Question
You have a situation where you need to use two stacks. You know that together they never have more than MAX elements. You decide to
You have a situation where you need to use two stacks. You know that together they never have more than MAX elements. You decide to use an array representation with both stacks residing in the same array but with two logical pointers to toptop1 (for stack1) and top2 (for stack2). Stack1 grows from left to right; stack2 grows from right to left. Notice this is not a linked list. Use the following definitions and declarations. Note that the stack is being passed as a parameter to these functions; they are not member functions of a class. You may assume that when the parameter is stack1, the user passes the stack where the top is top1, and that when the parameter is stack2, the user passes the stack where the top is top2. Read the following code segment below and fill in blank #4.
const MAX = 100; typedef int ItemType; // components on the stacks struct StackType { ItemType info[MAX]; int top1; // top for stack1 int top2 // top for stack2 }; void PushS1(StackType& stack1, ItemType item) // Pre: stack1 has been created. // Post: item is on stack1. { ____________; // 1 __________ = item; // 2 } void PushS2(StackType& stack2, ItemType item) // Pre: stack2 has been created. // Post: item is on stack 2. { ___________; // 3 ___________ = item; // 4 }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
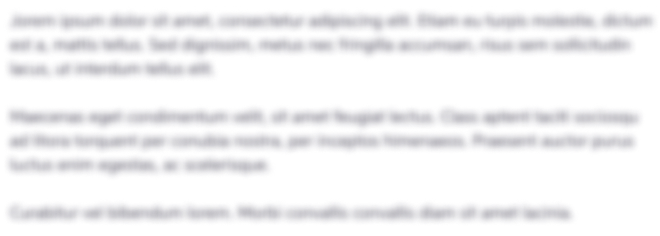
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started