Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write python code for this prompt: NOTE: This assignment is meant to be completed over three weeks. As such, it will likely be longer and
Write python code for this prompt: NOTE: This assignment is meant to be completed over three weeks. As such, it will
likely be longer and more complicated than past assessments. We recommend you start on
this assessment early and not wait until after the end of Spring Break to begin work on it
Introduction
In this assessment youll use functions and nested loops to write code to analyze a grid
of blackandwhite pixels to determine which letter it most resembles.
Problem
Computers have only recently been able to reliably recognize writing, and still cant
in certain difficult conditions. For this assignment, you will code a simplified version of a
writing recognition system. This code will only deal with single letters at a time a much
more approachable task than reading general writing
Importantly, letter data should always be considered in a x grid of black and white
pixels. In practical terms, this grid will be a list of lists, with each row of the grid represented
by its own sublist. Each pixels value will be for a black pixel or for a white one
eg the following list:
# list formatted for readability
lettergrid
would be rendered as a blackandwhite image like so:
Figure : A capital A in a x pixel grid
Your code will determine which letter best matches the given input grid by comparing
two grids together: one grid that is unknown and one grid that represents a known letter.
You will assign each of these comparisons a score that is how many pixels the two grids
have in common.
For example, take the following input image of an unknown letter.
Figure : A pixel grid with an as yet unidentified letter.
We can compare how many pixels this grid has in common with a known A and a known
B grid.
Figure : Two known letter grids for A and B
The unknown grid has pixels that match with the A grid and only pixels in
common with the B grid. Thus, we would conclude the unknown grid is an A because it
has the highest match score
Using a given list of letter grids, you will write code to manage and make these compar
isons. Like with the last assessment, you will accomplish this through a series of functions
you will define and implement.
Required Functions
get input grid
Parameters
There should not be any parameters for this function
Returns
This function should return EITHER a list of lists of integers or a single integer, de
pending on what is collected by the function through user input, ie the input function.
This input will normally be a series of grid rows, with pixel values separated by spaces. For
example, this function could cause the following interaction and would return the list in the
Example Usage section below.
ROW
ROW
ROW
ROW
ROW
However, if the user inputs DONE at any point then this function should immediately halt
and return instead.
ROW
ROW
ROW DONE # Function stops asking for input and returns a
Description
Unlike last week, this function will need to make use of the input function to collect
user input. You should accept at most lines of input, and convert each input line into a list
of integers. Besides checking for DONE, this function should not do any input validation.
That will be done by the following function.
Example usage
pixelgrid getinputgrid
#assuming the user inputs the rows in the example above
printpixelgrid # prints:
# list formatted for readability
#
#
#
grid is validpixel grid
Parameters
A list of lists of integers
Returns
This function should return a Boolean value indicating if the pixel grid parameter meets
the format requirements for analysis.
Description
The format requirements are:
The grid must be a list of exactly five lists
Each nested list must contain exactly integers, each with values of or
If the provided grid fails to meet these requirements, this function should return False.
Example usage
pixelgrid
isvalid gridisvalidpixelgrid
printisvalid # False
match scorepixel grid, letter grid
Parameters
A list of lists of integers
A list of lists of integers
You can assume these parameters have already been validated, and thus both will be exactly
x grids of integers that are or
Returns
This function should return an integer representing how many individual pixel values
from pixel grid matched pixels in the same location from letter grid.
Description
This function will need to loop over the pixel locations in the provided pixel grid, and
count how many individual values match with values in letter gri
Step by Step Solution
There are 3 Steps involved in it
Step: 1
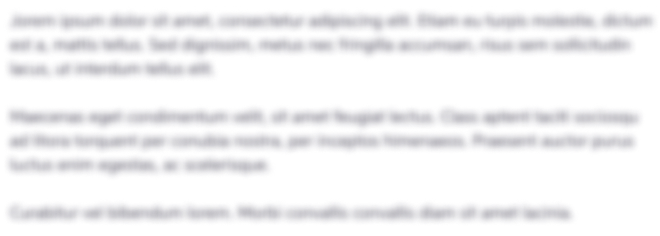
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started