Question
Write a program for playing a variation of the Subtraction game called the game of NIM. Our version of the game starts with up to
Write a program for playing a variation of the Subtraction game called the game of NIM. Our version of the game starts with up to 15 rods and up to 10 objects in each rod. Two players take turns removing objects from the rods. On a players turn, she chooses a rod and removes one or more objects from that rod. She can remove all objects from the rod. The player who takes the last object from the rods (so that all rods are empty) wins. Run the solution subtract_solution.exe to play the game. Your program must produce exactly the same output as this solution. DO NOT delete the code already given to you in the code template. You must use the code given to you in the template in your solution. The algorithm for the game is provided below. Most steps in the algorithm should be implemented as function calls. You need to implement the algorithm and the functions as described below. You may implement additional functions to help you in writing your solution. Though, in order to receive full credit you must follow the instructions in this assignment and de?ne these functions exactly as indicated. You will implement the following algorithm in the main function. Each line in the algorithm will correspond to a function call. Important! Write your code incrementally. This means you should write each function one at a time, check that it compiles and runs properly, test it, and then implement the next function. i. Prompt and read the number of rods ii. Prompt and read the number of objects in each rod; iii. Draw the rods with percentages; iv. Display statistics; v. while (some rod is NOT empty) do vi. Prompt and read the next players move (Prompt and read the rod to modify and the number of objects to remove from the rod); vii. Remove the speci?ed number of objects from the speci?ed rod; viii. if (all rods are empty) then ix. Print a message congratulating the winning player. x. else xi. Redraw the rods with percentages; xii. Display statistics; xiii. Change to the other player; xiv. end xv. end 1. All functions should be written AFTER the main procedure. 2. All function prototypes should be written for each function and placed BEFORE the main procedure. 3. Each function should have a comment explaining what it does. 4. Each function parameter should have a comment explaining the parameter. 5. Your program MUST NOT use any global variables nor any global constants. 6. In addition to implementing the above algorithm in the main function, you must de?ne the following constants and variables in the main function: - De?ne two constants to hold the maximum number rods (15) and the maximum number of objects per rod (10). - The list of rods will be stored in an array of integers, where each integer represents the number of objects in that rod. De?ne a pointer variable for this array (see the lecture notes on Arrays and Pointers). Also de?ne an integer to hold the size of this array. - De?ne a variable that represents the current player (1 or 2). 7. Important: PLEASE READ! The following description provides speci?cations for the functions you will write that includes input parameters and return values. You are responsible for implementing these functions as described in these speci?cations in order to receive full credit. These functions may call additional helper functions that you de?ne. You must determine good function names as well as good variable names for your function parameters and local variables. You must correctly identify whether a function parameter should be passed by value or passed by reference. In addition you must correctly determine whether a function parameter should be declared with the const speci?er. Remember, const is used to indicate that the function parameter is not modi?ed in the function (see the lecture notes). It may be applied to a parameter that is either passed by value or passed by reference. It allows code developers to better undertand your code and ensure that they (and you) adhere to your speci?cations if they want to modify the code. 8. Write a function to implement step (i) of the algorithm. De?ne the function to have one input parameter that holds the maximum number of rods (see the constant de?ned above) and return an integer. Prompt the user for the number of rods used in the game. If the number entered is not between 1 and the maximum number of rods then display a warning message and prompt again. This is called input validation on the users input. 9. In the main function, allocate an array in the declared array pointer representing the list of rods (see description above) to have the same size as the number of rods returned from the function in step (i). 10. Write a procedure (this is a function that does not return a value and so is declared with a return type of void) that implements step (ii) of the algorithm. De?ne the procedure to have three input parameters: the array of rods, the size of the array, and the maximum number of objects per rod (see the constant de?ned above). This procedure will traverse the array of rods and call the following helper procedure for each rod in the list. - Write a helper procedure that will prompt the user for the number of objects to place on this rod. If the number of objects entered for the rod is not between 1 and the maximum number of objects allowed then display a warning message and prompt again. De?ne this helper procedure to have three input parameters: the array entry for this rod (use pass by reference), the array index for this rod, and the maximum number of objects per rod. Important: Again, a procedure is a function that has no return value. You must de?ne these functions as procedures by de?ning their return type as void. 11. Write a procedure to draw the rods to implement steps (iii) and (xi) of the algorithm. Draw each rod on its own row. In the row for rod i, there is a label Rod i: followed by n #s where n is the number of objects in rod i. The procedure should output an empty line before and after the ?rst and last rows, respectively. For instance if rod 0 has 3 objects, rod 1 has zero objects, and rod 2 has 8 objects, the procedure (and helper procedures) should output: Rod 0: ### (27.273%) Rod 1: (0.000%) Rod 2: ######## (72.727%) De?ne the procedure to have two input parameters: the array of rods and the size of the array. First, calculate the total number of objects in the array of rods. Next, traverse the array of rods and call a helper procedure to display each complete row. - Write the helper procedure to have three input parameters: the array index for this rod, the number of objects for this rod, and the total number of objects in the array of rods. The percentage displayed at the end of each row is the fraction of objects on this rod given the total number of objects in the array of rods (you just computed). Format each row using setw in iomanip and avoid using hard coded spaces. When there are ten or more rods to display, your output must align the labels Rod ?: and Rod ??: appropriately, where ? is a digit. Use setw to format the text between Rod and the :. Using setw, format the #s in a column of size ten that is left justi?ed (look up the left speci?er under iomanip). There needs to be ?ve spaces between the column of #s and the percentage. Do NOT hard code these ?ve spaces. Instead, use setw to format the last column for the percentage. Look up how to use the right speci?er in iomanip to help you solve this problem. Also, format the percentage to show three digits after the decimal place. Decide if additional helper functions/procedures would be useful here. 12. Write a procedure to display the statistics for the list of rods that will be called in steps (iv) and (xii) of the algorithm. The statistics are 1) The rods with the smallest number of objects, 2) The rods with the largest number of objects, and 3) The average number of objects per rod taking into account only rods with objects. De?ne the procedure to have two input parameters: the array of rods and the size of the array. This procedure will call three helper procedures. - Write a helper procedure to display the rods with the smallest number of objects. This procedure will have two input parameters: the array of rods and the size of the array. Note that there may be more than one rod with the smallest number of objects. - Write a helper procedure to display the rods with the largest number of objects. This procedure will have two input parameters: the array of rods and the size of the array. Note that there may be more than one rod with the largest number of objects. - Write a helper procedure to display the average number of objects per rod, but only taking into accout rods with at least one object. The average must be formatted to display two digits after the decimal place. 13. Write a function which returns true (boolean) if all the rods have zero objects and returns false otherwise. Call this function to implement steps (v) and (viii) of the algorithm. Note that the same function will be used for both steps. 14. Write a procedure to implement step (vi) of the algorithm where the current player makes her next move. De?ne the procedure to have ?ve input parameters: the array of rods, the size of the array, player id, which rod chosen by the player on this turn, and how many objects the player would like to remove from this rod. The player id is either the integer 1 or 2 to indicate which player is taking a turn. This procedure will perform two steps with the help of helper functions/procedures as speci?ed below: 1) Using the following helper functions and procedures, prompt and read for the rod from which to remove objects. If the player inputs an invalid rod id or chooses a rod with no objects then display a warning message and prompt again. These three helper functions/procedures will be called from this procedure. In addition, the last two helper procedures will call the ?rst helper function. - First, write a helper function to have one input parameter, the player id. Prompt the player to choose a rod (rod id) and then return this value. Thus, this helper function has a return type that is NOT void. Do not perform any input validation in this function. - Next, write a second helper procedure to have three input parameters: the player id, the rod selected by this player, and the total number of rods (i.e., the size of the array of rods). This procedure will validate the rod selected by this player, i.e. the rod selected must be between 0 and n - 1 where n is the total number of rods. If the rod selected is invalid then the procedure displays a warning message and prompts for rod selection again by calling the ?rst helper function. - Finally, write a third helper procedure to have three input parameters: the array of rods, the player id, and the rod selected by this player. This procedure will validate whether the rod selected has at least one object. If the rod selected is invalid then the procedure displays a warning message and prompts for rod selection again by calling the ?rst helper function. 2) Using the following helper function, prompt and read how many objects to remove from the chosen rod. If the player inputs an invalid number of objects then display a warning message and prompt again. The number of objects to remove must be positive and must not exceed the number of objects on the chosen rod. The following helper function will be called from this procedure and will perform the following task described below. This procedure will check if the returned value from the helper function is valid and prompt the user again if necessary. - Write a helper function to have two input parameters: the number of objects on the chosen rod and the rod id. The function will prompt the player for the number of objects to remove from the indicated rod and returns this value. Note this helper function will NOT perform any input validation checks. Instead the calling procedure will perform validation on the returned value from this helper function. 15. Write a procedure to implement step (vii) of the algorithm. De?ne the procedure to have three input parameters: the array of rods, the rod id of the chosen rod, and the number of objects to remove. This function will modify the array of rods by subtracting the speci?ed number of objects from the chosen rod. 16. Write a procedure to implement step (ix) of the algorithm. De?ne the procedure to have a single input parameter that holds the player id. The procedure will print a message congratulating this winning player. The message should identify who won (player 1 or player 2). 17. Write a procedure to implement step (xiii) of the algorithm. De?ne this procedure to have a single input parameter that holds the player id. This procedure will switch the turn to the other player. In other words, if the player indicated in the input parameter is 1, then the procedure should change this value to 2. If the player indicated in the input parameter is 2, then the procedure should change this value to 1. 18. Be sure to modify the header comments File, Created by, Creation Date, and Synopsis at the top of the ?le. Each synopsis should contain a brief description of what the program does. 19. Be sure that there is a comment documenting each variable. 20. Be sure that your if statements, for and while loops and blocks are properly indented. 21. Check your output against the output from the solution executable provided. 22. Test your code thorougly!
?THE FOLLOWING IS A SAMPLE OUTPUT OF HOW THE GAME SHOULD WORK:
How many rods are in this game? 16 Number of rods must be positive and less than or equal to 15
Enter number of rods again : 3
How many objects are on rod 0: 11 Sorry, the number of objects must be positive and less than or equal to 10. How many objects are on rod 0: 3 How many objects are on rod 1: 4 How many objects are on rod 2: 5
Rod 0: ### (25.000%) Rod 1: #### (33.333%) Rod 2: ##### (41.667%)
Rod 0 has the smallest number of objects with 3 object(s). Rod 2 has the largest number of objects with 5 object(s). The average number of objects per rod (i.e., rods with objects) is 4.00 objects.
Player (1) : Which rod would you like to play? 3 Invalid rod number. Please try again.
Player (1) : Which rod would you like to play? 0 Enter number of objects to remove (3 or less) from rod 0: 4 Can only remove up to 3 object(s). Please try again. Enter number of objects to remove (3 or less) from rod 0: 3
Rod 0: (0.000%) Rod 1: #### (44.444%) Rod 2: ##### (55.556%)
Rod 1 has the smallest number of objects with 4 object(s). Rod 2 has the largest number of objects with 5 object(s). The average number of objects per rod (i.e., rods with objects) is 4.50 objects.
Player (2) : Which rod would you like to play? 1 Enter number of objects to remove (4 or less) from rod 1: 4
Rod 0: (0.000%) Rod 1: (0.000%) Rod 2: ##### (100.000%)
Rod 2 has the smallest number of objects with 5 object(s). Rod 2 has the largest number of objects with 5 object(s). The average number of objects per rod (i.e., rods with objects) is 5.00 objects.
Player (1) : Which rod would you like to play? 2 Enter number of objects to remove (5 or less) from rod 2: 5
Congratulations! Player 1 wins.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
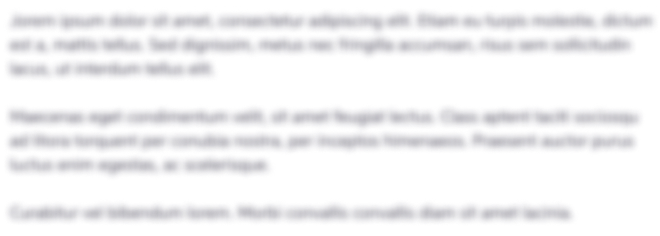
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started