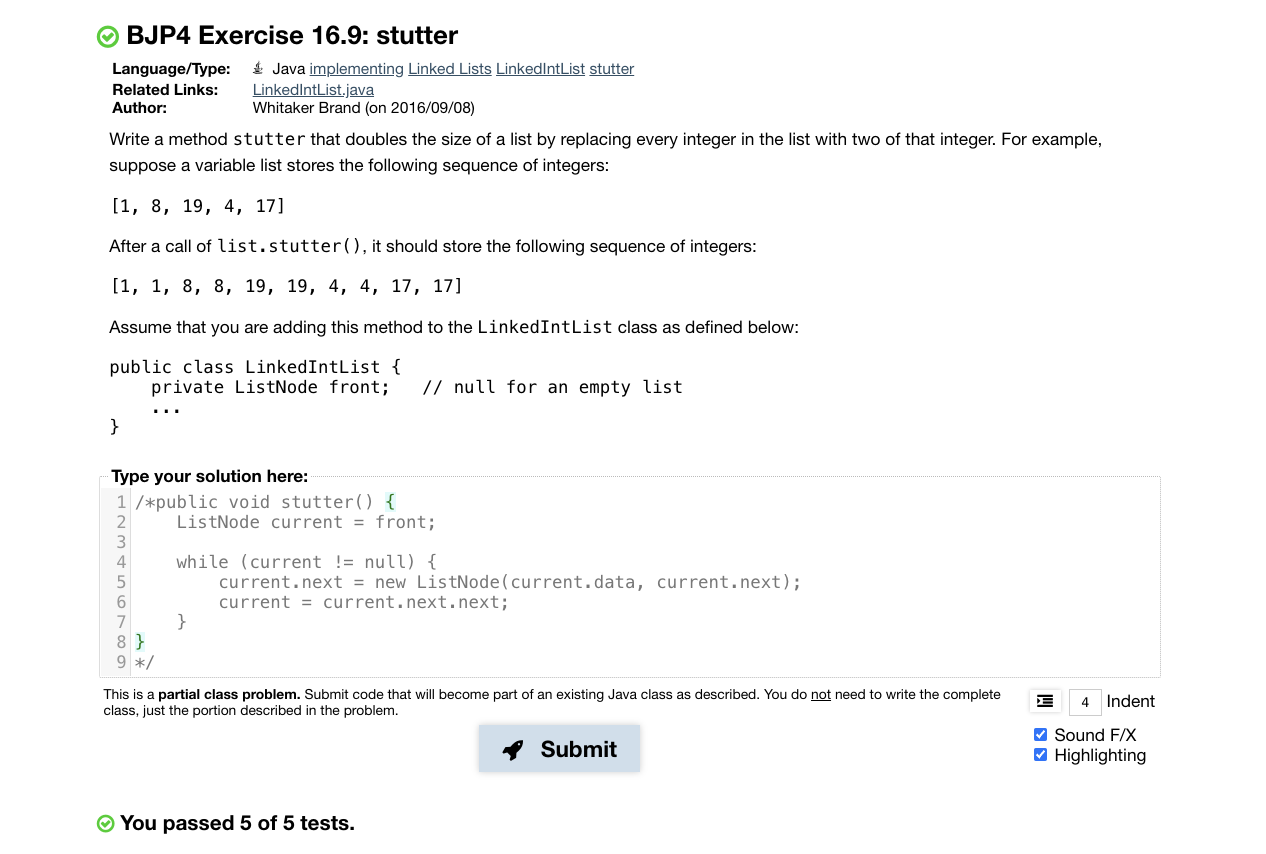
Write a method countDuplicates that returns the number of duplicates in a sorted list. The list will be in sorted order, so all of the duplicates will be grouped together. For example, if a variable list stores the sequence of values below, the call of list.countDuplicates () should return 7 because there are 2 duplicates of 1, 1 duplicate of 3, 1 duplicate of 15, 2 duplicates of 23 and 1 duplicate of 40: [1, 1, 1, 3, 3, 6, 9, 15, 15, 23, 23, 23, 40, 401 Remember that you may assume that the list is in sorted order, so any duplicates would occur consecutively. Assume that you are adding this method to the LinkedIntList class as defined below: public class LinkedIntList { private ListNode front; // null for an empty list } Type your solution here: 1/*public int countDuplicates() { int sum= 0; ListNode current = front; 2 3 4 5 6 LLLLLLL 7 8 T06X 9 10 11 12 13 14 15 16 17 } 18 */| if (front == null) { return 0; } while (current.next != null) { if (current. data == current.next.data) { sum++; } current current.next; } return sum; This is a partial class problem. Submit code that will become part of an existing Java class as described. You do not need to write the complete class, just the portion described in the problem. E 4 Indent OBJP4 Exercise 16.6: hasTwoConsecutive Language/Type: Java implementing Linked Lists LinkedIntList Related Links: LinkedIntList.java Author: Whitaker Brand (on 2016/09/08) Write a method has TwoConsecutive that returns whether or not a list of integers has two adjacent numbers that are consecutive integers (true if such a pair exists and false otherwise). For example, if a variable list stores the following sequence of values, then the call list.hasTwoConsecutive() should return true because the list contains the adjacent numbers (7, 8) which are a pair of consecutive numbers: [1, 18, 2, 7, 8, 39, 18, 40] If the list had stored the following sequence of values, then the method should return false: [1, 18, 17, 2, 7, 39, 18, 40, 8] This sequence contains some pairs of numbers that could represent consecutive integers (e.g., 1 and 2, 7 and 8, 39 and 40), but those pairs of numbers are not adjacent in the sequence. The list also has a pair of adjacent numbers (18, 17) that are not in the right order to be considered consecutive. You may not make any assumptions about how many elements are in the list. Assume that you are adding this method to the LinkedIntList class as defined below: public class LinkedIntList { private ListNode front; // null for an empty list } Type your solution here: 1/*public boolean has Two Consecutive() { if (front == null) return false; 2 34567 8 9 10 11 12 13 14 15 16 17 } 18 */ ListNode prev = front; ListNode current = prev.next; while (current != null) { } if (prev.data + 1 ==current.data) return true; prev = current; current= prev.next; return false; This is a partial class problem. Submit code that will become part of an existing Java class as described. You do not need to write the complete class, just the portion described in the problem. Submit E 4 Indent Sound F/X Highlighting O BJP4 Exercise 16.7: deleteBack Language/Type: Related Links: Author: Write a method deleteBack that deletes the last value (the value at the back of the list) and returns the deleted value. If the list is empty, your method should throw a NoSuch ElementException. Assume that you are adding this method to the LinkedIntList class as defined below: public class LinkedIntList { private ListNode front; // null for an empty list } Type your solution here: 1/*public int deleteBack() { if (front == null) 2 3 4 Java implementing Linked Lists LinkedIntList LinkedIntList.java Marty Stepp (on 2016/09/08) 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 } 22 */ } throw new NoSuch ElementException(); int data; if(front.next == null) { data front.data; front= null; return data; ListNode n = front; while(n.next.next!= null) n = n.next; data = n.next.data; n.next = null; return data; This is a partial class problem. Submit code that will become part of an existing Java class as described. You do not need to write the complete class, just the portion described in the problem. You passed 6 of 6 tests. Submit Indent Sound F/X Highlighting 4 O BJP4 Exercise 16.8: switchPairs Language/Type: * Java implementing Linked Lists LinkedIntList LinkedIntList.java Robert Baxter (on 2016/09/08) Related Links: Author: Write a method switchPairs that switches the order of elements in a linked list of integers in a pairwise fashion. Your method should switch the order of the first two values, then switch the order of the next two, switch the order of the next two, and so on. For example, if the list initially stores these values: [3, 7, 4, 9, 8, 12] Your method should switch the first pair (3, 7), the second pair (4, 9), the third pair (8, 12), etc. to yield this list: [7, 3, 9, 4, 12, 8] If there are an odd number of values, the final element is not moved. For example, if the list had been: [3, 7, 4, 9, 8, 12, 2] It would again switch pairs of values, but the final value (2) would not be moved, yielding this list: [7, 3, 9, 4, 12, 8, 2] Assume that we are adding this method to the LinkedIntList class as shown below. You may not call any other methods of the class to solve this problem, you may not construct any new nodes, and you may not use any auxiliary data structures to solve this problem (such as an array, ArrayList, Queue, String, etc.). You also may not change any data fields of the nodes. You must solve this problem by rearranging the links of the list. public class LinkedIntList { private ListNode front; 2 3 } public class ListNode { public int data; public ListNode next; } Type your solution here: 1/*public void switchPairs () { ListNode current = front; 7 8 9 10} 11 */ while (current != null && current.next != null) { int temp = current.data; current.data = current.next.data; } current.next. data = temp; current current.next.next; This is a partial class problem. Submit code that will become part of an existing Java class as described. You do not need to write the complete class, just the portion described in the problem. You passed 7 of 7 tests. Submit 4 Indent Sound F/X Highlighting E BJP4 Exercise 16.9: stutter Language/Type: Related Links: Author: Java implementing Linked Lists LinkedIntList stutter LinkedIntList.java Whitaker Brand (on 2016/09/08) Write a method stutter that doubles the size of a list by replacing every integer in the list with two of that integer. For example, suppose a variable list stores the following sequence of integers: [1, 8, 19, 4, 17] After a call of list.stutter(), it should store the following sequence of integers: [1, 1, 8, 8, 19, 19, 4, 4, 17, 17] Assume that you are adding this method to the LinkedIntList class as defined below: public class LinkedIntList { private ListNode front; // null for an empty list } Type your solution here: 1/*public void stutter() { 4 7 8} 9 */ ListNode current = front; while (current != null) { current.next = new ListNode (current.data, current.next); current = current.next.next; } This is a partial class problem. Submit code that will become part of an existing Java class as described. You do not need to write the complete class, just the portion described in the problem. You passed 5 of 5 tests. Submit E 4 Indent Sound F/X Highlighting
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Heres the implementation of the countDuplicates method public int countDup...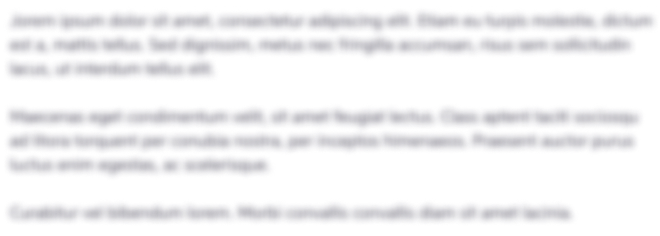
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started