Question
You have been given a class called IntSorter that contains some simple searching and sorting methods: linearSearch, slectionSort, and binarySearch. Your assignment is to prepare
You have been given a class called IntSorter that contains some simple searching and sorting methods: linearSearch, slectionSort, and binarySearch. Your assignment is to prepare a test plan for each of the methods and write a class IntSorterTester that implements this test plan.
- Under the src directory of your project csc205, create a package called: testing_complexity
- Under the package testing_complexity, create a new class named IntSortSearch. Copy and paste from the attached file IntSortSearch.java
- Now write the IntSortSearchTester class. It will contain the following methods:
- public static void linearSearchTester() This hard-codes the test data for linearSearch method
- public static void binarySearchTester() This hard-codes the test data for binarySearch method
- public static void sortTester() This hard-codes the test data for selectionSort method
- public static void main(String[] args) method which just calls all the tester methods, using statements like this: linearSearchTester();
How to create the test data for hardcoding into the tester methods:
- In each of the two search tester methods, you should have test cases (written in the style of the example given in lecture, see further details in the submission instructions below) that simulate the following situations:
- Array containing one element only, and the value being searched for is the element in the array
- Array containing one element only, and the value being searched for is NOT the element in the array
- Array containing two unequal elements, and the value being searched for is one of the elements of the array
- Array containing two unequal elements, and the value being searched for is NOT ANY of the elements of the array
- Array containing 3 elements, and the value being searched for is the very first element of the array
- Array containing 3 elements, and the value being searched for is the very last element of the array
- Array containing 3 elements, and the value being searched for is the middle element
- Array containing 3 elements, and the value being searched for is NOT ANY of the elements in the array
- For the one sort tester method, you should have test cases (again, written in the style of the example given in lecture) that simulate the following situations:
-
- Array containing one element only
- Array containing two unequal elements
- Array containing 3 elements, already sorted
- Array containing 3 elements, already sorted in the reverse order
- Array containing 3 elements, all of them negative
- Array containing 3 elements, one negative, one zero, one positive
- Array containing 3 elements, all of them the same value (e.g.: 5,5,5)
- Run IntSortSearchTester and turn in the code and the output that shows the results of whether your tests passed or failed.
NOTE:
You must write the tester code for each test case above in the style discussed. That is, you must clearly have the test data shown, the expected output and the actual output must be captured by calling the appropriate search or sort method (static method so it can be invoked using just the class name).
Then, for each test case, you must compare the actual result with the expected result (this could require a loop if the actual and expected results are arrays). The result of the comparison should simply be printed as true or false depending on whether the test passed or failed. Therefore, I will expect to see a series of true values printed, one on each line, and thus be assured that all tests passed. Note that you may need to remove some printout statements to only get a list of test cases and true/false for each test case.
Here is a sample of what your output should look like:
Testing linearSearch:
Test 4.a: true
Test 4.b: true
Test 4.c: true
Test 4.d: true
Test 4.e: true
Test 4.f: true
Test 4.g: true
Test 4.h: true
Testing binarySearch:
Test 4.a: true
Test 4.b: true
Test 4.c: false
package testing_complexity; public class IntSortSearch { private static int numComps = 0; //-------------------------------------------------- public static int getNumComps() { return numComps; } //-------------------------------------------------- public static int linearSearch(int[] a, int x) { numComps = 0; for (int k = 0; k < a.length; k++) { //------Line 1 numComps++; if (a[k] == x) { //-----------------------Line 2 System.out.println("Num Comps = " + numComps); return k;//-------------------------Line 3 } } System.out.println("Num Comps = " + numComps); return -1;//------------------------------Line 4 } //-------------------------------------------------- private static int select(int[] a, int k) { //finds the location of the smallest element in the array //between position k and the end of the array int smallestPos = k; //-------------------Line 5 for (int j = k; j < a.length; j++) { numComps++; if (a[j] < a[smallestPos])//----------Line 6 smallestPos = j;//-----------------Line 7 } return smallestPos;//--------------------Line 8 } //-------------------------------------------------- public static void selectionSort(int[] a){ int pos; //position of the smallest element int temp; //temp variable for swap numComps = 0; for (int k = 0; k < a.length-1; k++) {//---Line 9 //find the smallest element pos = select(a, k);//-----------------Line 10 //swap it with k-th element temp = a[k]; a[k] = a[pos]; a[pos] = temp; } System.out.println("Num Comps = " + numComps);//Line 11 } //-------------------------------------------------- public static int binarySearch(int[] a, int x) { int lowerLimit = 0; int upperLimit = a.length-1; int middle = 0; numComps = 0; while (lowerLimit <= upperLimit) {//-------------Line 12 numComps++; middle = (lowerLimit + upperLimit) / 2; if (a[middle] == x){//---------------------Line 13 System.out.println("Num Comps = " + numComps); return middle;//------------------------Line 14 } if (a[middle] < x){//----------------------Line 15 lowerLimit = middle + 1;//----------------Line 16 } else { upperLimit = middle - 1;//----------------Line 17 } } System.out.println("Num Comps = " + numComps); return -1;//-----------------------------------Line 18 } //-------------------------------------------------- }
SUBMISSION:
Copy and paste all the code and output into this file
Step by Step Solution
There are 3 Steps involved in it
Step: 1
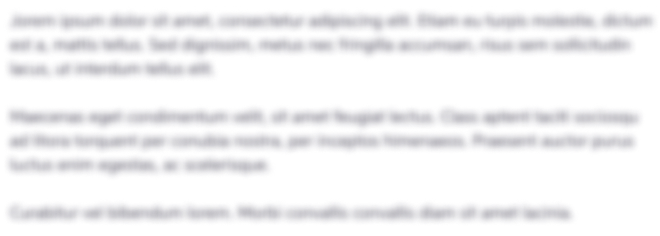
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started