Question
Written in C, requires linked lists. Please answer the 4 questions and show the output. Thank you! // Write your name here // Write the
Written in C, requires linked lists. Please answer the 4 questions and show the output. Thank you!
// Write your name here // Write the compiler used: Visual studio or gcc // Reminder that your file name is incredibly important. Please do not change it. // Reminder that we are compiling on Gradescope using GCC. // READ BEFORE YOU START: // You are given a partially completed program that creates a linked list of game items like you'd see in a folder. // Each item has this information: item name, game name, type of item, item ID. // The struct 'itemRecord' holds the information of one item. Variety is an enum. // A linked list of structs called 'list' is declared to hold the list of items. // To begin, you should trace through the given code and understand how it works. // Please read the instructions above each required function and follow the directions carefully. // You should not modify any of the given code, the return types, or the parameters. Otherwise, you risk getting compilation errors. // You are not allowed to modify main(). // You can use all string library functions. // You will have to write your functions from scratch by looking at what is expected to be passed into them in the pre-existing functions // WRITE COMMENTS FOR IMPORANT STEPS IN YOUR CODE. #include
char* fileName = "Item_List.txt"; load(fileName); // load list of items from file (if it exists). Initially there will be no file. char choice = 'i'; // initialized to a dummy value do { printf(" Enter your selection: "); printf("\t a: add a new item "); printf("\t d: display item list "); printf("\t r: remove a item from list "); printf("\t s: sort item list by ID "); printf("\t q: quit "); choice = getchar(); flushStdIn(); executeAction(choice); } while (choice != 'q'); save(fileName); // save list of items to file (overwrites file if it exists) return 0; } // flush out leftover ' ' characters void flushStdIn() { char c; do c = getchar(); while (c != ' ' && c != EOF); } // ask for details from user for the given selection and perform that action void executeAction(char c) { char itemName_input[MAX_NAME_LENGTH], gameName_input[MAX_NAME_LENGTH]; unsigned int itemId_input, add_result = 0; char itemtype_input[20]; switch (c) { case 'a': // input item record from user printf(" Enter item name: "); fgets(itemName_input, sizeof(itemName_input), stdin); itemName_input[strlen(itemName_input) - 1] = '\0'; // discard the trailing ' ' char printf("Enter game name: "); fgets(gameName_input, sizeof(gameName_input), stdin); gameName_input[strlen(gameName_input) - 1] = '\0'; // discard the trailing ' ' char printf("Enter item type (Health/Equip/Etc): "); fgets(itemtype_input, sizeof(itemtype_input), stdin); itemtype_input[strlen(itemtype_input) - 1] = '\0'; // discard the trailing ' ' char printf("Please enter item ID number: "); scanf("%d", &itemId_input); flushStdIn(); // add the item to the list add_result = add(itemName_input, gameName_input, itemtype_input,
itemId_input); if (add_result == 0) printf(" Item is already on the list! "); else if (add_result == 1) printf(" Item successfully added to the list! "); else printf(" Unable to add the item. the Item list is full! "); break; case 'r': printf("Please enter ID number of item to be deleted: "); scanf("%d", &itemId_input); flushStdIn(); int delete_result = delete(itemId_input); if (delete_result == 0) printf(" Item not found in the list! "); else printf(" Item deleted successfully! "); break; case 'd': display(); break; case 's': sort(); break; case 'q': break; default: printf("%c is invalid input! ", c); } } // This function displays the item list with the details (struct elements) of each item. // Display all items. void display() { struct itemRecord* tempList = list; // work on a copy of 'list' char* itemTypeString = "NoType"; // dummy init while (tempList != NULL) { // traverse all items in the list printf(" Item Name: %s", tempList->itemName); // display the item name printf(" Game name: %s", tempList->gameName); // display the game name if (tempList->variety == Health) // find what to display for item type itemTypeString = "Health"; else if(tempList->variety == Equip) itemTypeString = "Equip"; else itemTypeString = "Etc"; printf(" Item Type: %s", itemTypeString); // display item type printf(" Item ID: %d", tempList->itemID); // display item id printf(" ");
tempList = tempList->next; } } // save() is called at the end of main() // This function saves the linked list of structures to file. // save() is called at end of main() to save the item list to a file. // The file is saved at the same place as your C file. For VS, the default directory looks like this: // C:\Users\
// Q2 : sort (10 points) // This function is used to sort the list (linked list of structs) numerically by item ID. // Parse the list and compare the item IDs to check which one should appear before the other in the list. // Sorting should happen within the list. That is, you should not create a new node of structs having sorted items. // Please use this print statement to print after successfully sorting the list: // printf(" Item list sorted! Use display option 'd' to view sorted list. "); // Q3 : delete (10 points) // This function is used to delete an item by ID. // Parse the list and compare the item IDs to check which one should be deleted. // Return 0 if the specified ID was not found. Return 1 upon successful removal of a record. // Q4: load (10 points) // This function is called in the beginning of main(). // This function reads the item list from the saved file and builds the linked list of structures 'list'. // In the first run of the program, there will be no saved file because save() is called at the end of program. // So, at the begining of this function, write code to open the file and check if it exists. If file does not exist, then return from the function. // (See the expected output of add() in homework the question file. It displays "Item_List.txt not found" because the file did not exist initially.) // If the file exists, then parse the item list to read the item details from the file. // Use the save function given above as an example of how to write this function. Notice the order in which the struct elements are saved in save() // You need to use the same order to read the list back. // NOTE: The saved file is not exactly readable because all elements of the struct are not string or char type. // So you need to implement load() similar to how save() is implemented. Only then the 'list' will be loaded correctly. // You can simply delete the file to 'reset the list' or to avoid loading from it. // You'll need to use the following two print statements in your code: // printf("Item record loaded from %s. ", fileName); // printf("%s not found. ", fileName);
expected output for add:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
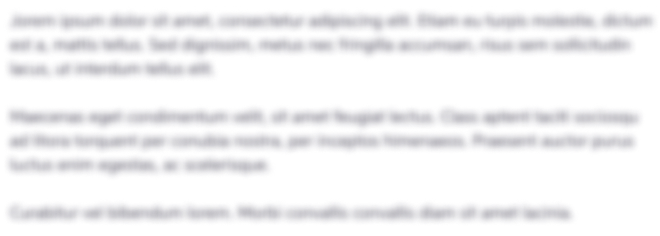
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started