Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a program called SDESencrypt.java or sdesencrypt.py that performs the entire SDES encryption algorithm. You may use the method you wrote in the last assignment.
Write a program called SDESencrypt.java or sdesencrypt.py that performs the entire SDES encryption
algorithm. You may use the method you wrote in the last assignment. You will need to:
add a method that takes as a parameter the bit input key and that returns a list of the four
bit subkeys
add a method that takes as parameters the bit input plaintext and the bit input key and
that returns the bit ciphertext
As usual, write a main methodmain section that gets the plaintext and the key as integer values from
command line parameters. It calls the second method described above and then prints the ciphertext as
a decimal integer and as a binary string.
Last Program was
public class Sdesround
private static in assignment final int S
Row
Row
;
private static final int S
Row
Row
;
Expand bits to eight bits by the predefined method.
private static int expandint input
return input & b
input & b
input & b
input & b
input & b
input & b
input & b
input & b;
Function fRi Ki
private static int functionFint input, int key
int expandedInput expandinput;
int temp expandedInput key;
Split temp into two bit values for the Sboxes.
int lefttemp & b;
int right temp & b;
Determine the row and column for S and S
int rowleft & b;
int rowright & b;
int col left & b;
int col right & b;
Get values from Sboxes and concatenate.
return Srowcol Srowcol;
Round of simplified DES.
public static int desRoundint input, int key
int Linput & b;
int R input & b;
int R L functionFR key;
int L R; As per definition Li Ri
return L R;
public static void mainString args
if argslength
System.out.printlnUsage: java Sdesround bit input as integerbit key as integer;
return;
Convert input to integers
int input Integer.parseIntargs;
int key Integer.parseIntargs;
Validate bit length
if input b key b
System.out.printlnInput must be a bit integer and key must be a bit integer.";
return;
one round of simplified DES encryption
int result desRoundinput getRoundKeykey; Assuming round here for simplicity
Print binary and decimal representations of the result
System.out.printlnBinary: b Integer.toBinaryStringresult decimal: result;
Function to get the Ki for the ith round
private static int getRoundKeyint key, int round
Left circular shift
int roundShift round ; There are bits in the key
return key roundShiftkey roundShift & b;
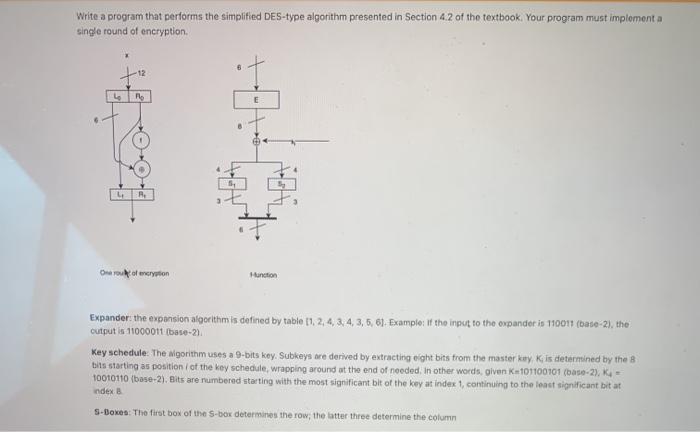
Step by Step Solution
There are 3 Steps involved in it
Step: 1
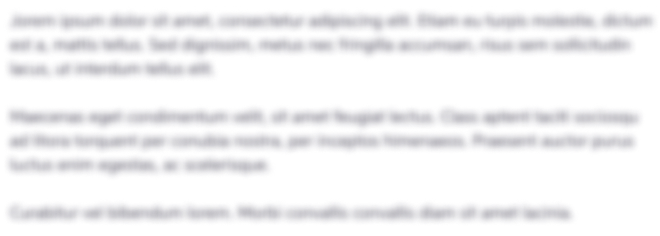
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started