Question
Write C programs queue.h, queue.c to implement the queue data structure by a linked list. queue.h contains the following type definition and function prototypes. queue.c
Write C programs queue.h, queue.c to implement the queue data structure by a linked list. queue.h contains the following type definition and function prototypes. queue.c contains the implementation of the functions.
- definition of queue node structure type QNODE which contains int data and queue node next pointer.
- void enqueue(QNODE **frontp, QNODE **rearp, int value); this creates a node and sets its data to value and inserts it to the rear end of the queue passed by frontp and rearp.
- int dequeue(QNODE **frontp, QUEUE **rearp); this gets the data of front node, deletes the front node and returns the data.
- int peek(QNODE *front); this returns the data of front node.
- Write the main function program a6q2.c to test the above functions.
queue.h
#ifndef QUEUE_H #define QUEUE_H #include#include typedef struct node { int data; struct node *next; } QNODE; void enqueue(QNODE **frontp, QNODE **rearp, int); int dequeue(QNODE **frontp, QNODE **rearp); int peek(QNODE *front); void clean(QNODE **frontp, QNODE **rearp); #endif
queue.c
#include "queue.h" void enqueue(QNODE **frontp, QNODE **rearp, int val) { // your code } int dequeue(QNODE **frontp, QNODE **rearp) { // your code } int peek(QNODE *front) { // your code } void clean(QNODE **frontp, QNODE **rearp) { // your code }
this can be tested by the following code below
#include
#include "queue.h"
int main(int argc, char* args[]) { QNODE *front = NULL, *rear = NULL; int i=0; for (i=1; i<=12; i++) { enqueue(&front, &rear, i); } printf("%d ", peek(front)); dequeue(&front, &rear); printf("%d ", dequeue(&front, &rear)); printf(" "); while (front != NULL) { printf("%d ", dequeue(&front, &rear)); } for (i=1; i<=12; i++) { enqueue(&front, &rear, i); } clean(&front, &rear);
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
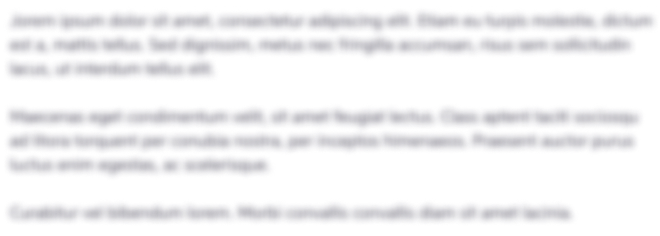
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started