Question
You need to use the node structure provided below struct node { string name; double resistance; double voltage_across; double power_across; }; Implement a series network
You need to use the node structure provided below
struct node {
string name;
double resistance;
double voltage_across;
double power_across;
};
Implement a series network that has unlimited number of resistors stored in a C++ vector. In general, to solve this problem you will do the following:
-Prompt for the input voltage
-Group input resistor values and store them in a vector.
-Solve for series current
-Calculate total circuit power
-Calculate the voltage drop across each resistor
-Calculate the power dissipated by each resistor.
-Display Network
You need to create at least five functions
Some/most of the above bullet points could/should become individual functions to solve this problem.
Please test your functions with a variety of inputs in main().
To determine the series current you will first need to add up all the series resistors (Rtotal). Once you have Rtotal the current simply becomes:
Series Current = Voltage Source / Rtotal
You can use this Current to determine the power dissipation and voltage drops across the individual resistors.
Can modify code below or start from scratch using C++
#include#include #include using namespace std; // You must use this structure struct node { string name; double resistance; double voltage_across; double power_across; }; // An Example of the type of function you may want to create string nodeName() { string node_name; cout << "Enter Node Name: "; cin >> node_name; return node_name; } // // The comments indicate good places to covvert code to short functions // int main() { node N1; // Enter source voltage double source_voltage; cout << "Enter Source Voltage: "; cin >> source_voltage; // Enter basic Node Information N1.name = nodeName(); cout << "Resistor Value: "; cin >> N1.resistance; // Calculate series current for the one-node network double total_resistance = N1.resistance; double series_current = source_voltage/ total_resistance; // Calculate voltage across the restistor N1.voltage_across = series_current * N1.resistance; // Calculate the power across the resistor N1.power_across = N1.voltage_across * series_current; // Display Network Information cout << "Series Network: " << endl; cout << "Source Voltage: " << source_voltage << "-Volts" << endl; cout << "Total Resistance: " << total_resistance << "-Ohms" << endl; cout << "Series Current: " << series_current << "-Amperes" << endl; // Display Node information cout << "Node Name: " << N1.name << endl; cout << "Node Voltage: " << N1.voltage_across << "-Volts" << endl; cout << "Node Resistance: " << N1.resistance << "-Ohms" << endl; cout << "Node Power: " << N1.power_across << "-Watts" << endl; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
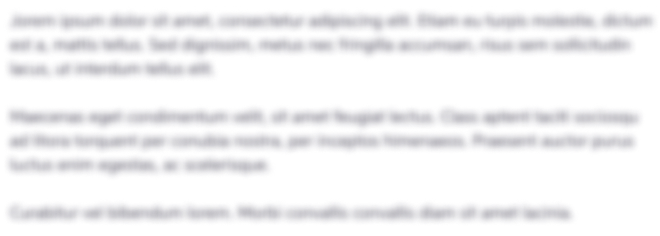
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started