Question
Write a Python program, with the given Python files. o Enhancing our Queue: Enhance and implement a Queue ADT (with Linked-List approach), by modifying our
Write a Python program, with the given Python files.
o Enhancing our Queue: Enhance and implement a Queue ADT (with Linked-List approach), by modifying our given Python file A2B.py (based on the one in our lecture notes, QueueLL.py)
o Extra Operations (methods of the class) to be implemented by Students:
At least one line of simple comment for each extra operation required
Operation (QueueLL) | Description |
isEmptyQ():bool | Check if the queue is empty or not |
sizeQ():int | Get the size of the queue (total number of elements) |
searchQ(elt):bool | Check if an input searching element elt is in the queue |
dispDupEltQ(): | Display duplicated items only once, as the sample display output. * You may use extra queues in this method to finish this task |
Given Materials:
o Python file A2B.py, to be modified and completed by student. Also modify top comments for your STUDENT INFO. (DO NOT modify this given portions, including given methods if any)
o Python file A2BT.py for basic running and testing.
DO NOT modify this given test file, except the STUDENT INFO part.
File A2B.py, to be modified and completed by student.
# A2B.py, for IDSA Assign 2
# ...
class QueueLL: # defining a class of Queue, with Linked-List
# ...
###################### STUDNET's WORK ######################
# simple comment HERE
def isEmptyQ(self):
pass # TO BE DONE
# MORE TO BE DONE
#######################################
--------------------------------------------------------------------------------------------------------------------------------
File A2BT.py for basic running and testing
# A2BT.py, for basic running and testing.
# * DO NOT modify this given test file, except the STUDENT INFO part.
# Main Testing Program
from A2B import QueueLL
def main():
print(" === A2B, Enhanced QueueLL program, by === ")
myQ = QueueLL()
print(" --- 1. New QueueLL created ---")
myQ.displayQ()
print(f" isEmptyQ() is: {myQ.isEmptyQ()}")
myQ.enqueue('AA'); myQ.enqueue('BB'); myQ.enqueue('CC')
myQ.enqueue('AA'); myQ.enqueue('BB'); myQ.enqueue('AA')
print(" --- 2. ENQueued: AA,BB,CC,AA,BB,AA ---")
myQ.displayQ()
print(f" isEmptyQ() is: {myQ.isEmptyQ()}")
print(f" sizeQ() is: {myQ.sizeQ()}")
print(f" searchQ('CC') is: {myQ.searchQ('CC')}")
print(f" searchQ('DD') is: {myQ.searchQ('DD')}")
print(" --- 3. dispDupEltQ() ---")
myQ.dispDupEltQ()
print(" === Program ends === ")
main()
----------------------------------------------------------------------------------------------------------------------
Sample console display output of executing the main testing program A2BT.py
=== A2B, Enhanced QueueLL program, by ===
--- 1. New QueueLL created ---
>>> Queue Display, (Front/Left to Rear/Right)
... AN EMPTY QUEUE
isEmptyQ() is: True
--- 2. ENQueued: AA,BB,CC,AA,BB,AA ---
>>> Queue Display, (Front/Left to Rear/Right)
... FRONT , REAR :
< AA < BB < CC < AA < BB < AA
isEmptyQ() is: False
False sizeQ() is: 6
searchQ('CC') is: True
searchQ('DD') is: False
--- 3. dispDupEltQ() ---
--- DUPLICATED ITEMS in QUEUE are:
AA BB
=== Program ends ===
-------------------------------------------------------------------------------------------------------------------------------
A2B.py
self.nextN = inNext # the next node, default None class QueueLL: # defining a class of Queue, with Linked-List def __init__(self): # constructor self.headN = None # the head Node (FRONT of Queue) self.tailN = None # the tail Node (REAR of Queue)
###################### STUDNET's WORK ###################### # simple comment HERE def isEmptyQ(self): pass # TO BE DONE # simple comment HERE def sizeQ(self): pass # TO BE DONE # simple comment HERE def searchQ(self, elt): pass # TO BE DONE # simple comment HERE def dispDupEltQ(self): # display duplicated items, only once pass # TO BE DONE ####################################### def displayQ(self): # (Front/Left to Rear/Right) print(f">>> Queue Display, (Front/Left to Rear/Right)") if self.headN==None: print(" ... AN EMPTY QUEUE"); return else: print(f" ... FRONT <{self.headN.value}>," f" REAR <{self.tailN.value}>:") curN = self.headN while curN!= None: print(f" < {curN.value}", end='') curN = curN.nextN print()
def peek(self): if self.headN==None: # case of empty Queue print("
def enqueue(self, inValue): # method, insert to tail / rear newN = Node(inValue, None) # create a new node for enqueue if self.headN==None: # case empty Queue, a new HEAD & TAIL self.headN = newN self.tailN = self.headN else: # insert as a new TAIL / REAR self.tailN.nextN = newN self.tailN = newN
def dequeue(self): # method, remove and return front elt if self.headN==None: # case of empty Queue print("
---------------------------------------------------------------------------------------------------------------------------------
A2BT.py
# A2BT.py, for basic running and testing. # * DO NOT modify this given test file, except the STUDENT INFO part. # Main Testing Program
from A2B import QueueLL
def main(): print(" === A2B, Enhanced QueueLL program, by
myQ.enqueue('AA'); myQ.enqueue('BB'); myQ.enqueue('CC') myQ.enqueue('AA'); myQ.enqueue('BB'); myQ.enqueue('AA') print(" --- 2. ENQueued: AA,BB,CC,AA,BB,AA ---") myQ.displayQ()
print(f"
print(" --- 3. dispDupEltQ() ---") myQ.dispDupEltQ() print(" === Program ends === ") main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
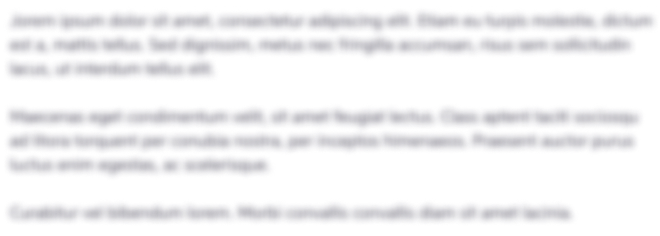
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started