Question
You will need to develop three programs: physical_wire.c, data_link_layer.c, and network_layer.c. You are given a header file (structs.h) that defines the frame and packet structures.
You will need to develop three programs: physical_wire.c, data_link_layer.c, and network_layer.c. You are given a header file (structs.h) that defines the frame and packet structures. Each host should be given a nickname such as host1 is Bob and host2 is Adam. Following are the algorithms for the three programs. The window outputs should look like the following images:
The code that I have already tried and is correct for the most part can be found here in the answers section: https://www.chegg.com/homework-help/questions-and-answerseed-develop-three-programs-physicalwirec-datalinklayerc-networklayerc-given-header-file-s-q25107132
physical_wire.c
{
Create a socket, bind its address to the socket, and listen to it.
Accept two connection requests. Generate a thread for each connection. (The thread will reads frames from a socket and send them to the other socket. If the frame contains "EXIT" then the socket is closed and the thread is terminated.)
The main function terminates after the two threads terminate.
}
data_link_layer.c
{
Create a socket and use it to connect to the physical wire.
Create a socket, bind its address to it, and listen to it.
Accept one connection request from the network layer.
Create a thread (child thread) to read frames from the wire, remove the header, and send the included packets to the network layer.
The main function will read packets from the network layer, wrap them in frames, and send them to the wire.
If the message in the packet is "EXIT", then
Kill the child thread
Close the socket to which the network layer is connected
Close the socket through which the physical wire is connected.Terminate the main function.
}
network_layer.c
{
Create a socket and use it to connect to the data link layer.
Create a thread (child thread) to receive packets from the data link layer.
The main will receive messages from keyboard, wrap them into packets and send them to the data link layer.
If the message is "EXIT", then
Kill the child thread
Close the socket
Terminate the main function.
}
Usage
physical_wire.exe wire_port
wire_port is the port the physical wire will listen to. It will accept two connection requests from data link layers through this port.
data_link_layer.exe wire_addr wire_port data_port
wire_addr is the IP address (or name) of the machine on which physical_wire.exe is running,
wire_port is the port number that physical_wire listens to,
data_prot is the port number that data link layer will listen to. It will accept one connection request from network layer through this port.
network_layer.exe data_addr data_port nickname
data_addr is the IP address of the machine on which data link layer (of this host) is running.
data_port is the port number that the data link layer (of this host) listens to.
Nickname is the name we assign to this host.
===========structs.h======================
typedef struct packet_type { char nickname[10]; char message[256]; }packet; typedef struct frame_type { int seq_num; int type; // 1 for acknowledgement; 0 for data packet my_packet; }frame;| ip chayan@labol;-/459/Project2 chayanlabl: /459/Project2s . etwork layer.exe lab01.cs ndsu.nodak.edu 2500 Bob Ready to communicate chayan@lab00:-/459/Project2 G EZ hayanlab00:/459/Project2s .etworklayer.exe lab00.cs.ndsu nodak.edu 3000 Adam Ready to communicate chayan@labl 0:-/459/Project2 chayan@1ab00:-/459/Project25 su.nodak.edu 4000 3000 create new socket 5 chayan@lab01: 459/Project . /data link 1ayer.exe 1ab0 2.cs.nd chayanlab01: /459/Project2s -/data link layer.exe lab02.c s.ndsu. nodak.edu 4000 2500 create new socket: 5 - cP chayan@lab02:-/459/Project2 chayanglab02: /459/eroject2s ./physical wire.exe 4000 create new socket: 4 create new socket: 5 | ip chayan@labol;-/459/Project2 chayanlabl: /459/Project2s . etwork layer.exe lab01.cs ndsu.nodak.edu 2500 Bob Ready to communicate chayan@lab00:-/459/Project2 G EZ hayanlab00:/459/Project2s .etworklayer.exe lab00.cs.ndsu nodak.edu 3000 Adam Ready to communicate chayan@labl 0:-/459/Project2 chayan@1ab00:-/459/Project25 su.nodak.edu 4000 3000 create new socket 5 chayan@lab01: 459/Project . /data link 1ayer.exe 1ab0 2.cs.nd chayanlab01: /459/Project2s -/data link layer.exe lab02.c s.ndsu. nodak.edu 4000 2500 create new socket: 5 - cP chayan@lab02:-/459/Project2 chayanglab02: /459/eroject2s ./physical wire.exe 4000 create new socket: 4 create new socket: 5
Step by Step Solution
There are 3 Steps involved in it
Step: 1
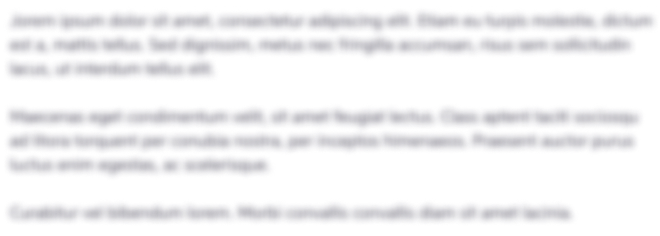
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started