Question
Write a new subroutine in assembly to convert the upper-case letters to lower-case letter. Example of lower case to upper is provided below: Lets look
Write a new subroutine in assembly to convert the upper-case letters to lower-case letter. Example of lower case to upper is provided below:
Lets look at a subroutine to capitalize all the lower-case letters in the string. We need to load each character, check to see if it is a letter, and if so, capitalize it.
Each character in the string is represented with its ASCII code. For example, A is represented with a 65 (0x41), B with 66 (0x42), and so on up to Z which uses 90 (0x5a). The lower case letters start at a (97, or 0x61) and end with z (122, or 0x7a). We can convert a lower case letter to an upper case letter by subtracting 32.
__asm void my_strcpy(const char *src, char *dst){ loop LDRB r2, [r0] ; Load byte into r2 from mem. pointed to by r0 (src pointer) ADDS r0, #1 ; Increment src pointer STRB r2, [r1] ; Store byte in r2 into memory pointed to by (dst pointer) ADDS r1, #1 ; Increment dst pointer CMP r2, #0 ; Was the byte 0? BNE loop ; If not, repeat the loop BX lr ; Else return from subroutine } __asm void my_capitalize(char *str){ cap_loop LDRB r1, [r0] ; Load byte into r1 from memory pointed to by r0 (str pointer) CMP r1, #'a'-1 ; compare it with the character before 'a' BLS cap_skip ; If byte is lower or same, then skip this byte CMP r1, #'z' ; Compare it with the 'z' character BHI cap_skip ; If it is higher, then skip this byte SUBS r1,#32 ; Else subtract out difference to capitalize it STRB r1, [r0] ; Store the capitalized byte back in memory cap_skip ADDS r0, r0, #1 ; Increment str pointer CMP r1, #0 ; Was the byte 0? BNE cap_loop ; If not, repeat the loop BX lr ; Else return from subroutine } /*---------------------------------------------------------------------------- MAIN function *----------------------------------------------------------------------------*/ int main(void){ const char a[] = "Hello world!"; char b[20]; my_strcpy(a, b); my_capitalize(b); while (1) ; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
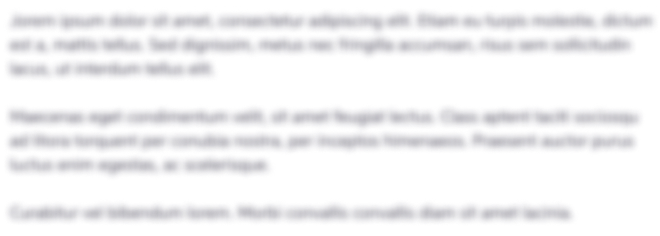
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started