Question
Write a program called Triangles.java that will generate information about triangles. Requirements: -Use a main class for accepting inputs and validating inputs Create an object
Write a program called Triangles.java that will generate information about triangles.
Requirements:
-Use a main class for accepting inputs and validating inputs
Create an object of type class triangle
Prompt the user to enter the three sides of a triangle.
Prompt the user for the base and the height
Accept decimal values
Validate input using try-catch and also nested-if statements as needed
If the user enters invalid data, the application displays an appropriate error message and prompts the user again until the user enters valid data.
- Use a method for evaluating what type of triangle it is (call the method via the object, NO STATIC METHOD)
- Use a method for calculating the area = (base * height)/2 (call the method via the object, NO STATIC METHOD)
- Use a method for calculating the perimeter = (length1 + length2 + length3) (call the method via the object, NO STATIC METHOD)
-After displaying all the triangle information, the application prompts the user to continue.
-When the user chooses not to continue, the application displays a goodbye message
***************************
Output :
Welcome to the Triangles program
Enter length 1: 3
Enter length 2: 5
Enter length 3: 5
Enter the base: 5
Enter the height: 8
---------------------
The triangle is Isosceles since it has two equal length sides. Isosceles triangle also has two equal angles
The area is: 20
The permimeter is: 13
Continue? (y/n): y
Enter length 1: 10
Enter length 2: 10
Enter length 3: 10
Enter the base: 10
Enter the height: 7
---------------------
The triangle is Equilateral since it has equal length on all three sides. All Equilateral triangle are equiangular triangles.
The area is: 35
The permimeter is: 30
Continue? (y/n): y
Enter length 1: 10
Enter length 2: 3
Enter length 3: 9
Enter the base: 10
Enter the height: 7
---------------------
The triangle is Scalene with all no equal side.
Scalene triangles have no equal angles.
The area is: 35
The permimeter is: 22
Continue? (y/n): n
Goodbye.
*****************************
**PROGRAM TEMPLATE**
package triangles; import java.util.Scanner; public class Triangles { private double area; private double permimeter; //CREATE A DEFAULT CONSTRUCTOR TO INITIALIZE area and permimeter //CREATE THE TWO SETTER METHODS FOR area and permimeter //CREATE THE TWO GETTER METHODS FOR area and permimeter public void triangleType(double side1, double side2, double side3) { if (side1 == side2 && side1 == side3) { System.out.println("The triangle is Equilateral since it has equal length on all three sides."); System.out.println("All Equilateral triangle are equiangular triangles."); } else { if (side1 == side2 && side1 != side3 || side1 != side2 && side2 == side3 || side1 == side3 && side1 != side2) { System.out.println("The triangle is Isosceles since it has two equal length sides."); System.out.println("Isosceles triangle also has two equal angles"); } else { if (side1 != side2 && side1 != side3) { System.out.println("The triangle is Scalene with all no equal side."); System.out.println("Scalene triangles have no equal angles."); } } } } //WRITE THE AREA METHOD WITH TWO DOUBLE PARAMETERS WITH A VOID RETURN TYPE { // CALCULATE THE AREA (this.area) System.out.println(this.area); } //WRITE THE PERMIMETER METHOD WITH THREE DOUBLE PARAMETERS WITH A VOID RETURN TYPE { // CALCULATE THE PERMIMETER (this.permimeter) System.out.println(this.permimeter); } public static void main(String[] args) { System.out.println("Welcome to the triangles program"); Scanner key = new Scanner(System.in); int x = 1; String y = "y"; //CREATE A TRIANGLES OBJECT CALLED triangleObj do { try { while ("y".equalsIgnoreCase(y)) { x = 1; System.out.print("Enter length 1: "); double side1 = key.nextDouble(); System.out.print("Enter length 2: "); double side2 = key.nextDouble(); System.out.print("Enter length 3: "); double side3 = key.nextDouble(); System.out.println(" "); System.out.print("Enter the Base: "); double base = key.nextDouble(); System.out.print("Enter the Height: "); double height = key.nextDouble(); System.out.println(" "); System.out.println("---------------------"); System.out.println(" "); triangleObj.triangleType(side1, side2, side3); triangleObj.area(base, height); triangleObj.permimeter(side1, side2, side3); x = 2; System.out.print("Continue? Y/N: "); y = key.nextLine(); y = key.nextLine(); } } //CREATE THE CATCH CLAUSE WITH Exception e { System.out.println("Please enter a valid number."); key.next(); } } while (x == 1); } }
**********
Thank you :)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
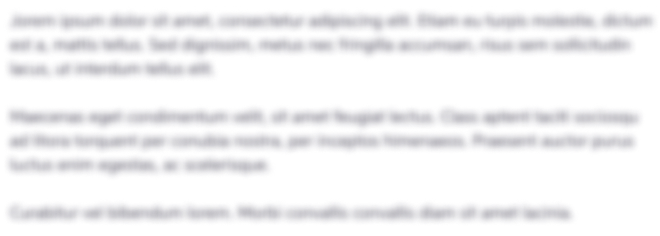
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started