Question
Write a program in C : (Arrays of Pointers to Functions) Rewrite the program of Fig. 6.22 to use a menu-driven interface. The program should
Write a program in C :
(Arrays of Pointers to Functions) Rewrite the program of Fig. 6.22 to use a menu-driven
interface. The program should offer the user four options as follows:
One restriction on using arrays of pointers to functions is that all the pointers must have the same
type. The pointers must be to functions of the same return type that receive arguments of the same
type. For this reason, the functions in Fig. 6.22 must be modified so that they each return the same
type and take the same parameters. Modify functions minimum and maximum to print the minimum
or maximum value and return nothing. For option 3, modify function average of Fig. 6.22 to output
the average for each student (not a specific student). Function average should return nothing
and take the same parameters as printArray, minimum and maximum. Store the pointers to the four
functions in array processGrades and use the choice made by the user as the subscript into the
array for calling each function.
Fig. 6.22: fig06_22.c // Two-dimensional array manipulations. #include
// function prototypes int minimum(const int grades[][EXAMS], size_t pupils, size_t tests); int maximum(const int grades[][EXAMS], size_t pupils, size_t tests); double average(const int setOfGrades[], size_t tests); void printArray(const int grades[][EXAMS], size_t pupils, size_t tests);
// function main begins program execution int main(void) { // initialize student grades for three students (rows) int studentGrades[STUDENTS][EXAMS] = { { 77, 68, 86, 73 }, { 96, 87, 89, 78 }, { 70, 90, 86, 81 } };
// output array studentGrades puts("The array is:"); printArray(studentGrades, STUDENTS, EXAMS);
// determine smallest and largest grade values printf(" Lowest grade: %d Highest grade: %d ", minimum(studentGrades, STUDENTS, EXAMS), maximum(studentGrades, STUDENTS, EXAMS));
// calculate average grade for each student for (size_t student = 0; student < STUDENTS; ++student) { printf("The average grade for student %u is %.2f ", student, average(studentGrades[student], EXAMS)); } }
// Find the minimum grade int minimum(const int grades[][EXAMS], size_t pupils, size_t tests) { int lowGrade = 100; // initialize to highest possible grade
// loop through rows of grades for (size_t i = 0; i < pupils; ++i) {
// loop through columns of grades for (size_t j = 0; j < tests; ++j) {
if (grades[i][j] < lowGrade) { lowGrade = grades[i][j]; } } }
return lowGrade; // return minimum grade }
// Find the maximum grade int maximum(const int grades[][EXAMS], size_t pupils, size_t tests) { int highGrade = 0; // initialize to lowest possible grade
// loop through rows of grades for (size_t i = 0; i < pupils; ++i) {
// loop through columns of grades for (size_t j = 0; j < tests; ++j) {
if (grades[i][j] > highGrade) { highGrade = grades[i][j]; } } }
return highGrade; // return maximum grade }
// Determine the average grade for a particular student double average(const int setOfGrades[], size_t tests) { int total = 0; // sum of test grades // total all grades for one student for (size_t i = 0; i < tests; ++i) { total += setOfGrades[i]; } return (double) total / tests; // average }
// Print the array void printArray(const int grades[][EXAMS], size_t pupils, size_t tests) { // output column heads printf("%s", " [0] [1] [2] [3]");
// output grades in tabular format for (size_t i = 0; i < pupils; ++i) {
// output label for row printf(" studentGrades[%u] ", i);
// output grades for one student for (size_t j = 0; j < tests; ++j) { printf("%-5d", grades[i][j]); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
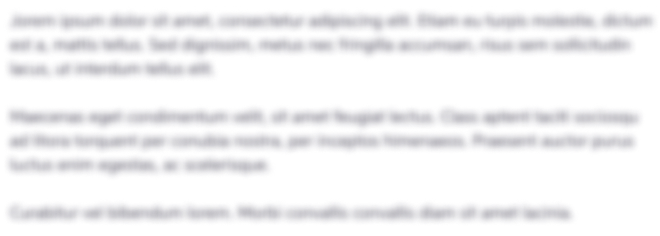
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started