Question
Write a visual display of Selection Sort and Insertion Sort. The GUI steps through the two sorting algorithms. Program Description Your program should display bars
Write a visual display of Selection Sort and Insertion Sort. The GUI "steps through" the two sorting algorithms.
Program Description
Your program should display bars of various heights, corresponding to random values stored in an array.
The selection and insertion sort arrays should initially contain the same numbers.
A button allows the user to step through a single pass of each sorting algorithm.
When the sort is complete, the button is disabled.
Notes
import javafx.application.*;
import javafx.event.*;
import javafx.geometry.*;
import javafx.scene.*;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.scene.paint.*;
import javafx.scene.shape.*;
import javafx.scene.text.*;
import javafx.stage.*;
public class SortGUI extends Application {
private Button sortButton;
private Pane selectionSortPane, insertionSortPane;
// variables for the display
private static final int ARRAY_SIZE = 50, BAR_WIDTH = 8, SPACE_APART = 5;
private static final int SSORT_Y_START = 120, ISORT_Y_START = 170;
private static final int MAX = 80, MIN = 1;
private int[] selectionArray, insertionArray;
public void start(Stage primaryStage) {
sortButton = new Button("Click to take one step in the sort");
sortButton.setOnAction(this::handleButton);
HBox buttonBox = new HBox(sortButton);
buttonBox.setAlignment(Pos.CENTER);
selectionArray = new int[ARRAY_SIZE];
insertionArray = new int[ARRAY_SIZE];
// ??? YOUR CODE HERE- FILL THE ARRAYS WITH RANDOM NUMBERS USING MIN AND MAX VARIABLES (DECLARED ABOVE)
// PUT THE SAME NUMBERS IN BOTH ARRAYS
// IF COMPLETING EXTRA CREDIT, ENSURE THAT THERE ARE NO DUPLICATE VALUES WITHIN EACH ARRAY
selectionSortPane = new Pane();
setupSortPane(selectionSortPane, SSORT_Y_START, selectionArray, Color.BLUE);
insertionSortPane = new Pane();
setupSortPane(insertionSortPane, ISORT_Y_START, insertionArray, Color.RED);
Text selectionText = new Text(" Selection Sort");
selectionText.setFont(Font.font(20));
Text insertionText = new Text(" Insertion Sort");
insertionText.setFont(Font.font(20));
VBox primaryBox = new VBox(buttonBox, selectionSortPane, selectionText, insertionSortPane, insertionText);
primaryBox.setStyle("-fx-background-color: white");
Scene scene = new Scene(primaryBox, 700, 400, Color.TRANSPARENT);
primaryStage.setScene(scene);
primaryStage.setResizable(false);
primaryStage.setTitle("Sorting GUI");
primaryStage.show();
}
private void setupSortPane(Pane pane, int startingY, int[] array, Color color) {
pane.getChildren().clear();
int x = 20;
for (int index = 0; index
Rectangle rect = new Rectangle(x, startingY - array[index], BAR_WIDTH, array[index]);
x = x + BAR_WIDTH + SPACE_APART; // Adds width and spaces so bars don't overlap
rect.setFill(color);
pane.getChildren().add(rect);
}
}
private void modifiedSelectionSort() {
// ??? YOUR CODE HERE- THIS SHOULD BE ONE SINGLE STEP OF THE SELECTION SORT
}
private void modifiedInsertionSort() {
// ??? YOUR CODE HERE- THIS SHOULD BE ONE SINGLE STEP OF THE INSERTION SORT
}
private void handleButton(ActionEvent event) {
// ??? YOUR CODE HERE- WHAT HAPPENS EACH TIME THE BUTTON IS CLICKED
// you might need to call these methods to update the display
setupSortPane(selectionSortPane, SSORT_Y_START, selectionArray, Color.BLUE);
setupSortPane(insertionSortPane, ISORT_Y_START, insertionArray, Color.RED);
}
public static void main(String[] args) {
launch(args);
}
}
Several places in the code have placeholders for you to add code: // ??? YOUR CODE HERE
You might also need to add code to additional places (including declaring instance data variables).
The code for the two sorting algorithms is posted on Canvas.
This is the original sorting algorithm code. You will need to modify it for the project.
For this program, you will need to "deconstruct" the sorting algorithms. You essentially want only one pass of the outer loop (for each algorithm) to happen with each click of the button. Think about how you can take apart that outer loop:
Where should you put the initialization part of the loop? This happens one time. Where should it go?
Where should you put the conditional check of the loop?
Where should you put the update condition of the loop?
Use the two private helper methods provided. You can include additional helper methods if necessary.
Extra Credit A (10 points)
Initialize your arrays with unique random numbers (i.e., no number may appear more than once in your array).
Mark your code with // EXTRA CREDIT HERE where you are initializing the array, to be sure I don't miss it!
Extra Credit B (10 points)
When the sort is complete, change the color of the bars to green.
Sorting GU Click to take one step in the sort ni ic Til Selection Sort Oh Insertion Sort
Step by Step Solution
There are 3 Steps involved in it
Step: 1
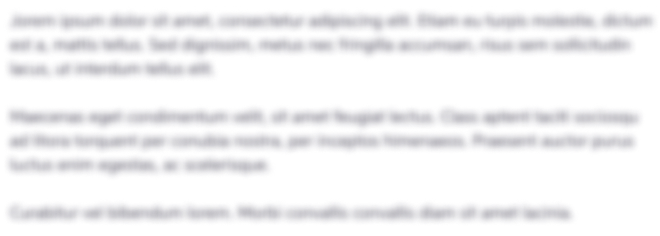
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started