Question
Write a program that displays a menu of choices, like this: a) Draw a rectangle b) Draw a triangle c) Greatest Common Divisor d) Reverse
Write a program that displays a menu of choices, like this: a) Draw a rectangle b) Draw a triangle c) Greatest Common Divisor d) Reverse Digits e) Exit program Enter your choice: If the user inputs a then ask the user to input the height and width of a rectangle as follows: Enter rectangle height and width [1 to 30]: 4 7 The program should display a rectangle filled with * as shown below: ******* ******* ******* ******* If the user inputs b then ask the user to input the height of a triangle as follows: Enter triangle height [1 to 30]: 3 The program should display an isosceles triangle filled with * as shown below: * *** ***** If the user inputs c then ask the user to input two integer numbers: Enter two integer numbers: 18 12 The program should compute and print the greatest common divisor as follows: The greatest common divisor of 18 and 12 is 6 If the user inputs d then ask the user to input an integer number: Enter an integer number: 7253 The program should compute and print the number with reverse digits as follows: The reverse of the integer number 7253 is 3527 Page 2 of 2 The program should display repeatedly the same menu and ask the user to re-enter a new choice. If the user enters a character other than a to e then print an error message and redisplay the menu again. If the user enters e then terminate the execution of the program. In addition, all user input should be checked. This includes the height and width of a rectangle, and the height of a triangle. Display an error message if the user input is outside the range [1 to 30]. You may also detect an input that is not an integer (such as a letter), and display an error message accordingly. The greatest common division gcd of two integers x and y is defined as follows: if y == 0 then gcd(x, 0) = x; else gcd(x, y) = gcd(y, x%y); The gcd can be calculated repeatedly in a loop until x%y becomes 0. For example, gcd(18, 12) gcd(12, 18%12) gcd(12, 6) gcd(6, 12%6) gcd(6, 0) 6 To reverse the digits of an integer, you should extract the digits and then reverse them in a loop. For example, 7253%10 extracts the digit 3. However, 7253/10 (equal to 725) removes the digit 3. Your program should define five functions, in addition to the main function. These are: char menu(); // display menu and return choice void draw_rectangle(int h, int w); // draw rectangle void draw_triangle(int h); // draw triangle int gcd(int x, int y); // calculate and return gcd of x and y int reverse_digits(int x); // calculate and return the reverse of x
Step by Step Solution
There are 3 Steps involved in it
Step: 1
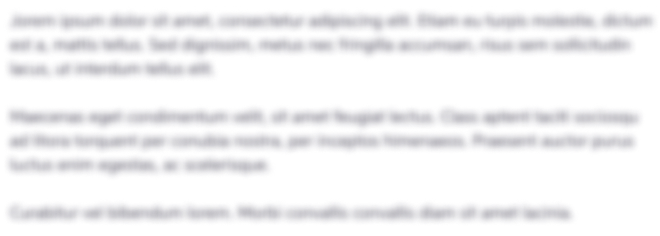
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started