Question
Write a program to play a song represented as text. In Eclipse, create a package called program2 (note the lowercase first letter), in which you
Write a program to play a song represented as text. In Eclipse, create a package called program2 (note the lowercase first letter), in which you will create a program called PlaySimpleSong.
Write the program so that it:
declares and creates a symbol table using the algs31.BinarySearchST class;
reads in a file notes_frequencies.txt where each line is a pair of strings separated by whitespace. The first string is the name of a musical note and the second its sound frequency as found on a piano. For example, the note A4 is paired with the frequency 440Hz and the note C4 with the frequency 261.626Hz. As each line is read, an entry is made in the symbol table where the note name is the key and the frequency is the value.
reads in a song file, where each line contains a note name and a duration in seconds, separated by whitespace. A sample file is sample_simple_song.txt, which plays every C for half a second. Another song you might try is lotr.txt. Looking up the frequency corresponding to the note name, the program calls the method below to play the note.
Both the notes and frequencies file and the song file should be placed into the Eclipse data directory and, as in the GPA program, read in using StdIn and the fromFilemethod.
To process a text file where each line contains a fixed set of data fields:
use the method readLine in the StdIn class, which returns a string;
split the string into an array of strings using the instance method split in the String class;
convert the numeric strings into numeric values using the method parseDouble in the Double class.
To play each note, place into your program and call this method:
public static void playTone(double frequency, double duration) { final int sliceCount = (int) (StdAudio.SAMPLE_RATE * duration); final double[] slices = new double[sliceCount+1]; for (int i = 0; i <= sliceCount; i++) { slices[i] = Math.sin(2 * Math.PI * i * frequency / StdAudio.SAMPLE_RATE); } StdAudio.play(slices); }
You will also have to import stdlib.StdAudio: http://introcs.cs.princeton.edu/java/stdlib/javadoc/StdAudio.html
sample_simple_song.txt
C1 0.5 C2 0.5 C3 0.5 C4 0.5 C5 0.5 C6 0.5 C7 0.5 C8 0.5
notes_frequencies.txt
A0 27.5 A#0 29.1353 B0 30.8677 C1 32.7032 C#1 34.6479 D1 36.7081 D#1 38.8909 E1 41.2035 F1 43.6536 F#1 46.2493 G1 48.9995 G#1 51.913A1 55 A#1 58.2705 B1 61.7354 C2 65.4064 C#2 69.2957 D2 73.4162 D#2 77.7817 E2 82.4069 F2 87.3071 F#2 92.4986 G2 97.9989 G#2 103.826 A2 110 A#2 116.541 B2 123.471 C3 130.813 C#3 138.591 D3 146.832 D#3 155.563 E3 164.814 F3 174.614 F#3 184.997 G3 195.998 G#3 207.652 A3 220 A#3 233.082 B3 246.942 C4 261.626 C#4 277.183 D4 293.665 D#4 311.127 E4 329.628 F4 349.228 F#4 369.994 G4 391.995 G#4 415.305 A4 440 A#4 466.164 B4 493.883 C5 523.251 C#5 554.365 D5 587.33 D#5 622.254 E5 659.255 F5 698.456 F#5 739.989 G5 783.991 G#5 830.609 A5 880 A#5 932.328 B5 987.767 C6 1046.5 C#6 1108.73 D6 1174.66 D#6 1244.51 E6 1318.51 F6 1396.91 F#6 1479.98 G6 1567.98 G#6 1661.22 A6 1760 A#6 1864.66 B6 1975.53 C7 2093 C#7 2217.46 D7 2349.32 D#7 2489.02 E7 2637.02 F7 2793.83 F#7 2959.96 G7 3135.96 G#7 3322.44 A7 3520 A#7 3729.31 B7 3951.07 C8 4186.01
Step by Step Solution
There are 3 Steps involved in it
Step: 1
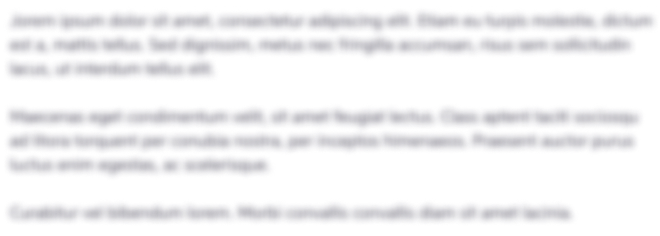
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started