Question
You are to write a C++ program to support the concatenation of arrays. The program will dynamically generate a new array consisting of two smaller
You are to write a C++ program to support the concatenation of arrays. The program will dynamically generate a new array consisting of two smaller arrays. Program Requirements: The program must contain and use the following functions: ConcatArray GetArrayN DisplayArray ReverseArray ScrambleArray
Each of the array functions must go in the file arraytools.cpp. Each of the functions should be prototyped in arraytools.h. To practice with pointer notation, you must use pointer notation when accessing arrays. *(Ptr + i) // ------ use this Ptr[i] // -------- not this The functions must be prototyped above the main () via include files. The program shall ask the user if he/she would like to run the process again. The user will enter y to continue or n to quit. The program source code shall be written in C++. The code should have comments. The code should be easily readable and should have proper indentation. The program shall compile with no errors or warnings. Detailed Design: Array Protocol: Every array in this program shall follow this protocol. All arrays that you declare and all arrays generated shall follow this protocol.
All Arrays are of short int type.
The first element in every array shall contain the total number of elements in the array including the first element itself.
Example: short int MyArray[5] = { 5, 10, 20, 33, 44 }; // 5 elements in the array, so first element = 5
Arrays and Functions: All arrays that are passed to functions shall be passed via a separate pointer variable. Example: short int MyArray[] = { 5, 10, 20, 33, 44 }; short int *Ptr; Ptr = &MyArray[0]; f1 ( Ptr ); // avoid using f1 (MyArray); or f1 (&MyArray[0]); // be sure to create a separate pointer variable Function Design Function Name:ConcatArray Inputs: This function takes in two parameters. Both parameters are pointers to short ints which refer to arrays. Return: This returns the address of new array that was created using dynamic memory allocation. Description: This function take in two pointers as parameters. These pointers are pointers to arrays. The function will create a new array using dynamic memory allocation. The new array will consist of ALL of the elements in the first array and all of the elements in the second array. The two arrays will simply be concatenated together in a new array. The function will return the address of the new array.
Example: Let Arr1 = {5, 1,2,3,4} and Arr2 = {5, 10, 20, 30, 40 }; If Arr1 and Arr2 were passed to this function, the new array would look like the following: NewArr = { 11, 5, 1, 2, 3, 4, 5, 10, 20, 30, 40 } Function Name:GetArrayN Inputs: This function takes in two parameters. One parameter is a pointer to a short int, the other is an integer. Return: This examines the array and finds array n that is embedded in orig. It returns that as a new array (dynamic memory). Description: n represents the array number that is embedded in the array.
NOTE - This function should work with any concatenated array with any size sub arrays.
Example: Assume the input parameters looked like this: orig: { 11, 5, 1, 2, 3, 4, 5, 10, 20, 30, 40 } n: 1 Note: With a value of n=1, the array 5,1,2,3,4 is returned since it is the first array embedded in orig. This would return a new dynamically allocated array with the values: {5,1,2,3,4} Example: Assume the input parameters looked like this: orig: { 11, 5, 1, 2, 3, 4, 5, 10, 20, 30, 40 } n: 2 Note: With a value of n=2, the array 5,10,20,30,40 is returned since it is the first array embedded in orig. This would return a new dynamically allocated array with the values: {5,10,20,30,40} Function Name: DisplayArray Inputs: A pointer to a short int Return: nothing Description
: This function will take as a parameter a pointer to a short int. This should be a pointer to an array. The function shall loop thru and print out each element of the array include the first element. The array should be displayed horizontally on the screen. There should be a space between each element printed and a line break after the last element is printed.
Function Name: ReverseArray Inputs: A pointer to a short int Return: nothing Description This function takes in a pointer to a short int as a parameter. This should be a pointer to an array. The function will reverse all of the elements in the array except for the first element. Remember that the first element should always contain the number of elements in the array. The function does not need to return anything as it will permanently change the values in the array. Example: Let Arr1 = { 5, 1, 2, 3, 4 } Assume Arr1 was passed in to the function. After this function executes, the array would look like the following: Arr1 = { 5, 4, 3, 2, 1} Note that this first element does not change. Function Name :ScrambleArray Inputs: A pointer to a short int Return: nothing Description
Description : This function takes in a pointer to a short int as a parameter. This function scrambles the array by randomly changing the positions of all of the elements except the first item. You must use a random number generator to choose the positions. Ensure that your random number generator is only seeded once in the during the program.
Example: Let Arr1 = { 5, 1, 2, 3, 4 }
Calling scramble the first time may result in the array being { 5, 3, 4, 1, 2 }.
The Header File should have these functions exactly as follows. Do not change the function names, return type, or parameter list.
void DisplayArray (short int* a);
short int* ConcatArray (short int* a1, short int* a2);
short int * GetArrayN(short int* orig, int n);
void ReverseArray( short int* arr);
void ScrambleArray ( short int* a);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
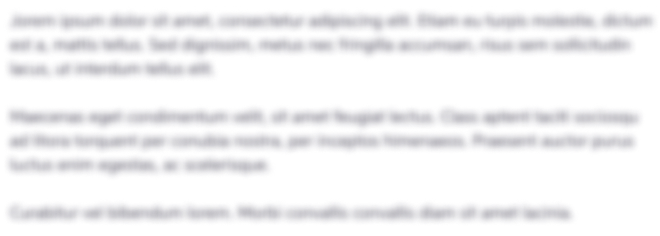
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started