Question
You are to write a program that will manipulate an array. Your program should handle up to 100 integer numbers. Label the results for each
You are to write a program that will manipulate an array. Your program should handle up to 100 integer numbers.
Label the results for each printed out below. You will have several different outputs below. Print your results in the same order given below.
For each part below, write one or more functions (there are a number of functions). Restriction: A function that does any of the calculations listed below can NOT print out the results in that function but must print out results in either the main routine or call a different function that only prints a results. A function does one task and only one task.
------------------------------------
1. Read in the values into the array. The last value is a 0 and is NOT one of the values to be used in any of the calculation below. The input is found in array.data
2. Print out all the values in the array with no more than 10 numbers per output line.
3. Print out the average of the set of numbers.
4. Print out how many values are larger than the average and how many are smaller than the average. Pass average as a parameter.
5. Convert every negative number to a positive number. Print out the array.
6. Print out the average of the set of numbers.
7. How many numbers in the array are multiples of 4 and 11?
8. Find the largest number and print out the number and its position.
9. Find the smallest number and print out the number and its position.
10. Zero out all even value numbers in the array and print out the array.
11. Print out the new average for the new array.
***** Array.data must be read in to obtain the data. Remember that the last value is a 0 and is NOT one of the values to be used in any of the calculation below. *******
***** The results must be printed out to a file. ********* The array.data file looks like:
22 27 64 7 44 26 45 66 83 62 52 -7 -36 62 78 -67 34 73 93 8 -3 -2 2 27 1 11 12 -73 83 77 37 72 82 23 0
I have this:
#include
using namespace std;
//define the array int arr[100]; int arr_length;
//function prototype void read_array(); void print_array(); double array_average(); int avg_greater(double avg); int avg_smaller(double avg); void neg_to_pos(); int no_of_multiple(int k); int largest(); int smallest(); void zero_out();
int main() {
//Task 1: Read the values in the array read_array();
//Task 2: Print array print_array();
//Task 3: Print out the average of array double avg = array_average(); cout << "Average of array is: " << avg << endl;
//Task 4(a): Find out the count of numbers which are greater than avg int max_no = avg_greater(avg); cout << "No. of values larger than average is: " << max_no << endl;
//Task 4(b): Find out the count of numbers which are greater than avg int min_no = avg_smaller(avg); cout << "No. of values smaller than average is: " << min_no << endl;
//Task 5: Convert negative number to positive number neg_to_pos();
//Task 6: Print out the average of array avg = array_average(); cout << "Average of array is:" << avg << endl;
//Task 7(a): Find the number of multiples of 4 and 11 int count1 = no_of_multiple(4); int count2 = no_of_multiple(11); cout << "No. of multiples of 4 and 11 in array are " << count1 << " and " << count2 << ",respectively" << endl;
//Task 8: Print out the largest noumber and its position int pos = largest(); cout << "Largest no. in the array is " << arr[pos] << " and it's position (starting from 1) is " << pos + 1 << endl;
//task 9: Print out the smallest no. and its position pos = smallest(); cout << "Smallest no. in the array is " << arr[pos] << " and it's position (starting from 1) is " << pos + 1 << endl;
//task 10: Zero out all the even values and print the array zero_out();
//task 11: Print out the new average of new array avg = array_average(); cout << "Average of new array is:" << avg << endl;
return 0; }
void read_array() { int i = 0, n; while (true) { cin >> n; if (n == 0) break; arr[i++] = n; } arr_length = i; }
void print_array() { int count = 0; cout << "Values of array are:" << endl; for (int j = 0; j < arr_length; j++) { cout << arr[j] << " "; count++;
//in order to print 10 values at each line if (count == 10) { cout << endl; count = 0; } } cout << endl; }
double array_average() { double sum = 0; for (int j = 0; j < arr_length; j++) { sum += arr[j]; } return (sum / arr_length); }
int avg_greater(double avg) { int count = 0; for (int j = 0;j < arr_length; j++) { if (arr[j] > avg) count++; } return count; }
int avg_smaller(double avg) { int count = 0; for (int j = 0;j < arr_length; j++) { if (arr[j] < avg) count++; } return count; }
void neg_to_pos() { for (int j = 0; j < arr_length; j++) { if (arr[j] < 0) { arr[j] = 0 - arr[j]; } } print_array(); }
int no_of_multiple(int k) { int count = 0; for (int j = 0;j < arr_length; j++) { if (arr[j] % k == 0) count++;
} return count; }
int largest() { int max = arr[0], pos = 0; for (int j = 1; j < arr_length; j++) { if (arr[j] > max) pos = j; } return pos; }
int smallest() { int min = arr[0], pos = 0; for (int j = 1; j < arr_length; j++) { if (arr[j] < min) pos = j; } return pos; }
void zero_out() { for (int j = 0; j < arr_length; j++) { if (arr[j] % 2 == 0) arr[j] = 0; } print_array(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
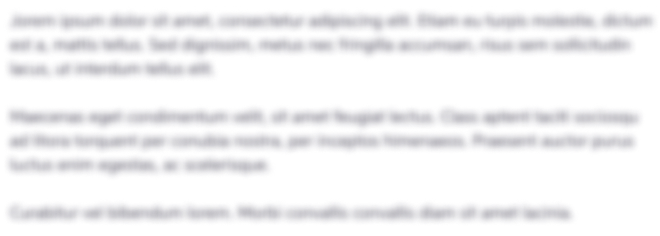
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started