Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write two programs called echo _ client.py and echo _ server.py , respectively. The echo _ client.py aims to connect and send messages to the
Write two programs called echoclient.py and echoserver.py respectively. The
echoclient.py aims to connect and send messages to the echo server. The echo server
can echo back the same message back to the client. Partial code of echoserver.py and
echoclient.py are provided in the course blackboard. You can download them from
the assessment folder to complete the code.
Run echo server using the following command:
$ python echoserver.py
Where portnumber refers to the port number that the echo server will listen to
Run echo client using the following command:
$ python echoclient.py
Where portnumber refers to the server port number that the client will connect to
The echo server will send back the exact message that it receives.
The client will print the returned message to clients stdout:
$ python echoclient.py # run the echo client
Hello World # client sends a message to the server.
Server Hello World # response from the server.
ECHOSERVER
import socket
import sys
class EchoServer:
def initself:
self.addr None
self.port None
self.socket None
self.host
self.conn None
def readportnumberself:
Read the port number from argument, store it to self.port.
Exit with status if invalid argument is provided.
if lensysargv:
printUsage: python echoserver.py
sysexit
try:
self.port intsysargv
except ValueError:
printInvalid port number"
sysexit
def listenonportself:
Create a socket listens on the specified port.
Store the socket object to self.socket.
Store the new accepted connection to self.conn.
self.socket socket.socketsocketAFINET, socket.SOCKSTREAM
self.socket.bindselfhost, self.port
self.socket.listen
printfListening on port selfport
self.conn, addr self.socket.accept
printfAccepted connection from addr
def receivemessageself:
Receive a TCP packet from the client.
:return: the received message
try:
data self.conn.recv
if not data:
printConnection closed by the client"
sysexit
return data.decode
except Exception as e:
printfError receiving message: e
return None
def sendmessageself msg:
Send a message back to the client
:param msg: the message to send to the client
:return: None
try:
self.conn.sendallmsgencode
except Exception as e:
printfError sending message: e
def echomessagesself:
Use a while loop to echo messages back to client
:return: None
while True:
try:
data self.receivemessage
if not data:
printConnection closed by the client"
break
printfReceived message: data
self.sendmessagedata # Send the received message back to the client
except Exception as e:
printfError receiving message: e
break
def runechoserverself:
Run the echo server to receive and echo back messages
:return: None
self.readportnumber
self.listenonport
self.echomessages
if namemain:
echoserver EchoServer
echoserver.runechoserver
ECHOCLIENT
import socket
import sys
class EchoClient:
def initself:
self.host
self.port None
self.socket None
def readportnumberself:
Read the port number from argument, store it to self.port.
Exit if invalid argument is provided.
:return: None
if lensysargv:
sysexit
try:
self.port intsysargv
except ValueError:
printInvalid port number"
sysexit
def connecttoportself:
Create a socket to try to connect to a specified port,
store the new socket object to self.socket.
:return: None
self.socket socket.socketsocketAFINET, socket.SOCKSTREAM
try:
self.socket.connectselfhost, self.port
except Exception as e:
printfError connecting to server: e
sysexit
def receiveandprintmessageself:
Receive a TCP packet from the server and print
Step by Step Solution
There are 3 Steps involved in it
Step: 1
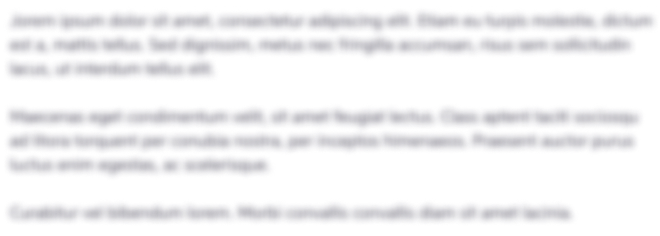
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started