Question
Write a program in a class FlowerCounter that computes the cost of flowers sold at a flower stand. There are 5 kinds of flowers, petunia,
Write a program in a class FlowerCounter that computes the cost of flowers sold at a flower stand.
There are 5 kinds of flowers, petunia, pansy, rose, violet, and carnation, which cost, respectively, 50, 75, $1.50, 50, and 80 per flower.
Create an array of Strings that holds the names of these flowers.\ Create another array that holds the cost of each corresponding flower.
Hints: you can use the Java array initializer syntax to create these; make sure the indexes of each flower's name and cost are the same.
Your program should read the name of a flower using Scanner's next() and the quantity (number of flowers) desired by a customer using nextInt() in a loop; when the user types quit, exit the loop.
Locate the flower in the name array and use that same index to obtain the cost per flower from the costarray. At the end, print the number of flowers bought and the total cost of the sale (you have to accumulate this information in the loop).
Hints: use the Scanner next() method to read in the name of the flower; if the user did not type quit in lower or upper case, use equalsIgnoreCase() in a loop to compare their flower's name against those in the array. Be sure to deal with the case where the user wants to buy a flower whose name doesn't exist in thename array, and if so print a message saying the shop does not sell that kind of flower.
IN JAVA:
public class FlowerCounter
{
// Compute the cost of flowers sold at a flower stand
public static void main(String[] args)
{
// create String array name and double array cost to hold the names
// and costs of each flower as above; make sure both arrays are the
// same length, and that the costs line up with the flower names;
// you can use the Java array initializer syntax to do this easily
/* your code goes below this comment */
Scanner170 keyboard = new Scanner170(System.in); // to read the user's requests
double totalSale = 0.0;
int numberBought = 0;
System.out.println("Welcome to our flower shop.");
System.out.println("Here are the flowers we have for sale, and their prices:");
System.out.println("Name\t\tPrice");
// in a for loop, print out the names and costs of each flower,
// separated by a tab character
/* your code to do this goes below this comment */
System.out.println();
// now write a while loop that continues until the user types "quit"
// (without the quotes) as the name of the flower they want to buy,
// then end the loop and the program; note: ignore upper and lower case
// for every other flower name, look in the names array to see if we
// sell that flower; if not, print "Sorry, we do not sell that flower."
// and continue the loop; note: ignore upper and lower case
// if the flower is in the names array, ask the user how many they want
// to purchase, and add the cost of that sale to the total cost so far
// then continue the loop
// once the loop ends, print out this message based on the user's flowers:
// Number of flowers bought:
/* your code to do this goes below this comment */
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
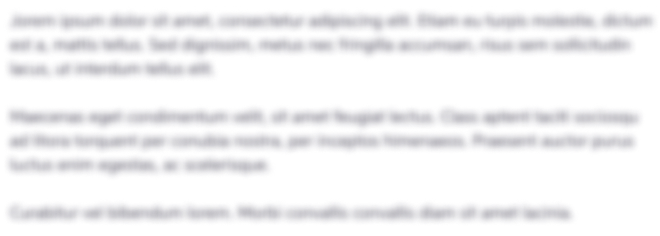
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started