Question
You will be writing the code the create a doubly linked list of vehicles. (1) Complete the VehicleNode class per the following specifications: Private class
You will be writing the code the create a doubly linked list of vehicles.
(1) Complete the VehicleNode class per the following specifications:
- Private class attributes
- String type
- int seats
- String color
- class reference for a next node
- class reference for a previous node
- Public methods:
- Constructors: empty and non-empty parameter list
- setNext()
- setPrevious()
- getNext()
- getPrevious()
(2) Complete the four TODO's in the BudgetRental class
- insertAtFront(String, int, String) - (2 pts)
- insertAtEnd(String, int, String) - (2 pts)
- printForward()
- printBackward()
(3) In the Main class, complete the two TODO sections to call the correct method and pass the arguments.
When the input is:
6 SUV,5,Black Sport,2,Red Sedan,4,Beige Truck,3,Blue Van,10,White SUV,6,Green
The output should be:
Type # of seats color ----- ---------- ----- SUV 6 Green Truck 3 Blue Sport 2 Red SUV 5 Black Sedan 4 Beige Van 10 White Data reversed Van 10 White Sedan 4 Beige SUV 5 Black Sport 2 Red Truck 3 Blue SUV 6 Green
import java.util.Scanner;
public class Main {
public static void main(String[] args) { Scanner scnr = new Scanner(System.in); BudgetRental budg = new BudgetRental(); int input = scnr.nextInt(); scnr.nextLine(); //clears the buffer for (int i = 0; i < input; i++) { String line = scnr.nextLine(); String[] tokens = line.split(","); if ((i % 2) == 0) { // TODO call the insertAtEnd method passing the arguments } else { // TODO call the insertAtFront method passing the arguments } } System.out.println(); System.out.printf("%-5s %12s %7s ","Type","# of seats","color"); System.out.printf("%-5s %12s %7s ","-----","----------", "-----"); budg.printForward(); System.out.println(); System.out.println("\tData reversed"); budg.printBackward(); }
}
public class BudgetRental {
private VehicleNode headNode; private VehicleNode tailNode;
public BudgetRental() { // Front and end of node list, the head and tail nodes don't store any data // and are known as dummy nodes headNode = new VehicleNode(); tailNode = new VehicleNode(); }
// TODO: Define the insertAtFront() method with the three needed parameters // that inserts a node at the front of the doubly linked list (after the dummy head node)
// TODO: Define the insertAtEnd() method with the three needed parameters // that inserts a node to the end of the doubly linked list (before the dummy tail node)
// TODO: Define the printInfo method to starts at the front of the list // and iterates through the list but excludes the dummy nodes
// TODO: Complete the printBackward method to starts at the tail of the list // and iterates through the list but excludes the dummy nodes
// The following two method are for the unit tests of the lab only public VehicleNode getHeadNext(){ return this.headNode.getNext(); } public VehicleNode getTailPrevious(){ return this.tailNode.getPrevious(); } }
public class VehicleNode {
public void printNodeData() { System.out.printf("%-5s %7d %12s ", type, seats, color); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
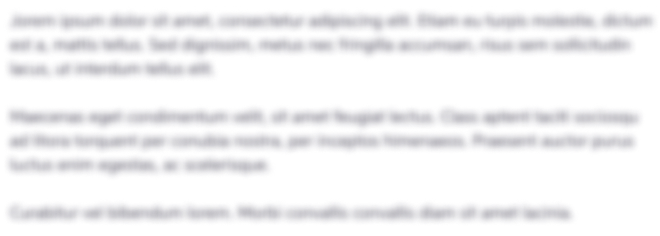
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started