Question
// You are given a partially completed program that creates a list of students. // Each student has the corresponding information: name, roll number and
// You are given a partially completed program that creates a list of students.
// Each student has the corresponding information: name, roll number and student type.
// This information is stored as an object of Student class.
// The classes Undergrad and Grad are child classes of the Stduent class.
// When adding a new student, these child classes are used to make the student node of the list.
//
// To begin, you should trace through the given code and understand how it works.
// Please read the instructions above each required function and follow the directions carefully.
// Do not modify given code.
//
// You can assume that all input is valid:
// Valid name: String containing alphabetical letters
// Valid roll number: a positive integer
#include
#include
#include
#include "Container.h"
#include "student.h"
#include "grad.h"
#include "undergrad.h"
using namespace std;
// forward declarations of functions already implemented:
void executeAction(char c);
Student* searchStudent(string name_input);
// forward declarations of functions that need implementation:
void addStudent(string name_input, int rollNo_input, studentType type); // 7 points
void displayList(); // 4 points
void save(string fileName); // 7 points
void load(string fileName); // 7 points
Container* list = NULL; // global list
int main()
{
char c = 'i'; // initialized to a dummy value
load("list.txt"); // During first execution, there will be no list.txt in source directory. list.txt is generated by save() while exiting the program.
do {
cout << " CSE240 HW10 ";
cout << "Please enter your selection: ";
cout << "\t a: add a new student to the list ";
cout << "\t d: display list of students ";
cout << "\t c: change roll number of a student ";
cout << "\t q: quit ";
cin >> c;
cin.ignore();
executeAction(c);
} while (c != 'q');
save("list.txt");
list = NULL;
return 0;
}
// Ask for details from user for the given selection and perform that action
// Read the function case by case
void executeAction(char c)
{
string name_input;
int rollNo_input;
int type_input = 2;
studentType type;
Student* studentFound = NULL;
switch (c)
{
case 'a': // add student
// input student details from user
cout << endl << "Enter student's name: ";
getline(cin, name_input);
cout << "Enter roll number: ";
cin >> rollNo_input;
cin.ignore();
while (!(type_input == 0 || type_input == 1))
{
cout << endl << "Enter student type: " << endl;
cout << "0. Undergrad " << endl;
cout << "1. Grad" << endl;
cin >> type_input;
cin.ignore();
}
type = (studentType)type_input;
// searchStudent() will return the student node if found, else returns NULL
studentFound = searchStudent(name_input);
if (studentFound == NULL)
{
addStudent(name_input, rollNo_input, type);
cout << endl << "Student added to list!" << endl << endl;
}
else
cout << endl << "Student already present in the list!" << endl << endl;
break;
case 'd': // display the list
displayList();
break;
case 'c': // change roll number
cout << endl << "Enter student's name: ";
getline(cin, name_input);
// searchStudent() will return the student node if found, else returns NULL
studentFound = searchStudent(name_input);
if (studentFound == NULL)
{
cout << endl << "Student not in list!" << endl << endl;
}
else
{
// if stduent exists in the list, then ask user for new roll number
cout << endl << "Enter new roll number: ";
cin >> rollNo_input;
cin.ignore();
// Q3c Call changeRollNo() here (1 point)
// 'studentFound' contains the student whose roll number is to be changed.
// 'rollNo_input' contains the new roll number of the student.
// Call the function with appropriate function arguments.
void changeRollNo(Student *studnet, int RollNo);
cout << endl << "Roll number changed!" << endl << endl;
}
break;
case 'q': // quit
break;
default: cout << c << " is invalid input! ";
}
}
// No implementation needed here, however it may be helpful to review this function
Student* searchStudent(string name_input)
{
Container* tempList = list; // work on a copy of 'list'
while (tempList != NULL) // parse till end of list
{
if (tempList->student->getName() == name_input)
{
return tempList->student; // return the student if found
}
tempList = tempList->next; // parse the list
}
return NULL; // return NULL if student not found in list
}
// Q3b: Define Friend Function changeRollNo() (3 points)
// Define the function changeRollNo()that is declared in student.h file.
// This function sets the new roll number of the student. The student and new roll number is to be passed as function arguments.
// Call this function in case 'c' of executeAction(). While testing, use 'd' display option after using 'c' option to verify whether the new roll number is set.
// You will need to implement add() and displayList() before you test this function.
// Q4: addStudent (7 points)
// This function is used to add a new student to the global linked list 'list'. You may add the new student to head or tail of the list. (Sample solution adds to tail)
// New student can be either undergrad or grad. You will need to use the function argument type to determine which constructor to use to create new student node.
// For example, if the user enters undergrad student, then you need to use Undergrad class and constructor to create new student node and add it to the list.
// NOTE: In executeAction(), searchStudent() is called before this function. Therefore no need to check here if the student exists in the list.
// See how this fucntion is called in case 'a' of executeAction()
void addStudent(string name_input, int rollNo_input, studentType type)
{
Container* tempList = list; // work on a copy of 'list'
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
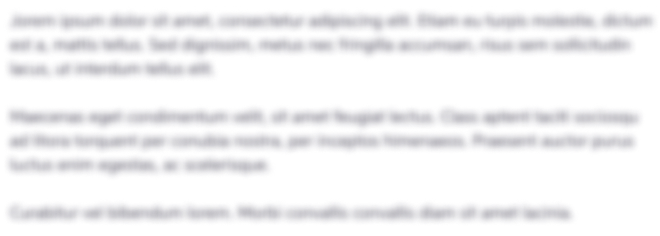
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started