Question
You have been given a partially implemented binary search tree class and a binary search tree node class to use. Your task is to implement
You have been given a partially implemented binary search tree class and a binary search tree node class to use. Your task is to implement the following methods in the binary search tree class BinTree according to the given specification:
boolean isEmpty() This function should determine whether the binary search tree is empty or not. It returns true if the binary search tree is empty and false otherwise.
BinTreeNode
Note: Make sure you dont make any changes to the original tree.
BinTreeNode
Note: Make sure you dont make any changes to the original tree. void printPreorder() This function should print out all the elements in the binary search tree as a space separated list that is terminated by a newline. This function should use the pre-order tree traversal strategy.
void printPostorder() This function should print out all the elements in the binary search tree as a space separated list that is terminated by a newline. This function should use the post-order tree traversal strategy.
void insert(T elem) This function should insert the given elem in the binary search tree. elem should be inserted in the correct position in the binary search tree to maintain the sorted order required by a binary search tree.
boolean deleteByMerge(T elem) This function should delete the given elem from the binary search tree. It returns true if elem is removed successfully and false otherwise. This function should use the delete by merging strategy.
boolean deleteByCopy(T elem) This function should delete the given elem from the binary search tree. It returns true if elem is removed successfully and false otherwise. This function should use the delete by copying strategy.
T search(T elem) This function should find the given elem in the binary search tree. If elem is found in the binary search tree it should return the element and otherwise it should return null.
Only implement the methods listed above. You can use both iterative and recursive approaches. You may use your own helper functions to assist in implementing the specification. However, you may not modify any of the given method signatures. Also do not modify any of the other code.
Given Code:
////////BinTreeNode.java//////////
public class BinTreeNode
////////BinTree.java//////////
@SuppressWarnings("unchecked") public class BinTree
////// You may not change any code above this line //////
////// Implement the functions below this line //////
Step by Step Solution
There are 3 Steps involved in it
Step: 1
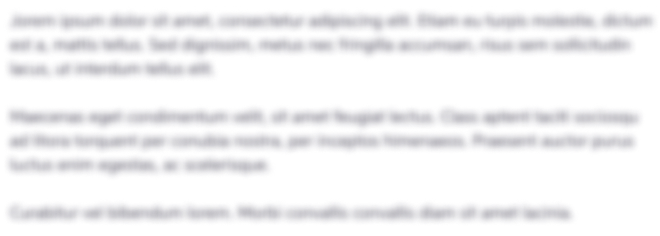
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started