Question
You have been asked to implement a program that determines the letter grade for students at a school. At this school, a students grade is
You have been asked to implement a program that determines the letter grade for students at a school. At this school, a students grade is determined entirely by tests. Because not every student has the same teacher, not every student will have taken the same number of tests. For example, student A may have taken 5 tests while student B has only taken 3 tests.
Your program should first prompt the user for the number of students they wish to enter. For each student, the user will be prompted to enter the students name, how many tests the student has taken, and the grade for each test. Each tests grade will be inputted as a number representing the grade for that test. Once each students information has been entered, the program will display the number of students. Additionally, each student will have their name, list of test scores, and an average of all test scores (shown as a letter grade) displayed.
Your program must have at least the following functions:
int inputData(string*&, double**&);
The inputData function accepts a string pointer and a pointer to a double pointer, both passed by reference. Inside the function, the user will be prompted to enter how many students they will input. For each student, the user will input the students name and a list of the students test scores. The function will return the number of students that have been entered.
After the function call, the string pointer should point to an array of every student name and the pointer to a double pointer should point to an array where each array entry contains an array of that students test scores. Remember that you do not know beforehand how many students will be entered or the number of test scores each student will have.
char* calcGrade(double**, int);
The calcGrade function accepts a pointer to the array of student test scores and the number of students. The function will calculate each students average test score, convert the score to a letter grade, and store the letter grade in a dynamically-allocated array. A standard grading scale will be used:
90 - 100 ? A
80 - 89 ? B
70 - 79 ? C
60 - 69 ? D
< 60 ? F
The function will return a pointer to the dynamically-allocated array containing every students letter grade.
void displayData(string*, double**, char*, int);
The displayData function accepts a pointer to the array of student names, a pointer to the array of student test scores, a pointer to the array of student letter grades, and the number of students. The function should output the following to the console:
The number of students,
and for each student
the students name
a list of the students test scores
the average for all test scores, shown as a letter grade
void cleanHeap(string*, double**, char*, int);
The cleanHeap function accepts a pointer to the array of student names, a pointer to the array of student test scores, a pointer to the array of student letter grades, and the number of students. Inside the function, anything that was dynamically-allocated should be cleaned up appropriately.
Additionally,
You must validate the users input
The number of students and the number of test scores must be a positive value
Each test score must be between 0 and 100, inclusive
You must use pointer arithmetic to access array elements instead of using the subscript operator. For example, to access the fifth element of arr, you must write *(arr + 4) instead of arr[4]
Sample Run:
How many students do you have in the system? 3 Enter the student's name: John Doe Enter how many tests John Doe took: 4 Enter grade # 1: 95.5 Enter grade # 2: 84.3 Enter grade # 3: 80 Enter grade # 4: 88.8 Enter the student's name: Anna Mull Enter how many tests Anna Mull took: 3 Enter grade # 1: 60 Enter grade # 2: 55.9 Enter grade # 3: 77.4 Enter the student's name: Paige Turner Enter how many tests Paige Turner took: -1 Please enter a positive number of tests taken Enter how many tests Paige Turner took: 2 Enter grade # 1: 75.6 Enter grade # 2: -10.3 Please enter a grade between 0 and 100 Enter grade # 2: 50.5 You have 3 students in the system. Name of student #1: John Doe Grades for student #1: 95.5 84.3 80 88.8 Average of grades for student #1:B Name of student #2: Anna Mull Grades for student #2: 60 55.9 77.4 Average of grades for student #2:D Name of student #3: Paige Turner Grades for student #3: 75.6 50.5 Average of grades for student #3:D Process returned 0 (0x0) execution time : 43.441 s Press any key to continue.
That's what I have so far:
#include
using namespace std;
int inputData(string*&, double**&); char* calcGrade(double**, int); void displayData(string*, double**, char*, int); void cleanHeap(string*, double**, char*, int);
int main() { int howManyStudents; string students; int numberOfTests; double grade; cout << "How many students do you have in the system?"; cin >> howManyStudents; cout << endl;
for(int i = 1; i <= howManyStudents; i++) { cout << "Enter the student's name:"; cin.ignore(); getline(cin,students); cout << "How many tests " << students << " took:"; cin >> numberOfTests; if( numberOfTests < 0) cout << "Please enter a positive number of tests taken"<< endl;
for(int j = 1; j <= numberOfTests; j++) { cout << "please enter grade #"<< j << ":"<< endl; cin >> grade; } cout << endl; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
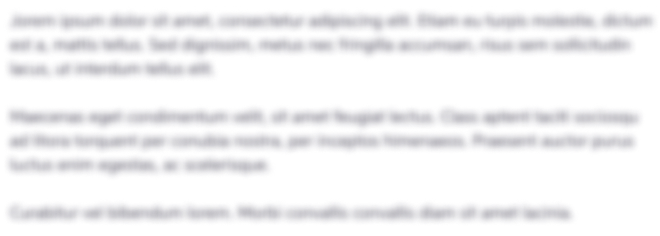
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started