Question
Write a program which will enter information relating to a speeding violation and then compute the amount of the speeding ticket. The program will need
Write a program which will enter information relating to a speeding violation and then compute the amount of the speeding ticket. The program will need to enter the posted speed limit, actual speed the car was going, and whether or not the car was in a school zone and the date of the violation. Additionally, you will collect information about the driver (see output for specifics).
The way speeding tickets are computed differs from city to city.
For this assignment, we will use these rules, which need to be applied in this order:
- All tickets will start with a minimum amount of $75.
- An additional $6 is charged for every mph over the posted speed limit.
- If you are traveling over 30mph over the posted speed limit, an additional $160 is charged.
- If the speeding occurred in a school zone, the cost of the ticket is doubled.
You will then print the ticket on the output screen.
This program is not concerned with checking for proper input. For example, if the posted speed limit is 55mph, and you were traveling 50mph, then a ticket would not be necessary in the first place. For this program, you can assume that the data will be entered correctly.
TicketRunner Student Version | Do not copy this file, which is provided. The ticket.java file is also provided. |
// The Speeding Ticket Program // //Name - //Date - //Class - //Lab -
import java.util.Scanner; import java.lang.System.*
public class TicketRunner { public static void main(String args[]) { System.out.println("The Speeding Ticket Program"); System.out.println(); System.out.println("By: John Smith"); // Substitute your own name here. System.out.println(" ============================== ");
} } |
Output #1
----jGRASP exec: java Lab07Av100 The Speeding Ticket Program By: John Smith ============================== Name of Driver? --> Johnny Viking
Drivers License Number? --> 02453756
Address? --> 234 Dulles
City? --> Sugar Land
State? --> TX
Zip? --> 77044
Did the violation occur in a school zone? {Y/N} --> N
Date of Violation: Month (number)? --> 02 Day? --> 14 Year? --> 21
What is the posted speed limit? --> 40 How fast was the car travelling in mph? --> 55 02/14/21
Johnny Viking (TDL: 02453756) 234 Dulles Sugar Land, TX 77044 Dear Citizen Viking,
You have received this citation for driving 55 mph in an area with a posted speed limit of 40.
This violation did not occur in a school zone.
Your fine is $165.00 and can be paid at the address below.
Please remember to buckle up and drive safely.
----jGRASP: operation complete.
|
Output #2
----jGRASP exec: java Lab07Av100
The Speeding Ticket Program By: John Smith ============================== Name of Driver? --> Cindy Knight
TDL? --> 23456835
Address? --> 2145 Elkins
City? --> Sugar Land
State? --> TX
Zip? --> 72345
Did the violation occur in a school zone? {Y/N} --> Y
Date of Violation: Month (number)? --> 01 Day? --> 28 Year? --> 21
What is the posted speed limit? --> 40 How fast was the car travelling in mph? --> 80
01/28/21
Cindy Knight(TDL: 23456835) 2145 Elkins Sugar Land, TX 72345 Dear Citizen Knight,
You have received this citation for driving 80 mph in an area with a posted speed limit of 40.
This violation occurred in a school zone.
Your fine is $950 and can be paid at the address below.
Please remember to buckle up and drive safely.
|
Ticket.java file 9 public class Ticket 10 { 11 //Driver Information 12 private String name, TDL, address, city, state, zip; 13 14 //violation Information 15 private int postedSpeed, travelling, ticketAmount, day, month, year; 16 private boolean schoolzone; 17 18 public Ticket() 19 { 20 } 21 22 public Ticket (String nm, String tdi, string addr, String sty, String stor string zp, int pspd, int travel, int d, int m, int vf, String school) 23 { 24 } 25 public void calcTicket() 27 { 28 } 29 30 public String lastName() 31 { 32 return ""; 33 } 34 35 public String tostring() 36 { 37 return""; 38 } 26 39 } | //Ticket Runner import java.util.Scanner; import java.lang.System.*; public class TicketRunner { public static void main(String args[]) { Scanner keyboard = new Scanner (System.in); System.out.println("The Speeding Ticket Program"); System.out.println(); System.out.println("By: John Smith"); // Substitute your own name here. System.out.println(" = "); // add code for input here // Instantiate and print System.out.println(); MAA } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
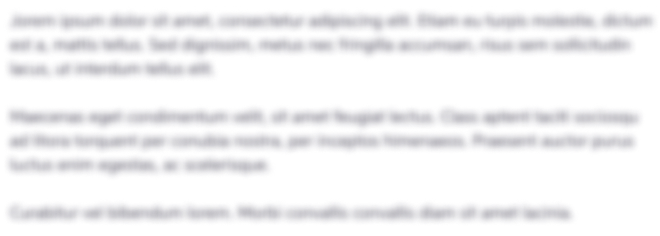
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started