Question
Yes, it's a java question. public interface Tree extends Iterable{ Position root(); Position parent(Position p)throws IllegalArgumentException; Iterable> children(Position p)throws IllegalArgumentException; int numChildren(Position p)throws IllegalArgumentException; boolean
Yes, it's a java question.
public interface Tree extends Iterable{ Position root(); Position parent(Position p)throws IllegalArgumentException; Iterable> children(Position p)throws IllegalArgumentException; int numChildren(Position p)throws IllegalArgumentException; boolean isInternal(Position p)throws IllegalArgumentException; boolean isExternal(Position p)throws IllegalArgumentException; boolean isRoot(Position p)throws IllegalArgumentException; int size(); boolean isEmpty(); Iterator iterator(); Iterable> positions(); }
public class LinkedBinaryTree extends AbstractBinaryTree{
protected static class Node implements Position { private E element; private Node parent; private Node right; private Node left; public Node(E e,Node above,Node leftChild,Node rightChild) { element=e; parent=above; left=leftChild; right=rightChild; } public E getElement() { return element; } public Node getParent() { return parent; } public Node getLeft() { return left; } public Node getRight() { return right; } public void setElement(E e) { element=e; } public void setParent(Node parentNode) { parent=parentNode; } public void setLeft(Node leftChild) { left=leftChild; } public void setRight(Node rightChild) { right=rightChild; } } protected Node root=null; private int size=0; public LinkedBinaryTree() {} protected Node createNode(E e,Node parent,Node left,Node right) { return new Node(e,parent,left,right); } protected Node validate(Position p) throws IllegalArgumentException{ if(!(p instanceof Node)) { throw new IllegalArgumentException("Not valid position type"); } Node node = (Node)p; if(node.getParent()==node) { throw new IllegalArgumentException("p is no longer in the tree"); } return node; } public int size() { return size; } public Position root() { return root; } public Position parent(Position p)throws IllegalArgumentException{ Node node=validate(p); return node.getParent(); } public Position left(Position p)throws IllegalArgumentException{ Node node=validate(p); return node.getLeft(); } public Position right(Position p)throws IllegalArgumentException{ Node node=validate(p); return node.getRight(); } public Position addRoot(E e )throws IllegalArgumentException{ if(!isEmpty()) { throw new IllegalArgumentException("Tree is not Empty"); } root=createNode(e,null,null,null); size=1; return root; } public Position addLeft(Position p,E e)throws IllegalArgumentException { Node parent=validate(p); if(parent.getLeft()!=null) { throw new IllegalArgumentException("P already has left child"); } Node child = createNode(e,parent,null,null); parent.setLeft(child); size++; return child; } public Position addRight(Position p,E e)throws IllegalArgumentException { Node parent=validate(p); if(parent.getRight()!=null) { throw new IllegalArgumentException("P already has right child"); } Node child = createNode(e,parent,null,null); parent.setRight(child); size++; return child; } public E set(Position p,E e) { Node node=validate(p); E temp=node.getElement(); node.setElement(e); return temp; } public E remove(Position p)throws IllegalArgumentException{ Node node=validate(p); if(numChildren(p)==2) { throw new IllegalArgumentException("p has two children"); } Node child = (node.getLeft() != null ? node.getLeft(): node.getRight()); if(child != null) { child.setParent(node.getParent()); } if(node==root) { root= child; } else { Node parent = node.getParent(); if(node==parent.getLeft()) { parent.setLeft(child); } else { parent.setRight(child); } } size--; E temp=node.getElement(); node.setElement(null); node.setLeft(null); node.setRight(null); node.setParent(node); return temp; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
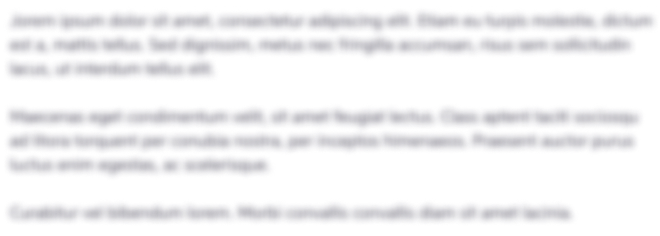
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started