Question
Write the code for the following: Modify the program so that the formatted output is shown to the screen and is also written to a
Write the code for the following:
Modify the program so that the formatted output is shown to the screen and is also written to a text file called `healthResults.txt
You will need to open the file for writing, ensure it is open (or else exit the program with an error) and close the file when finished writing to it. #include
#include
#includeusing namespace std;
int main() {
// Constants for activity levels
const double SEDENTARY = 1.2;
const double LIGHT_EXERCISE = 1.375;
const double MODERATE_EXERCISE = 1.55;
const double HEAVY_EXERCISE = 1.725;// Constants for unit conversions
const double INCHES_TO_METERS = 0.0254;
const double LBS_TO_KGS = 0.453592;// Variables to store user input
string firstName, lastName, sex;
int age;
double heightInInches, weightInLbs;// Prompt user for information
cout << "Health Metrics Calculator 1.0" << endl << endl;
cout << "Please enter the following information:" << endl;
cout << "First Name: ";
cin >> firstName;
cout << "Last Name: ";
cin >> lastName;
cout << "Sex (Male/Female): ";
cin >> sex;
cout << "Age: ";
cin >> age;
cout << "Height (in inches): ";
cin >> heightInInches;
cout << "Weight (in lbs): ";
cin >> weightInLbs;// Convert units
double heightInMeters = heightInInches * INCHES_TO_METERS;
double weightInKg = weightInLbs * LBS_TO_KGS;// Calculate BMI
double bmi = weightInKg / (heightInMeters * heightInMeters);// Calculate BMR based on sex
double bmr;
if (sex == "Male") {
bmr = 88.362 + (13.397 * weightInKg) + (4.799 * heightInMeters * 100) - (5.677 * age);
} else if (sex == "Female") {
bmr = 447.593 + (9.247 * weightInKg) + (3.098 * heightInMeters * 100) - (4.330 * age);
} else {
cout << "Invalid input for sex. Please enter Male or Female." << endl;
return 1;
}// Calculate calories needed to maintain weight for different activity levels
double sedentaryCalories = bmr * SEDENTARY;
double lightExerciseCalories = bmr * LIGHT_EXERCISE;
double moderateExerciseCalories = bmr * MODERATE_EXERCISE;
double heavyExerciseCalories = bmr * HEAVY_EXERCISE;// Display the results
cout << "\nConverting units..." << endl;
cout << fixed << setprecision(3);
cout << "Height in meters: " << heightInMeters << endl;
cout << "Weight in kg: " << weightInKg << endl;
cout << "\nCalculating metrics..." << endl;
cout << "BMI: " << bmi << endl;
cout << "BMR: " << bmr << " kcal/day" << endl;
cout << "\n----------------------------------------------" << endl;
cout << "Health Metrics Summary" << endl;
cout << "----------------------------------------------" << endl;
cout << "Name: " << firstName << " " << lastName << endl;
cout << "Sex: " << sex << endl;
cout << "Age: " << age << endl;
cout << "Height: " << heightInMeters << " meters" << endl;
cout << "Weight: " << weightInKg << " kg" << endl;
cout << "\nMetrics:" << endl;
cout << "- BMI: " << bmi << endl;
cout << "- BMR: " << bmr << " kcal/day" << endl;
cout << "\nCalories to Maintain Weight based on Activity Level:" << endl;
cout << "- Sedentary: " << sedentaryCalories << " kcal/day" << endl;
cout << "- Light Exercise: " << lightExerciseCalories << " kcal/day" << endl;
cout << "- Moderate Exercise: " << moderateExerciseCalories << " kcal/day" << endl;
cout << "- Heavy Exercise: " << heavyExerciseCalories << " kcal/day" << endl;
cout << "\nThank you for using the Health Metrics Calculator!" << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
include iostream include fstream include iomanip using namespace std int main Constants and variable...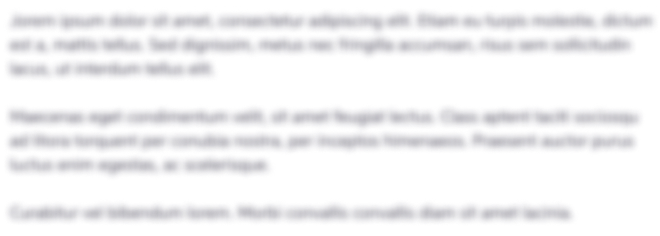
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started