Question
Write in Python a program, blackjack.py , which simulates a special version of Blackjack. In Blackjack, cards are drawn from a deck onto a playing
Write in Python a program, blackjack.py, which simulates a special version of Blackjack. In Blackjack, cards are drawn from a deck onto a playing hand, all the card values of a playing hand added, and the hand closest or equal to 21 wins. If the value of a playing hand is > 21, the corresponding player is "busted" and looses.
The rules in this version of Blackjack are the following:
The value of the cards 2-10, is the card itself.
The value of a face card (jack, queen, and king) is 10.
The value of an ace is 11 (but not 1).
A playing hand consists of 2-5 cards.
Cards of a playing hand are drawn from a deck of 52 cards.
There are two players: Player and Dealer.
One card at a time is dealt. While the value of a playing hand is < 17, the player receives more cards (but not more than 5).
Rules about winners:
If Player is "busted", Dealer wins.
If Dealer is "busted" but not Player, then Player wins.
If neither Player nor Delaer is "busted", then the one wins who's hand is closest to 21. Dealer wins if the hands are equal.
In the implementation, the following is given (which you HAVE TO use):
The function initialize_deck() which initializes a deck (shuffles the cards) in random manner. A deck is implemented as a list of size 52. Each element in the list is a string. The suit is not stored, only the card value.
Various constants.
The start of the main program.
The signature and the docstring for the functions deal(), get_card_value() and get_winner(). You must implement these functions.
The functionality of the program is the following:
At the start, the user is asked to input a seed used to initialize the random number generator and an integer stating how many rounds should be played.
In each round/iteration:
A deck is created.
Player is dealt 2-5 cards from the deck.
Dealer is dealt 2-5 cards from the deck.
The value of Player hand is written out, as well as the cards of the hand.
The value of Dealer hand is written out, as well as the cards of the hand.
The winner is written out.
Finally, information is written out showing how often Player won and how often Dealer won.
Here are two examples of the input/output of the program:
Random seed: 8
Number of rounds: 1
Player: 23 -> 3 Q K
Dealer: 21 -> 10
A Dealer won!
Player won 0 times
Dealer won 1 times
import random
JACK = 'J'
QUEEN = 'Q'
KING = 'K'
ACE = 'A'
LOWER_CARDS = ['2','3','4','5','6','7','8','9','10']
FACE_VALUE = 10 # The value of the jack, queen and king
ACE_VALUE = 11 # The value of an ace
MAX_HAND_VALUE = 21 # maximum sum of cards of a hand before being busted
MAX_HAND_CARDS = 5 # maximum number of cards in a hand
WINNER_PLAYER = 0 # means player wins a game
WINNER_DEALER = 1 # means dealer wins a game
def main():
'''Asks for a random seed and then starts the game loop.'''
seed = int(input('Random seed: '))
random.seed(seed)
number_of_rounds = int(input('Number of rounds: '))
# Your main program continues here
def create_deck():
'''Creates and returns a 52-card deck.'''
deck = []
for _ in range(0,4):
for card in range(2,11):
deck.append(str(card))
deck.append(JACK)
deck.append(QUEEN)
deck.append(KING)
deck.append(ACE)
random.shuffle(deck)
return(deck)
def deal(deck):
'''Deals cards from the given deck to a hand until
the sum of cards >= a constant or number of cards = a constant.
The dealt cards are removed from the deck at the right end,
and returned in a list.'''
pass
def get_card_value(card):
'''Returns the integer value of the given card,
which is represented as a string.'''
pass
def get_winner(player_hand, dealer_hand):
'''Given two lists of cards (player_hand, dealer_hand),
returns either WINNER_DEALER or WINNER_PLAYER, signifying
the dealer_hand or the player_hand as a winner respectively.'''
pass
if __name__ == "__main__":
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
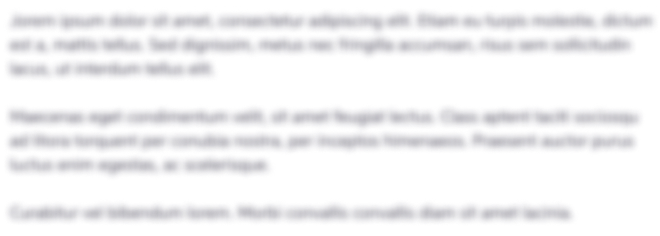
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started