Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a modularized, menu-driven program to read a file with an unknown number of inventory records using an array of structs/objects and an array of
Write a modularized, menu-driven program to read a file with an unknown number of inventory records using an array of structs/objects and an array of pointers.
in C++
- An item may have three statuses: active, discontinued, or recalled
- Menu (use a switch statement) options to
- print all items in the inventory unsorted
- print invalid records from an error file
- search for an item by ID or name
- print a report with the following details
- number of unique items in the inventory
- the total worth of the inventory and the total count of all items in the inventory
- quit the program
- A user should be able to run many as many times as the user wants; print the menu every time
- Implement the menu as a switch statement using enum
- All items are unique
- Record
- Item Id must be 5 characters long; maybe alpha-numeric
- The name may contain only alpha characters
- Quantity and price must be zero (a promotional item, an item is not in stock) or above
- status value is not in the input file
- Reading from the input file and storing the records
- The input file has an unknown number of records of inventory items;
- one record per line in the following order: item ID, item name (one word), quantity on hand, and a price;
- All fields in the input file are separated by a tab (\t) or a blank (up to you)
- the input file may be empty or has more records that your array can store- output the appropriate messages to the user.
- check for the array boundaries when storing the records
- Only go through the file once
- Do not read the file once to count the number of the records, close it, and then read it again
- Store all invalid records along with the error messages in a separate file
- Use an array of structs if you do not know how to write classes yet. Use classes if you have completed CS 216 or similar;
- do not store bad records in the array of records
- read a record into a temp struct; error check the record and only store the record in the array of records if it is valid
- assign "active" status when storing the item in the array of structs/objects
- use enum to store the item status
- Searching
- a user should be able to enter the name of the item using any case, (for example, sTRolleR)
- You may wish to store items in all lower/upper case to expedite the search
- write one search function that can search by ID and name and goes through the array of structs/objects only once
- the search function should return the item subscript- you will reuse the function to "recall" an item
HOW TO APPROACH
- Plan, plan and plan again
- test, test, and test again
- START SMALL and add functionality to your program incrementally, almost one at a time and test it before adding any more functionality; for example,
- write a function to read from a file ( getDta()) into an array of structures
- write a function to print the array of structures (print())
- test getData() and print() functions
- add a function(s) to validate a record (isValidRec()), modify getData() so it calls isValidRec() and stores good records in the array of records and stores bad records in the error file, test again
- modify main by adding a menu to print all inventory, bad records, and to quit, test menu, and the menu options
- write a function to search, add a menu choice to search, test again
- keep adding functionality and corresponding menu choices, keep testing each menu choice as you add them
Record sample:
9971x StroLLer 25 134.78
GENERAL NOTES
- Thoroughly test your program. Your grade partially depends on the quality of your test data.
- This and all other programs in this course must comply with the Guidelines and Standards posted under Resources
- use function prototypes
- function definitions must be below main
- no global variables (including files) except for const
- files must be declared in the functions
- use setw() to format output
- do not mix setw() and '\t'; it could produce different results on different computers
- const may be declared global; if global, do not have to be passed to function; any function has access to global variables output must be well-formatted; all numerical values must be right aligned with 2 decimal places; dots my be on the same vertical line
- Clearly label the output
- All numbers to be printed right-aligned
- vectors and template classes are not allowed
- no dynamic memory allocation
Step by Step Solution
There are 3 Steps involved in it
Step: 1
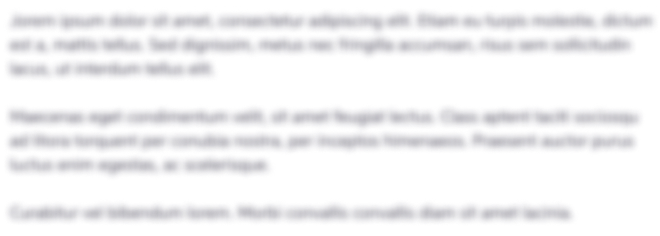
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started