-write assembly language programs to: -define a recursive procedure/function and call it. -use syscall operations to display integers and strings on the console window -use
-write assembly language programs to: -define a recursive procedure/function and call it. -use syscall operations to display integers and strings on the console window -use syscall operations to read integers from the keyboard.
Assignment Description:
Implement a MIPS assembly language program that defines "main", and "function1" procedures.
The function1 is recursive and should be defined as:
function1(n) = (3*n)-5 if n <= 3
= (n-1)*function1(n-1) + function1(n-2) - n otherwise.
The main asks a user to enter an integer for n and calls the function1 by passing the n value, then prints the result. If your program causes an infinite loop, press Control and 'C' keys at the same time to stop it. Name your source code file assignment7.s.
C program that will ask a user to enter an integer, calls the fuction1, and prints the returned value from the function1.
// The function1 is a recursive procedure/function defined by: // function1(n) = (3*n)-5 if n <= 3 // = (n-1)*function1(n-1) + function1(n-2) - n otherwise. int function1(int n) { if (n <= 3) { int ans1 = (3*n)-5; return ans1; } else { int ans1 = (n-1)*function1(n-1) + function1(n-2) - n; return ans1; } } // The main calls function1 by entering an integer given by a user. void main() { int ans, n; printf("Enter an integer: "); // read an integer from user and store it in "n" scanf("%d", &n); ans = function1(n); // print out the solution computed by function 1 printf("The solution is: %d ", ans); return; }
The following is a sample output (user input is in bold):
Enter an integer: 8 The solution is: 7842
--------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
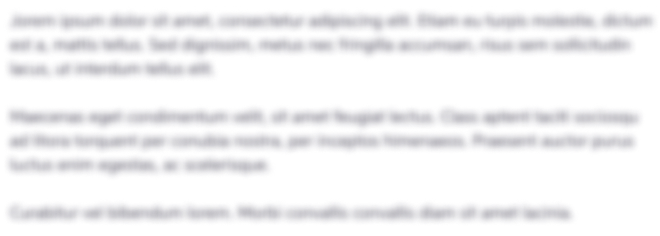
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started